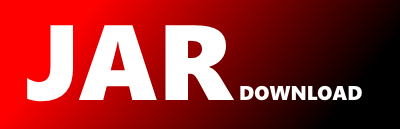
org.remapper.dto.RootNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of remapper Show documentation
Show all versions of remapper Show documentation
An automated code entity matching algorithm
The newest version!
package org.remapper.dto;
import org.eclipse.jdt.core.dom.ASTNode;
import org.eclipse.jdt.core.dom.CompilationUnit;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class RootNode extends DeclarationNodeTree {
private List allNodes;
public RootNode(CompilationUnit cu, String filePath, ASTNode node) {
super(cu, filePath, node);
super.setHeight(0);
super.setType(EntityType.COMPILATION_UNIT);
super.setNamespace("");
super.setName("root");
super.setRoot(true);
super.setLeaf(false);
}
/**
* All nodes after pruning
*/
public List getAllNodes() {
if (allNodes == null) {
allNodes = new ArrayList<>();
depthFirstSearch(allNodes, getChildren());
}
return allNodes;
}
public List getLeafNodes() {
return getAllNodes().stream().filter(node -> node.isLeaf() && !node.isMatched()).
map(node -> (LeafNode) node).collect(Collectors.toList());
}
public List getInternalNodes() {
return getAllNodes().stream().filter(node -> !node.isLeaf() && !node.isRoot() && !node.isMatched()).
map(node -> (InternalNode) node).collect(Collectors.toList());
}
public List getUnmatchedNodes() {
return getAllNodes().stream().filter(node -> !node.isMatched()).collect(Collectors.toList());
}
private void depthFirstSearch(List list, List children) {
for (DeclarationNodeTree child : children) {
depthFirstSearch(list, child.getChildren());
list.add(child);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy