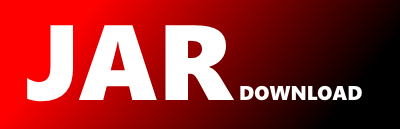
io.github.microcks.operator.api.base.v1alpha1.AICopilotSpecFluent Maven / Gradle / Ivy
package io.github.microcks.operator.api.base.v1alpha1;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import io.fabric8.kubernetes.api.builder.Nested;
import java.lang.Object;
import java.lang.String;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class AICopilotSpecFluent> extends BaseFluent{
public AICopilotSpecFluent() {
}
public AICopilotSpecFluent(AICopilotSpec instance) {
this.copyInstance(instance);
}
private boolean enabled;
private String implementation;
private OpenAISpecBuilder openai;
protected void copyInstance(AICopilotSpec instance) {
instance = (instance != null ? instance : new AICopilotSpec());
if (instance != null) {
this.withEnabled(instance.isEnabled());
this.withImplementation(instance.getImplementation());
this.withOpenai(instance.getOpenai());
}
}
public boolean isEnabled() {
return this.enabled;
}
public A withEnabled(boolean enabled) {
this.enabled = enabled;
return (A) this;
}
public boolean hasEnabled() {
return true;
}
public String getImplementation() {
return this.implementation;
}
public A withImplementation(String implementation) {
this.implementation = implementation;
return (A) this;
}
public boolean hasImplementation() {
return this.implementation != null;
}
public OpenAISpec buildOpenai() {
return this.openai != null ? this.openai.build() : null;
}
public A withOpenai(OpenAISpec openai) {
this._visitables.remove("openai");
if (openai != null) {
this.openai = new OpenAISpecBuilder(openai);
this._visitables.get("openai").add(this.openai);
} else {
this.openai = null;
this._visitables.get("openai").remove(this.openai);
}
return (A) this;
}
public boolean hasOpenai() {
return this.openai != null;
}
public OpenaiNested withNewOpenai() {
return new OpenaiNested(null);
}
public OpenaiNested withNewOpenaiLike(OpenAISpec item) {
return new OpenaiNested(item);
}
public OpenaiNested editOpenai() {
return withNewOpenaiLike(java.util.Optional.ofNullable(buildOpenai()).orElse(null));
}
public OpenaiNested editOrNewOpenai() {
return withNewOpenaiLike(java.util.Optional.ofNullable(buildOpenai()).orElse(new OpenAISpecBuilder().build()));
}
public OpenaiNested editOrNewOpenaiLike(OpenAISpec item) {
return withNewOpenaiLike(java.util.Optional.ofNullable(buildOpenai()).orElse(item));
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
AICopilotSpecFluent that = (AICopilotSpecFluent) o;
if (enabled != that.enabled) return false;
if (!java.util.Objects.equals(implementation, that.implementation)) return false;
if (!java.util.Objects.equals(openai, that.openai)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(enabled, implementation, openai, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
sb.append("enabled:"); sb.append(enabled + ",");
if (implementation != null) { sb.append("implementation:"); sb.append(implementation + ","); }
if (openai != null) { sb.append("openai:"); sb.append(openai); }
sb.append("}");
return sb.toString();
}
public A withEnabled() {
return withEnabled(true);
}
public class OpenaiNested extends OpenAISpecFluent> implements Nested{
OpenaiNested(OpenAISpec item) {
this.builder = new OpenAISpecBuilder(this, item);
}
OpenAISpecBuilder builder;
public N and() {
return (N) AICopilotSpecFluent.this.withOpenai(builder.build());
}
public N endOpenai() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy