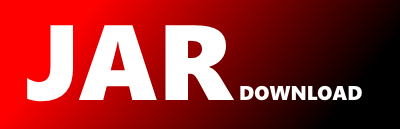
io.github.microcks.operator.api.artifact.v1alpha1.APISourceSpecFluent Maven / Gradle / Ivy
package io.github.microcks.operator.api.artifact.v1alpha1;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.Nested;
import java.util.ArrayList;
import java.lang.String;
import java.util.function.Predicate;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.Iterator;
import java.util.Collection;
import java.lang.Object;
import java.util.List;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class APISourceSpecFluent> extends BaseFluent{
public APISourceSpecFluent() {
}
public APISourceSpecFluent(APISourceSpec instance) {
this.copyInstance(instance);
}
private boolean keepAPIOnDelete;
private ArrayList artifacts;
private List importers;
protected void copyInstance(APISourceSpec instance) {
instance = (instance != null ? instance : new APISourceSpec());
if (instance != null) {
this.withKeepAPIOnDelete(instance.isKeepAPIOnDelete());
this.withArtifacts(instance.getArtifacts());
this.withImporters(instance.getImporters());
}
}
public boolean isKeepAPIOnDelete() {
return this.keepAPIOnDelete;
}
public A withKeepAPIOnDelete(boolean keepAPIOnDelete) {
this.keepAPIOnDelete = keepAPIOnDelete;
return (A) this;
}
public boolean hasKeepAPIOnDelete() {
return true;
}
public A addToArtifacts(int index,ArtifactSpec item) {
if (this.artifacts == null) {this.artifacts = new ArrayList();}
ArtifactSpecBuilder builder = new ArtifactSpecBuilder(item);
if (index < 0 || index >= artifacts.size()) { _visitables.get("artifacts").add(builder); artifacts.add(builder); } else { _visitables.get("artifacts").add(index, builder); artifacts.add(index, builder);}
return (A)this;
}
public A setToArtifacts(int index,ArtifactSpec item) {
if (this.artifacts == null) {this.artifacts = new ArrayList();}
ArtifactSpecBuilder builder = new ArtifactSpecBuilder(item);
if (index < 0 || index >= artifacts.size()) { _visitables.get("artifacts").add(builder); artifacts.add(builder); } else { _visitables.get("artifacts").set(index, builder); artifacts.set(index, builder);}
return (A)this;
}
public A addToArtifacts(io.github.microcks.operator.api.artifact.v1alpha1.ArtifactSpec... items) {
if (this.artifacts == null) {this.artifacts = new ArrayList();}
for (ArtifactSpec item : items) {ArtifactSpecBuilder builder = new ArtifactSpecBuilder(item);_visitables.get("artifacts").add(builder);this.artifacts.add(builder);} return (A)this;
}
public A addAllToArtifacts(Collection items) {
if (this.artifacts == null) {this.artifacts = new ArrayList();}
for (ArtifactSpec item : items) {ArtifactSpecBuilder builder = new ArtifactSpecBuilder(item);_visitables.get("artifacts").add(builder);this.artifacts.add(builder);} return (A)this;
}
public A removeFromArtifacts(io.github.microcks.operator.api.artifact.v1alpha1.ArtifactSpec... items) {
if (this.artifacts == null) return (A)this;
for (ArtifactSpec item : items) {ArtifactSpecBuilder builder = new ArtifactSpecBuilder(item);_visitables.get("artifacts").remove(builder); this.artifacts.remove(builder);} return (A)this;
}
public A removeAllFromArtifacts(Collection items) {
if (this.artifacts == null) return (A)this;
for (ArtifactSpec item : items) {ArtifactSpecBuilder builder = new ArtifactSpecBuilder(item);_visitables.get("artifacts").remove(builder); this.artifacts.remove(builder);} return (A)this;
}
public A removeMatchingFromArtifacts(Predicate predicate) {
if (artifacts == null) return (A) this;
final Iterator each = artifacts.iterator();
final List visitables = _visitables.get("artifacts");
while (each.hasNext()) {
ArtifactSpecBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildArtifacts() {
return this.artifacts != null ? build(artifacts) : null;
}
public ArtifactSpec buildArtifact(int index) {
return this.artifacts.get(index).build();
}
public ArtifactSpec buildFirstArtifact() {
return this.artifacts.get(0).build();
}
public ArtifactSpec buildLastArtifact() {
return this.artifacts.get(artifacts.size() - 1).build();
}
public ArtifactSpec buildMatchingArtifact(Predicate predicate) {
for (ArtifactSpecBuilder item : artifacts) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingArtifact(Predicate predicate) {
for (ArtifactSpecBuilder item : artifacts) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withArtifacts(List artifacts) {
if (this.artifacts != null) {
this._visitables.get("artifacts").clear();
}
if (artifacts != null) {
this.artifacts = new ArrayList();
for (ArtifactSpec item : artifacts) {
this.addToArtifacts(item);
}
} else {
this.artifacts = null;
}
return (A) this;
}
public A withArtifacts(io.github.microcks.operator.api.artifact.v1alpha1.ArtifactSpec... artifacts) {
if (this.artifacts != null) {
this.artifacts.clear();
_visitables.remove("artifacts");
}
if (artifacts != null) {
for (ArtifactSpec item : artifacts) {
this.addToArtifacts(item);
}
}
return (A) this;
}
public boolean hasArtifacts() {
return this.artifacts != null && !this.artifacts.isEmpty();
}
public ArtifactsNested addNewArtifact() {
return new ArtifactsNested(-1, null);
}
public ArtifactsNested addNewArtifactLike(ArtifactSpec item) {
return new ArtifactsNested(-1, item);
}
public ArtifactsNested setNewArtifactLike(int index,ArtifactSpec item) {
return new ArtifactsNested(index, item);
}
public ArtifactsNested editArtifact(int index) {
if (artifacts.size() <= index) throw new RuntimeException("Can't edit artifacts. Index exceeds size.");
return setNewArtifactLike(index, buildArtifact(index));
}
public ArtifactsNested editFirstArtifact() {
if (artifacts.size() == 0) throw new RuntimeException("Can't edit first artifacts. The list is empty.");
return setNewArtifactLike(0, buildArtifact(0));
}
public ArtifactsNested editLastArtifact() {
int index = artifacts.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last artifacts. The list is empty.");
return setNewArtifactLike(index, buildArtifact(index));
}
public ArtifactsNested editMatchingArtifact(Predicate predicate) {
int index = -1;
for (int i=0;i();}
this.importers.add(index, item);
return (A)this;
}
public A setToImporters(int index,ImporterSpec item) {
if (this.importers == null) {this.importers = new ArrayList();}
this.importers.set(index, item); return (A)this;
}
public A addToImporters(io.github.microcks.operator.api.artifact.v1alpha1.ImporterSpec... items) {
if (this.importers == null) {this.importers = new ArrayList();}
for (ImporterSpec item : items) {this.importers.add(item);} return (A)this;
}
public A addAllToImporters(Collection items) {
if (this.importers == null) {this.importers = new ArrayList();}
for (ImporterSpec item : items) {this.importers.add(item);} return (A)this;
}
public A removeFromImporters(io.github.microcks.operator.api.artifact.v1alpha1.ImporterSpec... items) {
if (this.importers == null) return (A)this;
for (ImporterSpec item : items) { this.importers.remove(item);} return (A)this;
}
public A removeAllFromImporters(Collection items) {
if (this.importers == null) return (A)this;
for (ImporterSpec item : items) { this.importers.remove(item);} return (A)this;
}
public List getImporters() {
return this.importers;
}
public ImporterSpec getImporter(int index) {
return this.importers.get(index);
}
public ImporterSpec getFirstImporter() {
return this.importers.get(0);
}
public ImporterSpec getLastImporter() {
return this.importers.get(importers.size() - 1);
}
public ImporterSpec getMatchingImporter(Predicate predicate) {
for (ImporterSpec item : importers) {
if (predicate.test(item)) {
return item;
}
}
return null;
}
public boolean hasMatchingImporter(Predicate predicate) {
for (ImporterSpec item : importers) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withImporters(List importers) {
if (importers != null) {
this.importers = new ArrayList();
for (ImporterSpec item : importers) {
this.addToImporters(item);
}
} else {
this.importers = null;
}
return (A) this;
}
public A withImporters(io.github.microcks.operator.api.artifact.v1alpha1.ImporterSpec... importers) {
if (this.importers != null) {
this.importers.clear();
_visitables.remove("importers");
}
if (importers != null) {
for (ImporterSpec item : importers) {
this.addToImporters(item);
}
}
return (A) this;
}
public boolean hasImporters() {
return this.importers != null && !this.importers.isEmpty();
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
APISourceSpecFluent that = (APISourceSpecFluent) o;
if (keepAPIOnDelete != that.keepAPIOnDelete) return false;
if (!java.util.Objects.equals(artifacts, that.artifacts)) return false;
if (!java.util.Objects.equals(importers, that.importers)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(keepAPIOnDelete, artifacts, importers, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
sb.append("keepAPIOnDelete:"); sb.append(keepAPIOnDelete + ",");
if (artifacts != null && !artifacts.isEmpty()) { sb.append("artifacts:"); sb.append(artifacts + ","); }
if (importers != null && !importers.isEmpty()) { sb.append("importers:"); sb.append(importers); }
sb.append("}");
return sb.toString();
}
public A withKeepAPIOnDelete() {
return withKeepAPIOnDelete(true);
}
public class ArtifactsNested extends ArtifactSpecFluent> implements Nested{
ArtifactsNested(int index,ArtifactSpec item) {
this.index = index;
this.builder = new ArtifactSpecBuilder(this, item);
}
ArtifactSpecBuilder builder;
int index;
public N and() {
return (N) APISourceSpecFluent.this.setToArtifacts(index,builder.build());
}
public N endArtifact() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy