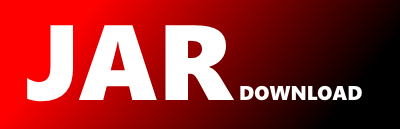
io.github.matteobertozzi.rednaco.collections.ImmutableCollections Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rednaco-core Show documentation
Show all versions of rednaco-core Show documentation
REDNACO Commons and Utils for Java.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.matteobertozzi.rednaco.collections;
import java.util.AbstractList;
import java.util.AbstractMap;
import java.util.AbstractSet;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import io.github.matteobertozzi.rednaco.collections.iterators.ArrayIterator;
public final class ImmutableCollections {
private ImmutableCollections() {
// no-op
}
public static List listOf(final T[] items) {
return new ImmutableList<>(items);
}
public static Set setOf(final T[] items) {
return new ImmutableArraySet<>(items);
}
public static Set setOf(final List items) {
return new ImmutableListSet<>(items);
}
public static Map mapOf(final K[] keys, final V[] values) {
return new ImmutableMap<>(new MapEntryWithKeyValArrays<>(keys, values));
}
private static final class ImmutableList extends AbstractList {
private final T[] items;
public ImmutableList(final T[] items) {
this.items = items;
}
@Override
public int size() {
return items.length;
}
@Override
public T get(final int index) {
return items[index];
}
@Override
public boolean addAll(final Collection extends T> c) {
throw new UnsupportedOperationException();
}
}
private static final class ImmutableArraySet extends AbstractSet {
private final T[] items;
private ImmutableArraySet(final T[] items) {
this.items = items;
}
@Override
public int size() {
return items.length;
}
@Override
public Iterator iterator() {
return new ArrayIterator<>(items);
}
@Override
public boolean addAll(final Collection extends T> c) {
throw new UnsupportedOperationException();
}
}
private static final class ImmutableListSet extends AbstractSet {
private final List items;
private ImmutableListSet(final List items) {
this.items = items;
}
@Override
public int size() {
return items.size();
}
@Override
public Iterator iterator() {
return items.iterator();
}
@Override
public boolean addAll(final Collection extends T> c) {
throw new UnsupportedOperationException();
}
}
private static final class ImmutableMap extends AbstractMap {
private final Set> entries;
public ImmutableMap(final Set> entries) {
this.entries = entries;
}
@Override
public Set> entrySet() {
return entries;
}
}
private static final class MapEntryWithKeyValArrays extends AbstractSet> {
private final K[] keys;
private final V[] vals;
public MapEntryWithKeyValArrays(final K[] keys, final V[] vals) {
this.keys = keys;
this.vals = vals;
}
@Override
public int size() {
return keys.length;
}
@Override
public Iterator> iterator() {
return new IteratorEntryWithKeyValArrays<>(keys, vals);
}
@Override
public boolean addAll(final Collection extends Entry> c) {
throw new UnsupportedOperationException();
}
}
private static final class IteratorEntryWithKeyValArrays implements Iterator> {
private final K[] keys;
private final V[] vals;
private int index;
public IteratorEntryWithKeyValArrays(final K[] keys, final V[] vals) {
this.keys = keys;
this.vals = vals;
this.index = 0;
}
@Override
public boolean hasNext() {
return index < keys.length;
}
@Override
public Entry next() {
final Entry entry = Map.entry(keys[index], vals[index]);
index++;
return entry;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy