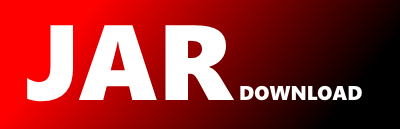
io.github.mmm.marshall.snakeyaml.impl.state.SnakeYamlArrayState Maven / Gradle / Ivy
package io.github.mmm.marshall.snakeyaml.impl.state;
import java.util.Collection;
import java.util.Iterator;
import io.github.mmm.marshall.StructuredReader.State;
/**
*
*/
public class SnakeYamlArrayState extends SnakeYamlParentState {
final Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy