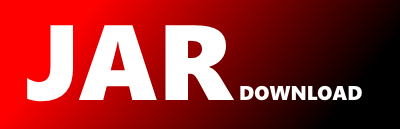
com.ly.mybatis.mapperservice.table.MyBatisTableUtil Maven / Gradle / Ivy
package com.ly.mybatis.mapperservice.table;
import com.baomidou.mybatisplus.core.toolkit.support.SFunction;
import com.ly.mybatis.mapperservice.table.lambda.Lambda;
import com.ly.mybatis.mapperservice.table.lambda.LambdaUtil;
import java.util.Arrays;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
@SuppressWarnings("unused")
public class MyBatisTableUtil {
private static final MyBatisTableHolder myBatisTableHolder = new MyBatisTableHolder();
public static String getColumnBySFunction(SFunction function) {
Class tClass = LambdaUtil.getClass(function);
Map map = getTableFieldMap(tClass, function);
Map.Entry entry = map.entrySet().parallelStream()
.findAny().orElse(null);
if (entry == null) {
return null;
}
return entry.getValue();
}
@SafeVarargs
public static Map getTableFieldMap(Class tClass, SFunction... functions) {
TableInfo tableInfo = getTableInfo(tClass);
Map map = tableInfo.getFieldList().parallelStream()
.collect(Collectors.toMap(
TableFieldInfo::getProperty,
TableFieldInfo::getColumn,
(a, b) -> a
));
if (functions.length != 0) {
return Arrays.stream(functions).parallel()
.map(LambdaUtil::getFieldName)
.filter(map::containsKey)
.collect(Collectors.toMap(Function.identity(), map::get, (a, b) -> a));
} else {
return map;
}
}
public static TableInfo getTableInfo(Class> clazz) {
return myBatisTableHolder.get(clazz);
}
public static TableFieldInfo getTableField(SFunction function) {
Lambda lambda = LambdaUtil.getLambda(function);
TableInfo tableInfo = getTableInfo(lambda.getImplClass());
return tableInfo.getFieldList().parallelStream()
.filter(tableFieldInfo -> tableFieldInfo.getProperty().equals(lambda.getImplField().getName()))
.findFirst()
.orElse(null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy