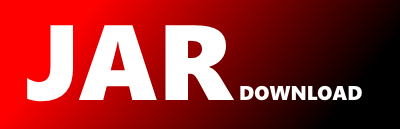
io.github.microcks.web.SoapController Maven / Gradle / Ivy
/*
* Licensed to Laurent Broudoux (the "Author") under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. Author licenses this
* file to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package io.github.microcks.web;
import io.github.microcks.domain.Operation;
import io.github.microcks.domain.Response;
import io.github.microcks.domain.Service;
import io.github.microcks.repository.ResponseRepository;
import io.github.microcks.repository.ServiceRepository;
import io.github.microcks.util.DispatchStyles;
import io.github.microcks.util.IdBuilder;
import io.github.microcks.util.SoapMessageValidator;
import io.github.microcks.util.soapui.SoapUIScriptEngineBinder;
import io.github.microcks.util.soapui.SoapUIXPathBuilder;
import org.apache.xmlbeans.XmlError;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.ApplicationContext;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import org.xml.sax.InputSource;
import javax.script.ScriptEngine;
import javax.script.ScriptEngineManager;
import javax.servlet.http.HttpServletRequest;
import javax.xml.xpath.XPathExpression;
import java.io.StringReader;
import java.util.List;
import java.util.regex.Pattern;
/**
* A controller for mocking Soap responses.
* @author laurent
*/
@org.springframework.web.bind.annotation.RestController
@RequestMapping("/soap")
public class SoapController {
/** A simple logger for diagnostic messages. */
private static Logger log = LoggerFactory.getLogger(SoapController.class);
@Autowired
private ServiceRepository serviceRepository;
@Autowired
private ResponseRepository responseRepository;
@Autowired
private ApplicationContext applicationContext;
@Value("${validation.resourceUrl}")
private final String resourceUrl = null;
@RequestMapping(value = "/{service}/{version}/**", method = RequestMethod.POST)
public ResponseEntity> execute(
@PathVariable("service") String serviceName,
@PathVariable("version") String version,
@RequestParam(value="validate", required=false) Boolean validate,
@RequestParam(value="delay", required=false) Long delay,
@RequestBody String body,
HttpServletRequest request
) {
log.info("Servicing mock response for service [{}, {}]", serviceName, version);
log.debug("Toto");
log.debug("Request body: " + body);
long startTime = System.currentTimeMillis();
// If serviceName was encoded with '+' instead of '%20', replace them.
if (serviceName.contains("+")) {
serviceName = serviceName.replace('+', ' ');
}
log.info("Service name: " + serviceName);
// Retrieve service and correct operation.
Service service = serviceRepository.findByNameAndVersion(serviceName, version);
Operation rOperation = null;
for (Operation operation : service.getOperations()) {
// Enhancement : try getting operation from soap:body directly!
if (hasPayloadCorrectStructureForOperation(body, operation.getInputName())) {
rOperation = operation;
log.info("Found valid operation {}", rOperation.getName());
break;
}
}
if (rOperation != null) {
log.debug("Found a valid operation with rules: {}", rOperation.getDispatcherRules());
if (validate != null && validate) {
log.debug("Soap message validation is turned on, validating...");
try {
List errors = SoapMessageValidator.validateSoapMessage(
rOperation.getInputName(), service.getXmlNS(), body,
resourceUrl + service.getName() + "-" + version + ".wsdl", true);
log.debug("SoapBody validation errors: " + errors.size());
// Return a 400 http code with errors.
if (errors != null && errors.size() > 0) {
return new ResponseEntity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy