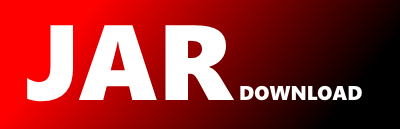
io.github.mmm.code.api.copy.AbstractCodeCopyMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mmm-code-api Show documentation
Show all versions of mmm-code-api Show documentation
The API to parse, analyze, transform and generate code.
/* Copyright (c) The m-m-m Team, Licensed under the Apache License, Version 2.0
* http://www.apache.org/licenses/LICENSE-2.0 */
package io.github.mmm.code.api.copy;
import java.util.HashMap;
import java.util.Map;
import io.github.mmm.base.exception.DuplicateObjectException;
import io.github.mmm.code.api.node.CodeNode;
/**
* Implementation of {@link CodeCopyMapper} for default mapping (copy only children).
*
* @author Joerg Hohwiller (hohwille at users.sourceforge.net)
* @since 1.0.0
*/
public abstract class AbstractCodeCopyMapper implements CodeCopyMapper {
private final Map mapping;
/**
* The constructor.
*/
public AbstractCodeCopyMapper() {
super();
this.mapping = new HashMap<>();
}
@SuppressWarnings("unchecked")
@Override
public N map(N node, CodeCopyType type) {
if (node == null) {
return null;
}
CodeNode result = getMapping(node);
if ((result == null) && !this.mapping.containsKey(node)) {
result = doMap(node, type);
}
return (N) result;
}
/**
* @param type of the given {@link CodeNode}.
* @param node the {@link CodeNode} to get from the existing mapping.
* @return the mapped {@link CodeNode} or {@code null} if no mapping is {@link #registerMapping(CodeNode, CodeNode)
* registered}.
*/
@SuppressWarnings("unchecked")
protected N getMapping(N node) {
return (N) this.mapping.get(node);
}
/**
* @see #map(CodeNode, CodeCopyType)
* @param type of the given {@link CodeNode}.
* @param node the {@link CodeNode} to map.
* @param type the {@link CodeCopyType} defining the relation of the {@link CodeNode} to map.
* @return the given {@link CodeNode} or a mapped replacement (e.g. a {@link CodeNodeItemCopyable#copy() copy} of it).
*/
protected abstract N doMap(N node, CodeCopyType type);
/**
* @param type of the given {@link CodeNode}s.
* @param source the original {@link CodeNode} to map.
* @param target the {@link CodeNode} copy to map to.
*/
public void registerMapping(N source, N target) {
CodeNode duplicate = this.mapping.put(source, target);
if (duplicate != null) {
throw new DuplicateObjectException(target, source, duplicate);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy