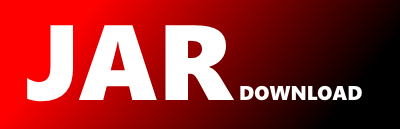
jaskell.util.Result Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaskell-java8 Show documentation
Show all versions of jaskell-java8 Show documentation
This is a utils library for java 8 project.
It include parsec combinators and sql generators library.
package jaskell.util;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.Optional;
import java.util.function.Function;
// TODO: type safe
@SuppressWarnings("unchecked")
public class Result implements Iterable, Iterator {
private final Object slot;
private final boolean _ok;
private int idx = 0;
public Result(T value) {
this.slot = value;
this._ok = true;
}
public Result(E error) {
this.slot = error;
this._ok = false;
}
public Optional ok(){
if(_ok){
return Optional.of((T)slot);
} else {
return Optional.empty();
}
}
public Optional err(){
if(_ok) {
return Optional.empty();
} else {
return Optional.of((E)slot);
}
}
public boolean isOk(){
return this._ok;
}
public boolean isErr(){
return !_ok;
}
public boolean equals(Object obj) {
if (!this.slot.getClass().isInstance(obj)) {
return false;
}
Result other = (Result) (obj);
return this.isOk() == other.isOk() && this.slot.equals(other.slot);
}
public Result map(Function super T, ? extends U> mapper) {
if (_ok) {
return new Result<>(mapper.apply((T)this.slot));
} else {
return new Result<>((E)this.slot);
}
}
public Result flatMap(Function super T, Result> mapper) {
if (_ok) {
return mapper.apply((T)this.slot);
} else {
return new Result<>((E) this.slot);
}
}
public T get() throws E{
if(this._ok) {
return (T)this.slot;
} else {
throw (E)this.slot;
}
}
public T orElse(T value) {
if(this._ok){
return (T)this.slot;
} else {
return value;
}
}
public T orElseGet(Result extends E, ?extends T> other) throws E {
if(this._ok){
return (T)this.slot;
} else {
return other.get();
}
}
@Override
public Iterator iterator() {
if(isOk()){
return new Result((T)slot);
} else {
return new Result((E)slot);
}
}
@Override
public boolean hasNext() {
return isOk() && idx == 0;
}
@Override
public T next() {
if (isOk() && idx == 0) {
idx ++;
return (T)slot;
} else {
throw new NoSuchElementException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy