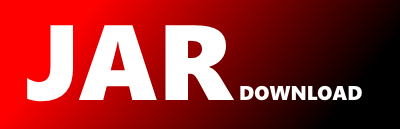
io.github.microcks.operator.api.base.v1alpha1.AmazonServiceConnectionSpecFluent Maven / Gradle / Ivy
package io.github.microcks.operator.api.base.v1alpha1;
import io.github.microcks.operator.api.model.SecretReferenceSpecBuilder;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.Nested;
import io.github.microcks.operator.api.model.SecretReferenceSpecFluent;
import java.lang.String;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.Object;
import io.github.microcks.operator.api.model.SecretReferenceSpec;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class AmazonServiceConnectionSpecFluent> extends BaseFluent{
public AmazonServiceConnectionSpecFluent() {
}
public AmazonServiceConnectionSpecFluent(AmazonServiceConnectionSpec instance) {
this.copyInstance(instance);
}
private String region;
private AmazonServiceConnectionSpec.AmazonCredentialsProviderType credentialsType;
private String credentialsProfile;
private SecretReferenceSpecBuilder credentialsSecretRef;
protected void copyInstance(AmazonServiceConnectionSpec instance) {
instance = (instance != null ? instance : new AmazonServiceConnectionSpec());
if (instance != null) {
this.withRegion(instance.getRegion());
this.withCredentialsType(instance.getCredentialsType());
this.withCredentialsProfile(instance.getCredentialsProfile());
this.withCredentialsSecretRef(instance.getCredentialsSecretRef());
}
}
public String getRegion() {
return this.region;
}
public A withRegion(String region) {
this.region = region;
return (A) this;
}
public boolean hasRegion() {
return this.region != null;
}
public AmazonServiceConnectionSpec.AmazonCredentialsProviderType getCredentialsType() {
return this.credentialsType;
}
public A withCredentialsType(AmazonServiceConnectionSpec.AmazonCredentialsProviderType credentialsType) {
this.credentialsType = credentialsType;
return (A) this;
}
public boolean hasCredentialsType() {
return this.credentialsType != null;
}
public String getCredentialsProfile() {
return this.credentialsProfile;
}
public A withCredentialsProfile(String credentialsProfile) {
this.credentialsProfile = credentialsProfile;
return (A) this;
}
public boolean hasCredentialsProfile() {
return this.credentialsProfile != null;
}
public SecretReferenceSpec buildCredentialsSecretRef() {
return this.credentialsSecretRef != null ? this.credentialsSecretRef.build() : null;
}
public A withCredentialsSecretRef(SecretReferenceSpec credentialsSecretRef) {
this._visitables.remove("credentialsSecretRef");
if (credentialsSecretRef != null) {
this.credentialsSecretRef = new SecretReferenceSpecBuilder(credentialsSecretRef);
this._visitables.get("credentialsSecretRef").add(this.credentialsSecretRef);
} else {
this.credentialsSecretRef = null;
this._visitables.get("credentialsSecretRef").remove(this.credentialsSecretRef);
}
return (A) this;
}
public boolean hasCredentialsSecretRef() {
return this.credentialsSecretRef != null;
}
public CredentialsSecretRefNested withNewCredentialsSecretRef() {
return new CredentialsSecretRefNested(null);
}
public CredentialsSecretRefNested withNewCredentialsSecretRefLike(SecretReferenceSpec item) {
return new CredentialsSecretRefNested(item);
}
public CredentialsSecretRefNested editCredentialsSecretRef() {
return withNewCredentialsSecretRefLike(java.util.Optional.ofNullable(buildCredentialsSecretRef()).orElse(null));
}
public CredentialsSecretRefNested editOrNewCredentialsSecretRef() {
return withNewCredentialsSecretRefLike(java.util.Optional.ofNullable(buildCredentialsSecretRef()).orElse(new SecretReferenceSpecBuilder().build()));
}
public CredentialsSecretRefNested editOrNewCredentialsSecretRefLike(SecretReferenceSpec item) {
return withNewCredentialsSecretRefLike(java.util.Optional.ofNullable(buildCredentialsSecretRef()).orElse(item));
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
AmazonServiceConnectionSpecFluent that = (AmazonServiceConnectionSpecFluent) o;
if (!java.util.Objects.equals(region, that.region)) return false;
if (!java.util.Objects.equals(credentialsType, that.credentialsType)) return false;
if (!java.util.Objects.equals(credentialsProfile, that.credentialsProfile)) return false;
if (!java.util.Objects.equals(credentialsSecretRef, that.credentialsSecretRef)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(region, credentialsType, credentialsProfile, credentialsSecretRef, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (region != null) { sb.append("region:"); sb.append(region + ","); }
if (credentialsType != null) { sb.append("credentialsType:"); sb.append(credentialsType + ","); }
if (credentialsProfile != null) { sb.append("credentialsProfile:"); sb.append(credentialsProfile + ","); }
if (credentialsSecretRef != null) { sb.append("credentialsSecretRef:"); sb.append(credentialsSecretRef); }
sb.append("}");
return sb.toString();
}
public class CredentialsSecretRefNested extends SecretReferenceSpecFluent> implements Nested{
CredentialsSecretRefNested(SecretReferenceSpec item) {
this.builder = new SecretReferenceSpecBuilder(this, item);
}
SecretReferenceSpecBuilder builder;
public N and() {
return (N) AmazonServiceConnectionSpecFluent.this.withCredentialsSecretRef(builder.build());
}
public N endCredentialsSecretRef() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy