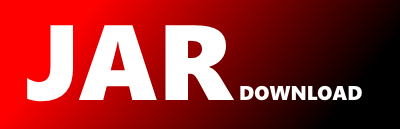
commonMain.io.github.lyxnx.compose.pine.InputFieldDefaults.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pine Show documentation
Show all versions of pine Show documentation
Jetpack Compose Pine Theme
package io.github.lyxnx.compose.pine
import androidx.compose.foundation.layout.PaddingValues
import androidx.compose.foundation.text.selection.TextSelectionColors
import androidx.compose.runtime.Composable
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.unit.dp
import io.github.lyxnx.compose.ui.AnimatedInteractionStateColorSelector
/**
* Contains the default values used by an [InputField]
*/
public object InputFieldDefaults {
/**
* Default input field content padding
*/
public val ContentPadding: PaddingValues = PaddingValues(16.dp)
/**
* Default style of the main input field text
*/
public val TextStyle: TextStyle
@Composable
get() = PineTheme.typography.bodyMedium
/**
* Default style of the input field placeholder
*/
public val PlaceholderStyle: TextStyle
@Composable
get() = PineTheme.typography.bodyMedium
/**
* Creates an [InputFieldColors] instance that represents the colors in different states
*/
@Composable
public fun inputFieldColors(
defaultBorderColor: Color = PineTheme.colors.grey300,
pressedBorderColor: Color = PineTheme.colors.grey200,
focusedBorderColor: Color = PineTheme.colors.primary500,
disabledBorderColor: Color = PineTheme.colors.grey300,
defaultBackgroundColor: Color = PineTheme.colors.background,
pressedBackgroundColor: Color = PineTheme.colors.grey100,
focusedBackgroundColor: Color = PineTheme.colors.background,
disabledBackgroundColor: Color = PineTheme.colors.grey300,
defaultContentColor: Color = PineTheme.colors.grey900,
pressedContentColor: Color = PineTheme.colors.grey900,
focusedContentColor: Color = PineTheme.colors.grey900,
disabledContentColor: Color = PineTheme.colors.grey400,
defaultPlaceholderContentColor: Color = PineTheme.colors.grey500,
pressedPlaceholderContentColor: Color = PineTheme.colors.grey500,
focusedPlaceholderContentColor: Color = PineTheme.colors.grey500,
disabledPlaceholderContentColor: Color = PineTheme.colors.grey400,
defaultPrefixContentColor: Color = PineTheme.colors.grey900,
pressedPrefixContentColor: Color = PineTheme.colors.grey900,
focusedPrefixContentColor: Color = PineTheme.colors.grey900,
disabledPrefixContentColor: Color = PineTheme.colors.grey900,
defaultLeadingIconColor: Color = PineTheme.colors.grey500,
pressedLeadingIconColor: Color = PineTheme.colors.grey500,
focusedLeadingIconColor: Color = PineTheme.colors.grey500,
disabledLeadingIconColor: Color = PineTheme.colors.grey400,
defaultTrailingIconColor: Color = PineTheme.colors.grey500,
pressedTrailingIconColor: Color = PineTheme.colors.grey500,
focusedTrailingIconColor: Color = PineTheme.colors.grey500,
disabledTrailingIconColor: Color = PineTheme.colors.grey400,
defaultSuffixContentColor: Color = PineTheme.colors.grey900,
pressedSuffixContentColor: Color = PineTheme.colors.grey900,
focusedSuffixContentColor: Color = PineTheme.colors.grey900,
disabledSuffixContentColor: Color = PineTheme.colors.grey900,
selectionColors: TextSelectionColors = TextSelectionColors(
handleColor = PineTheme.colors.primary500,
backgroundColor = PineTheme.colors.primary100
),
focusedShadowColor: Color = PineTheme.colors.primary600.copy(alpha = 0.25f)
): InputFieldColors = InputFieldColors(
borderColor = AnimatedInteractionStateColorSelector(
default = defaultBorderColor,
pressed = pressedBorderColor,
focused = focusedBorderColor,
disabled = disabledBorderColor
),
backgroundColor = AnimatedInteractionStateColorSelector(
default = defaultBackgroundColor,
pressed = pressedBackgroundColor,
focused = focusedBackgroundColor,
disabled = disabledBackgroundColor
),
contentColor = AnimatedInteractionStateColorSelector(
default = defaultContentColor,
pressed = pressedContentColor,
focused = focusedContentColor,
disabled = disabledContentColor
),
placeholderContentColor = AnimatedInteractionStateColorSelector(
default = defaultPlaceholderContentColor,
pressed = pressedPlaceholderContentColor,
focused = focusedPlaceholderContentColor,
disabled = disabledPlaceholderContentColor
),
prefixContentColor = AnimatedInteractionStateColorSelector(
default = defaultPrefixContentColor,
pressed = pressedPrefixContentColor,
focused = focusedPrefixContentColor,
disabled = disabledPrefixContentColor
),
leadingIconColor = AnimatedInteractionStateColorSelector(
default = defaultLeadingIconColor,
pressed = pressedLeadingIconColor,
focused = focusedLeadingIconColor,
disabled = disabledLeadingIconColor
),
trailingIconColor = AnimatedInteractionStateColorSelector(
default = defaultTrailingIconColor,
pressed = pressedTrailingIconColor,
focused = focusedTrailingIconColor,
disabled = disabledTrailingIconColor
),
suffixContentColor = AnimatedInteractionStateColorSelector(
default = defaultSuffixContentColor,
pressed = pressedSuffixContentColor,
focused = focusedSuffixContentColor,
disabled = disabledSuffixContentColor
),
selectionColors = selectionColors,
focusedShadowColor = focusedShadowColor
)
/**
* Creates an [InputFieldColors] instance that represents the colors in an error state
*/
@Composable
public fun errorInputFieldColors(
defaultBorderColor: Color = PineTheme.colors.error300,
pressedBorderColor: Color = PineTheme.colors.error200,
focusedBorderColor: Color = PineTheme.colors.error500,
disabledBorderColor: Color = PineTheme.colors.grey300,
defaultBackgroundColor: Color = PineTheme.colors.background,
pressedBackgroundColor: Color = PineTheme.colors.grey100,
focusedBackgroundColor: Color = PineTheme.colors.background,
disabledBackgroundColor: Color = PineTheme.colors.grey300,
defaultContentColor: Color = PineTheme.colors.error500,
pressedContentColor: Color = PineTheme.colors.error500,
focusedContentColor: Color = PineTheme.colors.error500,
disabledContentColor: Color = PineTheme.colors.error400,
defaultPlaceholderContentColor: Color = PineTheme.colors.error300,
pressedPlaceholderContentColor: Color = PineTheme.colors.error300,
focusedPlaceholderContentColor: Color = PineTheme.colors.error300,
disabledPlaceholderContentColor: Color = PineTheme.colors.grey400,
defaultPrefixContentColor: Color = PineTheme.colors.error900,
pressedPrefixContentColor: Color = PineTheme.colors.error900,
focusedPrefixContentColor: Color = PineTheme.colors.error900,
disabledPrefixContentColor: Color = PineTheme.colors.grey900,
defaultLeadingIconColor: Color = PineTheme.colors.error500,
pressedLeadingIconColor: Color = PineTheme.colors.error500,
focusedLeadingIconColor: Color = PineTheme.colors.error500,
disabledLeadingIconColor: Color = PineTheme.colors.grey400,
defaultTrailingIconColor: Color = PineTheme.colors.error500,
pressedTrailingIconColor: Color = PineTheme.colors.error500,
focusedTrailingIconColor: Color = PineTheme.colors.error500,
disabledTrailingIconColor: Color = PineTheme.colors.grey400,
defaultSuffixContentColor: Color = PineTheme.colors.error500,
pressedSuffixContentColor: Color = PineTheme.colors.error500,
focusedSuffixContentColor: Color = PineTheme.colors.error500,
disabledSuffixContentColor: Color = PineTheme.colors.grey900,
selectionColors: TextSelectionColors = TextSelectionColors(
handleColor = PineTheme.colors.primary500,
backgroundColor = PineTheme.colors.primary100
),
focusedShadowColor: Color = PineTheme.colors.error600.copy(alpha = 0.25f)
): InputFieldColors = inputFieldColors(
defaultBorderColor = defaultBorderColor,
pressedBorderColor = pressedBorderColor,
focusedBorderColor = focusedBorderColor,
disabledBorderColor = disabledBorderColor,
defaultBackgroundColor = defaultBackgroundColor,
pressedBackgroundColor = pressedBackgroundColor,
focusedBackgroundColor = focusedBackgroundColor,
disabledBackgroundColor = disabledBackgroundColor,
defaultContentColor = defaultContentColor,
pressedContentColor = pressedContentColor,
focusedContentColor = focusedContentColor,
disabledContentColor = disabledContentColor,
defaultPlaceholderContentColor = defaultPlaceholderContentColor,
pressedPlaceholderContentColor = pressedPlaceholderContentColor,
focusedPlaceholderContentColor = focusedPlaceholderContentColor,
disabledPlaceholderContentColor = disabledPlaceholderContentColor,
defaultPrefixContentColor = defaultPrefixContentColor,
pressedPrefixContentColor = pressedPrefixContentColor,
focusedPrefixContentColor = focusedPrefixContentColor,
disabledPrefixContentColor = disabledPrefixContentColor,
defaultLeadingIconColor = defaultLeadingIconColor,
pressedLeadingIconColor = pressedLeadingIconColor,
focusedLeadingIconColor = focusedLeadingIconColor,
disabledLeadingIconColor = disabledLeadingIconColor,
defaultTrailingIconColor = defaultTrailingIconColor,
pressedTrailingIconColor = pressedTrailingIconColor,
focusedTrailingIconColor = focusedTrailingIconColor,
disabledTrailingIconColor = disabledTrailingIconColor,
defaultSuffixContentColor = defaultSuffixContentColor,
pressedSuffixContentColor = pressedSuffixContentColor,
focusedSuffixContentColor = focusedSuffixContentColor,
disabledSuffixContentColor = disabledSuffixContentColor,
selectionColors = selectionColors,
focusedShadowColor = focusedShadowColor
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy