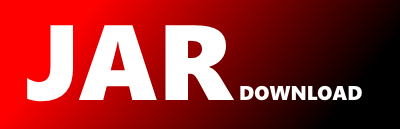
commonMain.io.github.lyxnx.compose.pine.ButtonDefaults.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pine Show documentation
Show all versions of pine Show documentation
Jetpack Compose Pine Theme
package io.github.lyxnx.compose.pine
import androidx.compose.foundation.layout.PaddingValues
import androidx.compose.foundation.shape.CornerBasedShape
import androidx.compose.runtime.Composable
import androidx.compose.runtime.ReadOnlyComposable
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.DpSize
import androidx.compose.ui.unit.dp
import io.github.lyxnx.compose.ui.AnimatedInteractionStateColorSelector
/**
* Contains the default values used by a [Button]
*/
public object ButtonDefaults {
/**
* The default shape of a [Button]. Uses [PineShapes.small]
*/
public val Shape: CornerBasedShape
@ReadOnlyComposable
@Composable
get() = PineTheme.shapes.small
/**
* The default padding of button content between the content and its border
*/
public val Padding: PaddingValues = PaddingValues(horizontal = 15.dp, vertical = 8.dp)
/**
* The default padding of a [TextButton]
*/
public val TextButtonPadding: PaddingValues = PaddingValues(horizontal = 12.dp, vertical = 12.dp)
/**
* The default padding of a button that contains only an icon
*/
public val IconPadding: PaddingValues = PaddingValues(10.dp)
/**
* The default size of a button icon
*/
public val IconSize: DpSize = DpSize(24.dp, 24.dp)
/**
* Creates a [ButtonColors] instance that represents the colors of a primary button in different states
*/
@Composable
public fun buttonColors(
borderColor: Color = PineTheme.colors.primary500,
pressedBorderColor: Color = PineTheme.colors.primary600,
focusedBorderColor: Color = PineTheme.colors.primary400,
disabledBorderColor: Color = PineTheme.colors.grey300,
backgroundColor: Color = PineTheme.colors.primary500,
pressedBackgroundColor: Color = PineTheme.colors.primary600,
focusedBackgroundColor: Color = PineTheme.colors.primary400,
disabledBackgroundColor: Color = PineTheme.colors.grey300,
contentColor: Color = PineTheme.colors.white,
pressedContentColor: Color = PineTheme.colors.white,
focusedContentColor: Color = PineTheme.colors.white,
disabledContentColor: Color = PineTheme.colors.grey400,
focusedShadowColor: Color = PineTheme.colors.primary600.copy(alpha = 0.5f),
loadingTrackColor: Color = PineTheme.colors.primary400,
loadingBarColor: Color = PineTheme.colors.white
): ButtonColors = ButtonColors(
borderColor = AnimatedInteractionStateColorSelector(
default = borderColor,
pressed = pressedBorderColor,
focused = focusedBorderColor,
disabled = disabledBorderColor
),
backgroundColor = AnimatedInteractionStateColorSelector(
default = backgroundColor,
pressed = pressedBackgroundColor,
focused = focusedBackgroundColor,
disabled = disabledBackgroundColor
),
contentColor = AnimatedInteractionStateColorSelector(
default = contentColor,
pressed = pressedContentColor,
focused = focusedContentColor,
disabled = disabledContentColor
),
focusedShadowColor = focusedShadowColor,
loadingTrackColor = loadingTrackColor,
loadingBarColor = loadingBarColor
)
/**
* Creates a [ButtonColors] instance that represents the colors of a secondary button in different states
*/
@Composable
public fun secondaryButtonColors(
borderColor: Color = PineTheme.colors.primary200,
pressedBorderColor: Color = PineTheme.colors.primary700,
focusedBorderColor: Color = PineTheme.colors.primary400,
disabledBorderColor: Color = PineTheme.colors.grey300,
backgroundColor: Color = PineTheme.colors.primary100,
pressedBackgroundColor: Color = PineTheme.colors.primary100,
focusedBackgroundColor: Color = PineTheme.colors.primary100,
disabledBackgroundColor: Color = PineTheme.colors.grey300,
contentColor: Color = PineTheme.colors.primary600,
pressedContentColor: Color = PineTheme.colors.primary700,
focusedContentColor: Color = PineTheme.colors.primary400,
disabledContentColor: Color = PineTheme.colors.grey400,
focusedShadowColor: Color = PineTheme.colors.primary600.copy(alpha = 0.5f),
loadingTrackColor: Color = PineTheme.colors.primary200,
loadingBarColor: Color = PineTheme.colors.primary600
): ButtonColors = ButtonColors(
borderColor = AnimatedInteractionStateColorSelector(
default = borderColor,
pressed = pressedBorderColor,
focused = focusedBorderColor,
disabled = disabledBorderColor
),
backgroundColor = AnimatedInteractionStateColorSelector(
default = backgroundColor,
pressed = pressedBackgroundColor,
focused = focusedBackgroundColor,
disabled = disabledBackgroundColor
),
contentColor = AnimatedInteractionStateColorSelector(
default = contentColor,
pressed = pressedContentColor,
focused = focusedContentColor,
disabled = disabledContentColor
),
focusedShadowColor = focusedShadowColor,
loadingTrackColor = loadingTrackColor,
loadingBarColor = loadingBarColor
)
/**
* Creates a [ButtonColors] instance that represents the colors of a tertiary button in different states
*/
@Composable
public fun tertiaryButtonColors(
borderColor: Color = PineTheme.colors.grey200,
pressedBorderColor: Color = PineTheme.colors.grey700,
focusedBorderColor: Color = PineTheme.colors.grey500,
disabledBorderColor: Color = PineTheme.colors.grey300,
backgroundColor: Color = PineTheme.colors.background,
pressedBackgroundColor: Color = PineTheme.colors.grey100,
focusedBackgroundColor: Color = PineTheme.colors.grey100,
disabledBackgroundColor: Color = PineTheme.colors.grey300,
contentColor: Color = PineTheme.colors.grey600,
pressedContentColor: Color = PineTheme.colors.grey700,
focusedContentColor: Color = PineTheme.colors.grey500,
disabledContentColor: Color = PineTheme.colors.grey400,
focusedShadowColor: Color = PineTheme.colors.grey600.copy(alpha = 0.5f),
loadingTrackColor: Color = PineTheme.colors.grey200,
loadingBarColor: Color = PineTheme.colors.grey600
): ButtonColors = ButtonColors(
borderColor = AnimatedInteractionStateColorSelector(
default = borderColor,
pressed = pressedBorderColor,
focused = focusedBorderColor,
disabled = disabledBorderColor
),
backgroundColor = AnimatedInteractionStateColorSelector(
default = backgroundColor,
pressed = pressedBackgroundColor,
focused = focusedBackgroundColor,
disabled = disabledBackgroundColor
),
contentColor = AnimatedInteractionStateColorSelector(
default = contentColor,
pressed = pressedContentColor,
focused = focusedContentColor,
disabled = disabledContentColor
),
focusedShadowColor = focusedShadowColor,
loadingTrackColor = loadingTrackColor,
loadingBarColor = loadingBarColor
)
/**
* Creates a [ButtonColors] instance that represents the colors of a text button
*/
@Composable
public fun textButtonColors(
borderColor: Color = Color.Unspecified,
pressedBorderColor: Color = Color.Unspecified,
focusedBorderColor: Color = Color.Unspecified,
disabledBorderColor: Color = Color.Unspecified,
backgroundColor: Color = Color.Unspecified,
pressedBackgroundColor: Color = Color.Unspecified,
focusedBackgroundColor: Color = Color.Unspecified,
disabledBackgroundColor: Color = Color.Unspecified,
contentColor: Color = PineTheme.colors.primary500,
pressedContentColor: Color = PineTheme.colors.primary500,
focusedContentColor: Color = PineTheme.colors.primary500,
disabledContentColor: Color = PineTheme.colors.grey400,
focusedShadowColor: Color = Color.Unspecified
): ButtonColors = ButtonColors(
borderColor = AnimatedInteractionStateColorSelector(
default = borderColor,
pressed = pressedBorderColor,
focused = focusedBorderColor,
disabled = disabledBorderColor
),
backgroundColor = AnimatedInteractionStateColorSelector(
default = backgroundColor,
pressed = pressedBackgroundColor,
focused = focusedBackgroundColor,
disabled = disabledBackgroundColor
),
contentColor = AnimatedInteractionStateColorSelector(
default = contentColor,
pressed = pressedContentColor,
focused = focusedContentColor,
disabled = disabledContentColor
),
focusedShadowColor = focusedShadowColor,
loadingTrackColor = Color.Unspecified,
loadingBarColor = Color.Unspecified
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy