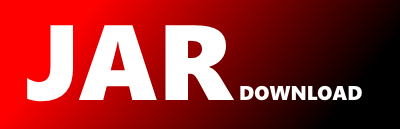
io.github.microcks.operator.api.base.v1alpha1.KafkaSpecFluent Maven / Gradle / Ivy
package io.github.microcks.operator.api.base.v1alpha1;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.Nested;
import java.lang.String;
import io.fabric8.kubernetes.api.model.ResourceRequirements;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.Object;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class KafkaSpecFluent> extends BaseFluent{
public KafkaSpecFluent() {
}
public KafkaSpecFluent(KafkaSpec instance) {
this.copyInstance(instance);
}
private boolean install;
private String url;
private String ingressClassName;
private boolean persistent;
private String volumeSize;
private SchemaRegistrySpecBuilder schemaRegistry;
private KafkaAuthenticationSpecBuilder authentication;
private ResourceRequirements resources;
private ResourceRequirements zkResources;
protected void copyInstance(KafkaSpec instance) {
instance = (instance != null ? instance : new KafkaSpec());
if (instance != null) {
this.withInstall(instance.isInstall());
this.withUrl(instance.getUrl());
this.withIngressClassName(instance.getIngressClassName());
this.withPersistent(instance.isPersistent());
this.withVolumeSize(instance.getVolumeSize());
this.withSchemaRegistry(instance.getSchemaRegistry());
this.withAuthentication(instance.getAuthentication());
this.withResources(instance.getResources());
this.withZkResources(instance.getZkResources());
}
}
public boolean isInstall() {
return this.install;
}
public A withInstall(boolean install) {
this.install = install;
return (A) this;
}
public boolean hasInstall() {
return true;
}
public String getUrl() {
return this.url;
}
public A withUrl(String url) {
this.url = url;
return (A) this;
}
public boolean hasUrl() {
return this.url != null;
}
public String getIngressClassName() {
return this.ingressClassName;
}
public A withIngressClassName(String ingressClassName) {
this.ingressClassName = ingressClassName;
return (A) this;
}
public boolean hasIngressClassName() {
return this.ingressClassName != null;
}
public boolean isPersistent() {
return this.persistent;
}
public A withPersistent(boolean persistent) {
this.persistent = persistent;
return (A) this;
}
public boolean hasPersistent() {
return true;
}
public String getVolumeSize() {
return this.volumeSize;
}
public A withVolumeSize(String volumeSize) {
this.volumeSize = volumeSize;
return (A) this;
}
public boolean hasVolumeSize() {
return this.volumeSize != null;
}
public SchemaRegistrySpec buildSchemaRegistry() {
return this.schemaRegistry != null ? this.schemaRegistry.build() : null;
}
public A withSchemaRegistry(SchemaRegistrySpec schemaRegistry) {
this._visitables.remove("schemaRegistry");
if (schemaRegistry != null) {
this.schemaRegistry = new SchemaRegistrySpecBuilder(schemaRegistry);
this._visitables.get("schemaRegistry").add(this.schemaRegistry);
} else {
this.schemaRegistry = null;
this._visitables.get("schemaRegistry").remove(this.schemaRegistry);
}
return (A) this;
}
public boolean hasSchemaRegistry() {
return this.schemaRegistry != null;
}
public SchemaRegistryNested withNewSchemaRegistry() {
return new SchemaRegistryNested(null);
}
public SchemaRegistryNested withNewSchemaRegistryLike(SchemaRegistrySpec item) {
return new SchemaRegistryNested(item);
}
public SchemaRegistryNested editSchemaRegistry() {
return withNewSchemaRegistryLike(java.util.Optional.ofNullable(buildSchemaRegistry()).orElse(null));
}
public SchemaRegistryNested editOrNewSchemaRegistry() {
return withNewSchemaRegistryLike(java.util.Optional.ofNullable(buildSchemaRegistry()).orElse(new SchemaRegistrySpecBuilder().build()));
}
public SchemaRegistryNested editOrNewSchemaRegistryLike(SchemaRegistrySpec item) {
return withNewSchemaRegistryLike(java.util.Optional.ofNullable(buildSchemaRegistry()).orElse(item));
}
public KafkaAuthenticationSpec buildAuthentication() {
return this.authentication != null ? this.authentication.build() : null;
}
public A withAuthentication(KafkaAuthenticationSpec authentication) {
this._visitables.remove("authentication");
if (authentication != null) {
this.authentication = new KafkaAuthenticationSpecBuilder(authentication);
this._visitables.get("authentication").add(this.authentication);
} else {
this.authentication = null;
this._visitables.get("authentication").remove(this.authentication);
}
return (A) this;
}
public boolean hasAuthentication() {
return this.authentication != null;
}
public AuthenticationNested withNewAuthentication() {
return new AuthenticationNested(null);
}
public AuthenticationNested withNewAuthenticationLike(KafkaAuthenticationSpec item) {
return new AuthenticationNested(item);
}
public AuthenticationNested editAuthentication() {
return withNewAuthenticationLike(java.util.Optional.ofNullable(buildAuthentication()).orElse(null));
}
public AuthenticationNested editOrNewAuthentication() {
return withNewAuthenticationLike(java.util.Optional.ofNullable(buildAuthentication()).orElse(new KafkaAuthenticationSpecBuilder().build()));
}
public AuthenticationNested editOrNewAuthenticationLike(KafkaAuthenticationSpec item) {
return withNewAuthenticationLike(java.util.Optional.ofNullable(buildAuthentication()).orElse(item));
}
public ResourceRequirements getResources() {
return this.resources;
}
public A withResources(ResourceRequirements resources) {
this.resources = resources;
return (A) this;
}
public boolean hasResources() {
return this.resources != null;
}
public ResourceRequirements getZkResources() {
return this.zkResources;
}
public A withZkResources(ResourceRequirements zkResources) {
this.zkResources = zkResources;
return (A) this;
}
public boolean hasZkResources() {
return this.zkResources != null;
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
KafkaSpecFluent that = (KafkaSpecFluent) o;
if (install != that.install) return false;
if (!java.util.Objects.equals(url, that.url)) return false;
if (!java.util.Objects.equals(ingressClassName, that.ingressClassName)) return false;
if (persistent != that.persistent) return false;
if (!java.util.Objects.equals(volumeSize, that.volumeSize)) return false;
if (!java.util.Objects.equals(schemaRegistry, that.schemaRegistry)) return false;
if (!java.util.Objects.equals(authentication, that.authentication)) return false;
if (!java.util.Objects.equals(resources, that.resources)) return false;
if (!java.util.Objects.equals(zkResources, that.zkResources)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(install, url, ingressClassName, persistent, volumeSize, schemaRegistry, authentication, resources, zkResources, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
sb.append("install:"); sb.append(install + ",");
if (url != null) { sb.append("url:"); sb.append(url + ","); }
if (ingressClassName != null) { sb.append("ingressClassName:"); sb.append(ingressClassName + ","); }
sb.append("persistent:"); sb.append(persistent + ",");
if (volumeSize != null) { sb.append("volumeSize:"); sb.append(volumeSize + ","); }
if (schemaRegistry != null) { sb.append("schemaRegistry:"); sb.append(schemaRegistry + ","); }
if (authentication != null) { sb.append("authentication:"); sb.append(authentication + ","); }
if (resources != null) { sb.append("resources:"); sb.append(resources + ","); }
if (zkResources != null) { sb.append("zkResources:"); sb.append(zkResources); }
sb.append("}");
return sb.toString();
}
public A withInstall() {
return withInstall(true);
}
public A withPersistent() {
return withPersistent(true);
}
public class SchemaRegistryNested extends SchemaRegistrySpecFluent> implements Nested{
SchemaRegistryNested(SchemaRegistrySpec item) {
this.builder = new SchemaRegistrySpecBuilder(this, item);
}
SchemaRegistrySpecBuilder builder;
public N and() {
return (N) KafkaSpecFluent.this.withSchemaRegistry(builder.build());
}
public N endSchemaRegistry() {
return and();
}
}
public class AuthenticationNested extends KafkaAuthenticationSpecFluent> implements Nested{
AuthenticationNested(KafkaAuthenticationSpec item) {
this.builder = new KafkaAuthenticationSpecBuilder(this, item);
}
KafkaAuthenticationSpecBuilder builder;
public N and() {
return (N) KafkaSpecFluent.this.withAuthentication(builder.build());
}
public N endAuthentication() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy