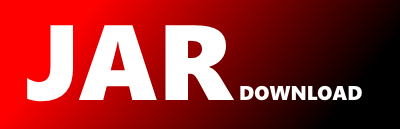
io.github.microcks.operator.api.base.v1alpha1.KeycloakSpecFluent Maven / Gradle / Ivy
package io.github.microcks.operator.api.base.v1alpha1;
import java.lang.SuppressWarnings;
import io.github.microcks.operator.api.model.ImageSpecFluent;
import io.fabric8.kubernetes.api.builder.Nested;
import java.lang.String;
import io.github.microcks.operator.api.model.ImageSpec;
import io.github.microcks.operator.api.model.IngressSpecFluent;
import java.util.LinkedHashMap;
import io.github.microcks.operator.api.model.IngressSpec;
import io.github.microcks.operator.api.model.ImageSpecBuilder;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import io.github.microcks.operator.api.model.OpenShiftSpecBuilder;
import io.github.microcks.operator.api.model.OpenShiftSpec;
import io.github.microcks.operator.api.model.IngressSpecBuilder;
import io.github.microcks.operator.api.model.OpenShiftSpecFluent;
import java.lang.Object;
import java.util.Map;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class KeycloakSpecFluent> extends BaseFluent{
public KeycloakSpecFluent() {
}
public KeycloakSpecFluent(KeycloakSpec instance) {
this.copyInstance(instance);
}
private boolean install;
private String realm;
private ImageSpecBuilder image;
private String url;
private String privateUrl;
private IngressSpecBuilder ingress;
private boolean persistent;
private ImageSpecBuilder postgresImage;
private String volumeSize;
private String storageClassName;
private Map pvcAnnotations;
private String serviceAccount;
private String serviceAccountCredentials;
private boolean operatorServiceAccountEnabled;
private OpenShiftSpecBuilder openshift;
protected void copyInstance(KeycloakSpec instance) {
instance = (instance != null ? instance : new KeycloakSpec());
if (instance != null) {
this.withInstall(instance.isInstall());
this.withRealm(instance.getRealm());
this.withImage(instance.getImage());
this.withUrl(instance.getUrl());
this.withPrivateUrl(instance.getPrivateUrl());
this.withIngress(instance.getIngress());
this.withPersistent(instance.isPersistent());
this.withPostgresImage(instance.getPostgresImage());
this.withVolumeSize(instance.getVolumeSize());
this.withStorageClassName(instance.getStorageClassName());
this.withPvcAnnotations(instance.getPvcAnnotations());
this.withServiceAccount(instance.getServiceAccount());
this.withServiceAccountCredentials(instance.getServiceAccountCredentials());
this.withOperatorServiceAccountEnabled(instance.isOperatorServiceAccountEnabled());
this.withOpenshift(instance.getOpenshift());
}
}
public boolean isInstall() {
return this.install;
}
public A withInstall(boolean install) {
this.install = install;
return (A) this;
}
public boolean hasInstall() {
return true;
}
public String getRealm() {
return this.realm;
}
public A withRealm(String realm) {
this.realm = realm;
return (A) this;
}
public boolean hasRealm() {
return this.realm != null;
}
public ImageSpec buildImage() {
return this.image != null ? this.image.build() : null;
}
public A withImage(ImageSpec image) {
this._visitables.remove("image");
if (image != null) {
this.image = new ImageSpecBuilder(image);
this._visitables.get("image").add(this.image);
} else {
this.image = null;
this._visitables.get("image").remove(this.image);
}
return (A) this;
}
public boolean hasImage() {
return this.image != null;
}
public ImageNested withNewImage() {
return new ImageNested(null);
}
public ImageNested withNewImageLike(ImageSpec item) {
return new ImageNested(item);
}
public ImageNested editImage() {
return withNewImageLike(java.util.Optional.ofNullable(buildImage()).orElse(null));
}
public ImageNested editOrNewImage() {
return withNewImageLike(java.util.Optional.ofNullable(buildImage()).orElse(new ImageSpecBuilder().build()));
}
public ImageNested editOrNewImageLike(ImageSpec item) {
return withNewImageLike(java.util.Optional.ofNullable(buildImage()).orElse(item));
}
public String getUrl() {
return this.url;
}
public A withUrl(String url) {
this.url = url;
return (A) this;
}
public boolean hasUrl() {
return this.url != null;
}
public String getPrivateUrl() {
return this.privateUrl;
}
public A withPrivateUrl(String privateUrl) {
this.privateUrl = privateUrl;
return (A) this;
}
public boolean hasPrivateUrl() {
return this.privateUrl != null;
}
public IngressSpec buildIngress() {
return this.ingress != null ? this.ingress.build() : null;
}
public A withIngress(IngressSpec ingress) {
this._visitables.remove("ingress");
if (ingress != null) {
this.ingress = new IngressSpecBuilder(ingress);
this._visitables.get("ingress").add(this.ingress);
} else {
this.ingress = null;
this._visitables.get("ingress").remove(this.ingress);
}
return (A) this;
}
public boolean hasIngress() {
return this.ingress != null;
}
public IngressNested withNewIngress() {
return new IngressNested(null);
}
public IngressNested withNewIngressLike(IngressSpec item) {
return new IngressNested(item);
}
public IngressNested editIngress() {
return withNewIngressLike(java.util.Optional.ofNullable(buildIngress()).orElse(null));
}
public IngressNested editOrNewIngress() {
return withNewIngressLike(java.util.Optional.ofNullable(buildIngress()).orElse(new IngressSpecBuilder().build()));
}
public IngressNested editOrNewIngressLike(IngressSpec item) {
return withNewIngressLike(java.util.Optional.ofNullable(buildIngress()).orElse(item));
}
public boolean isPersistent() {
return this.persistent;
}
public A withPersistent(boolean persistent) {
this.persistent = persistent;
return (A) this;
}
public boolean hasPersistent() {
return true;
}
public ImageSpec buildPostgresImage() {
return this.postgresImage != null ? this.postgresImage.build() : null;
}
public A withPostgresImage(ImageSpec postgresImage) {
this._visitables.remove("postgresImage");
if (postgresImage != null) {
this.postgresImage = new ImageSpecBuilder(postgresImage);
this._visitables.get("postgresImage").add(this.postgresImage);
} else {
this.postgresImage = null;
this._visitables.get("postgresImage").remove(this.postgresImage);
}
return (A) this;
}
public boolean hasPostgresImage() {
return this.postgresImage != null;
}
public PostgresImageNested withNewPostgresImage() {
return new PostgresImageNested(null);
}
public PostgresImageNested withNewPostgresImageLike(ImageSpec item) {
return new PostgresImageNested(item);
}
public PostgresImageNested editPostgresImage() {
return withNewPostgresImageLike(java.util.Optional.ofNullable(buildPostgresImage()).orElse(null));
}
public PostgresImageNested editOrNewPostgresImage() {
return withNewPostgresImageLike(java.util.Optional.ofNullable(buildPostgresImage()).orElse(new ImageSpecBuilder().build()));
}
public PostgresImageNested editOrNewPostgresImageLike(ImageSpec item) {
return withNewPostgresImageLike(java.util.Optional.ofNullable(buildPostgresImage()).orElse(item));
}
public String getVolumeSize() {
return this.volumeSize;
}
public A withVolumeSize(String volumeSize) {
this.volumeSize = volumeSize;
return (A) this;
}
public boolean hasVolumeSize() {
return this.volumeSize != null;
}
public String getStorageClassName() {
return this.storageClassName;
}
public A withStorageClassName(String storageClassName) {
this.storageClassName = storageClassName;
return (A) this;
}
public boolean hasStorageClassName() {
return this.storageClassName != null;
}
public A addToPvcAnnotations(String key,String value) {
if(this.pvcAnnotations == null && key != null && value != null) { this.pvcAnnotations = new LinkedHashMap(); }
if(key != null && value != null) {this.pvcAnnotations.put(key, value);} return (A)this;
}
public A addToPvcAnnotations(Map map) {
if(this.pvcAnnotations == null && map != null) { this.pvcAnnotations = new LinkedHashMap(); }
if(map != null) { this.pvcAnnotations.putAll(map);} return (A)this;
}
public A removeFromPvcAnnotations(String key) {
if(this.pvcAnnotations == null) { return (A) this; }
if(key != null && this.pvcAnnotations != null) {this.pvcAnnotations.remove(key);} return (A)this;
}
public A removeFromPvcAnnotations(Map map) {
if(this.pvcAnnotations == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.pvcAnnotations != null){this.pvcAnnotations.remove(key);}}} return (A)this;
}
public Map getPvcAnnotations() {
return this.pvcAnnotations;
}
public A withPvcAnnotations(Map pvcAnnotations) {
if (pvcAnnotations == null) {
this.pvcAnnotations = null;
} else {
this.pvcAnnotations = new LinkedHashMap(pvcAnnotations);
}
return (A) this;
}
public boolean hasPvcAnnotations() {
return this.pvcAnnotations != null;
}
public String getServiceAccount() {
return this.serviceAccount;
}
public A withServiceAccount(String serviceAccount) {
this.serviceAccount = serviceAccount;
return (A) this;
}
public boolean hasServiceAccount() {
return this.serviceAccount != null;
}
public String getServiceAccountCredentials() {
return this.serviceAccountCredentials;
}
public A withServiceAccountCredentials(String serviceAccountCredentials) {
this.serviceAccountCredentials = serviceAccountCredentials;
return (A) this;
}
public boolean hasServiceAccountCredentials() {
return this.serviceAccountCredentials != null;
}
public boolean isOperatorServiceAccountEnabled() {
return this.operatorServiceAccountEnabled;
}
public A withOperatorServiceAccountEnabled(boolean operatorServiceAccountEnabled) {
this.operatorServiceAccountEnabled = operatorServiceAccountEnabled;
return (A) this;
}
public boolean hasOperatorServiceAccountEnabled() {
return true;
}
public OpenShiftSpec buildOpenshift() {
return this.openshift != null ? this.openshift.build() : null;
}
public A withOpenshift(OpenShiftSpec openshift) {
this._visitables.remove("openshift");
if (openshift != null) {
this.openshift = new OpenShiftSpecBuilder(openshift);
this._visitables.get("openshift").add(this.openshift);
} else {
this.openshift = null;
this._visitables.get("openshift").remove(this.openshift);
}
return (A) this;
}
public boolean hasOpenshift() {
return this.openshift != null;
}
public OpenshiftNested withNewOpenshift() {
return new OpenshiftNested(null);
}
public OpenshiftNested withNewOpenshiftLike(OpenShiftSpec item) {
return new OpenshiftNested(item);
}
public OpenshiftNested editOpenshift() {
return withNewOpenshiftLike(java.util.Optional.ofNullable(buildOpenshift()).orElse(null));
}
public OpenshiftNested editOrNewOpenshift() {
return withNewOpenshiftLike(java.util.Optional.ofNullable(buildOpenshift()).orElse(new OpenShiftSpecBuilder().build()));
}
public OpenshiftNested editOrNewOpenshiftLike(OpenShiftSpec item) {
return withNewOpenshiftLike(java.util.Optional.ofNullable(buildOpenshift()).orElse(item));
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
KeycloakSpecFluent that = (KeycloakSpecFluent) o;
if (install != that.install) return false;
if (!java.util.Objects.equals(realm, that.realm)) return false;
if (!java.util.Objects.equals(image, that.image)) return false;
if (!java.util.Objects.equals(url, that.url)) return false;
if (!java.util.Objects.equals(privateUrl, that.privateUrl)) return false;
if (!java.util.Objects.equals(ingress, that.ingress)) return false;
if (persistent != that.persistent) return false;
if (!java.util.Objects.equals(postgresImage, that.postgresImage)) return false;
if (!java.util.Objects.equals(volumeSize, that.volumeSize)) return false;
if (!java.util.Objects.equals(storageClassName, that.storageClassName)) return false;
if (!java.util.Objects.equals(pvcAnnotations, that.pvcAnnotations)) return false;
if (!java.util.Objects.equals(serviceAccount, that.serviceAccount)) return false;
if (!java.util.Objects.equals(serviceAccountCredentials, that.serviceAccountCredentials)) return false;
if (operatorServiceAccountEnabled != that.operatorServiceAccountEnabled) return false;
if (!java.util.Objects.equals(openshift, that.openshift)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(install, realm, image, url, privateUrl, ingress, persistent, postgresImage, volumeSize, storageClassName, pvcAnnotations, serviceAccount, serviceAccountCredentials, operatorServiceAccountEnabled, openshift, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
sb.append("install:"); sb.append(install + ",");
if (realm != null) { sb.append("realm:"); sb.append(realm + ","); }
if (image != null) { sb.append("image:"); sb.append(image + ","); }
if (url != null) { sb.append("url:"); sb.append(url + ","); }
if (privateUrl != null) { sb.append("privateUrl:"); sb.append(privateUrl + ","); }
if (ingress != null) { sb.append("ingress:"); sb.append(ingress + ","); }
sb.append("persistent:"); sb.append(persistent + ",");
if (postgresImage != null) { sb.append("postgresImage:"); sb.append(postgresImage + ","); }
if (volumeSize != null) { sb.append("volumeSize:"); sb.append(volumeSize + ","); }
if (storageClassName != null) { sb.append("storageClassName:"); sb.append(storageClassName + ","); }
if (pvcAnnotations != null && !pvcAnnotations.isEmpty()) { sb.append("pvcAnnotations:"); sb.append(pvcAnnotations + ","); }
if (serviceAccount != null) { sb.append("serviceAccount:"); sb.append(serviceAccount + ","); }
if (serviceAccountCredentials != null) { sb.append("serviceAccountCredentials:"); sb.append(serviceAccountCredentials + ","); }
sb.append("operatorServiceAccountEnabled:"); sb.append(operatorServiceAccountEnabled + ",");
if (openshift != null) { sb.append("openshift:"); sb.append(openshift); }
sb.append("}");
return sb.toString();
}
public A withInstall() {
return withInstall(true);
}
public A withPersistent() {
return withPersistent(true);
}
public A withOperatorServiceAccountEnabled() {
return withOperatorServiceAccountEnabled(true);
}
public class ImageNested extends ImageSpecFluent> implements Nested{
ImageNested(ImageSpec item) {
this.builder = new ImageSpecBuilder(this, item);
}
ImageSpecBuilder builder;
public N and() {
return (N) KeycloakSpecFluent.this.withImage(builder.build());
}
public N endImage() {
return and();
}
}
public class IngressNested extends IngressSpecFluent> implements Nested{
IngressNested(IngressSpec item) {
this.builder = new IngressSpecBuilder(this, item);
}
IngressSpecBuilder builder;
public N and() {
return (N) KeycloakSpecFluent.this.withIngress(builder.build());
}
public N endIngress() {
return and();
}
}
public class PostgresImageNested extends ImageSpecFluent> implements Nested{
PostgresImageNested(ImageSpec item) {
this.builder = new ImageSpecBuilder(this, item);
}
ImageSpecBuilder builder;
public N and() {
return (N) KeycloakSpecFluent.this.withPostgresImage(builder.build());
}
public N endPostgresImage() {
return and();
}
}
public class OpenshiftNested extends OpenShiftSpecFluent> implements Nested{
OpenshiftNested(OpenShiftSpec item) {
this.builder = new OpenShiftSpecBuilder(this, item);
}
OpenShiftSpecBuilder builder;
public N and() {
return (N) KeycloakSpecFluent.this.withOpenshift(builder.build());
}
public N endOpenshift() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy