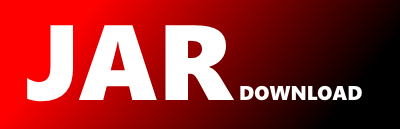
io.github.microcks.operator.api.base.v1alpha1.MicrocksSpecFluent Maven / Gradle / Ivy
package io.github.microcks.operator.api.base.v1alpha1;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.Nested;
import java.util.ArrayList;
import java.lang.String;
import java.util.LinkedHashMap;
import java.util.function.Predicate;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.List;
import io.fabric8.kubernetes.api.model.Toleration;
import java.lang.Long;
import io.fabric8.kubernetes.api.model.Affinity;
import java.util.Collection;
import java.lang.Object;
import java.util.Map;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class MicrocksSpecFluent> extends BaseFluent{
public MicrocksSpecFluent() {
}
public MicrocksSpecFluent(MicrocksSpec instance) {
this.copyInstance(instance);
}
private String version;
private MicrocksServiceSpecBuilder microcks;
private PostmanRuntimeSpecBuilder postman;
private KeycloakSpecBuilder keycloak;
private FeaturesSpecBuilder features;
private Map commonLabels;
private Map commonAnnotations;
private Affinity commonAffinities;
private List commonTolerations;
protected void copyInstance(MicrocksSpec instance) {
instance = (instance != null ? instance : new MicrocksSpec());
if (instance != null) {
this.withVersion(instance.getVersion());
this.withMicrocks(instance.getMicrocks());
this.withPostman(instance.getPostman());
this.withKeycloak(instance.getKeycloak());
this.withFeatures(instance.getFeatures());
this.withCommonLabels(instance.getCommonLabels());
this.withCommonAnnotations(instance.getCommonAnnotations());
this.withCommonAffinities(instance.getCommonAffinities());
this.withCommonTolerations(instance.getCommonTolerations());
}
}
public String getVersion() {
return this.version;
}
public A withVersion(String version) {
this.version = version;
return (A) this;
}
public boolean hasVersion() {
return this.version != null;
}
public MicrocksServiceSpec buildMicrocks() {
return this.microcks != null ? this.microcks.build() : null;
}
public A withMicrocks(MicrocksServiceSpec microcks) {
this._visitables.remove("microcks");
if (microcks != null) {
this.microcks = new MicrocksServiceSpecBuilder(microcks);
this._visitables.get("microcks").add(this.microcks);
} else {
this.microcks = null;
this._visitables.get("microcks").remove(this.microcks);
}
return (A) this;
}
public boolean hasMicrocks() {
return this.microcks != null;
}
public MicrocksNested withNewMicrocks() {
return new MicrocksNested(null);
}
public MicrocksNested withNewMicrocksLike(MicrocksServiceSpec item) {
return new MicrocksNested(item);
}
public MicrocksNested editMicrocks() {
return withNewMicrocksLike(java.util.Optional.ofNullable(buildMicrocks()).orElse(null));
}
public MicrocksNested editOrNewMicrocks() {
return withNewMicrocksLike(java.util.Optional.ofNullable(buildMicrocks()).orElse(new MicrocksServiceSpecBuilder().build()));
}
public MicrocksNested editOrNewMicrocksLike(MicrocksServiceSpec item) {
return withNewMicrocksLike(java.util.Optional.ofNullable(buildMicrocks()).orElse(item));
}
public PostmanRuntimeSpec buildPostman() {
return this.postman != null ? this.postman.build() : null;
}
public A withPostman(PostmanRuntimeSpec postman) {
this._visitables.remove("postman");
if (postman != null) {
this.postman = new PostmanRuntimeSpecBuilder(postman);
this._visitables.get("postman").add(this.postman);
} else {
this.postman = null;
this._visitables.get("postman").remove(this.postman);
}
return (A) this;
}
public boolean hasPostman() {
return this.postman != null;
}
public PostmanNested withNewPostman() {
return new PostmanNested(null);
}
public PostmanNested withNewPostmanLike(PostmanRuntimeSpec item) {
return new PostmanNested(item);
}
public PostmanNested editPostman() {
return withNewPostmanLike(java.util.Optional.ofNullable(buildPostman()).orElse(null));
}
public PostmanNested editOrNewPostman() {
return withNewPostmanLike(java.util.Optional.ofNullable(buildPostman()).orElse(new PostmanRuntimeSpecBuilder().build()));
}
public PostmanNested editOrNewPostmanLike(PostmanRuntimeSpec item) {
return withNewPostmanLike(java.util.Optional.ofNullable(buildPostman()).orElse(item));
}
public KeycloakSpec buildKeycloak() {
return this.keycloak != null ? this.keycloak.build() : null;
}
public A withKeycloak(KeycloakSpec keycloak) {
this._visitables.remove("keycloak");
if (keycloak != null) {
this.keycloak = new KeycloakSpecBuilder(keycloak);
this._visitables.get("keycloak").add(this.keycloak);
} else {
this.keycloak = null;
this._visitables.get("keycloak").remove(this.keycloak);
}
return (A) this;
}
public boolean hasKeycloak() {
return this.keycloak != null;
}
public KeycloakNested withNewKeycloak() {
return new KeycloakNested(null);
}
public KeycloakNested withNewKeycloakLike(KeycloakSpec item) {
return new KeycloakNested(item);
}
public KeycloakNested editKeycloak() {
return withNewKeycloakLike(java.util.Optional.ofNullable(buildKeycloak()).orElse(null));
}
public KeycloakNested editOrNewKeycloak() {
return withNewKeycloakLike(java.util.Optional.ofNullable(buildKeycloak()).orElse(new KeycloakSpecBuilder().build()));
}
public KeycloakNested editOrNewKeycloakLike(KeycloakSpec item) {
return withNewKeycloakLike(java.util.Optional.ofNullable(buildKeycloak()).orElse(item));
}
public FeaturesSpec buildFeatures() {
return this.features != null ? this.features.build() : null;
}
public A withFeatures(FeaturesSpec features) {
this._visitables.remove("features");
if (features != null) {
this.features = new FeaturesSpecBuilder(features);
this._visitables.get("features").add(this.features);
} else {
this.features = null;
this._visitables.get("features").remove(this.features);
}
return (A) this;
}
public boolean hasFeatures() {
return this.features != null;
}
public FeaturesNested withNewFeatures() {
return new FeaturesNested(null);
}
public FeaturesNested withNewFeaturesLike(FeaturesSpec item) {
return new FeaturesNested(item);
}
public FeaturesNested editFeatures() {
return withNewFeaturesLike(java.util.Optional.ofNullable(buildFeatures()).orElse(null));
}
public FeaturesNested editOrNewFeatures() {
return withNewFeaturesLike(java.util.Optional.ofNullable(buildFeatures()).orElse(new FeaturesSpecBuilder().build()));
}
public FeaturesNested editOrNewFeaturesLike(FeaturesSpec item) {
return withNewFeaturesLike(java.util.Optional.ofNullable(buildFeatures()).orElse(item));
}
public A addToCommonLabels(String key,String value) {
if(this.commonLabels == null && key != null && value != null) { this.commonLabels = new LinkedHashMap(); }
if(key != null && value != null) {this.commonLabels.put(key, value);} return (A)this;
}
public A addToCommonLabels(Map map) {
if(this.commonLabels == null && map != null) { this.commonLabels = new LinkedHashMap(); }
if(map != null) { this.commonLabels.putAll(map);} return (A)this;
}
public A removeFromCommonLabels(String key) {
if(this.commonLabels == null) { return (A) this; }
if(key != null && this.commonLabels != null) {this.commonLabels.remove(key);} return (A)this;
}
public A removeFromCommonLabels(Map map) {
if(this.commonLabels == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.commonLabels != null){this.commonLabels.remove(key);}}} return (A)this;
}
public Map getCommonLabels() {
return this.commonLabels;
}
public A withCommonLabels(Map commonLabels) {
if (commonLabels == null) {
this.commonLabels = null;
} else {
this.commonLabels = new LinkedHashMap(commonLabels);
}
return (A) this;
}
public boolean hasCommonLabels() {
return this.commonLabels != null;
}
public A addToCommonAnnotations(String key,String value) {
if(this.commonAnnotations == null && key != null && value != null) { this.commonAnnotations = new LinkedHashMap(); }
if(key != null && value != null) {this.commonAnnotations.put(key, value);} return (A)this;
}
public A addToCommonAnnotations(Map map) {
if(this.commonAnnotations == null && map != null) { this.commonAnnotations = new LinkedHashMap(); }
if(map != null) { this.commonAnnotations.putAll(map);} return (A)this;
}
public A removeFromCommonAnnotations(String key) {
if(this.commonAnnotations == null) { return (A) this; }
if(key != null && this.commonAnnotations != null) {this.commonAnnotations.remove(key);} return (A)this;
}
public A removeFromCommonAnnotations(Map map) {
if(this.commonAnnotations == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.commonAnnotations != null){this.commonAnnotations.remove(key);}}} return (A)this;
}
public Map getCommonAnnotations() {
return this.commonAnnotations;
}
public A withCommonAnnotations(Map commonAnnotations) {
if (commonAnnotations == null) {
this.commonAnnotations = null;
} else {
this.commonAnnotations = new LinkedHashMap(commonAnnotations);
}
return (A) this;
}
public boolean hasCommonAnnotations() {
return this.commonAnnotations != null;
}
public Affinity getCommonAffinities() {
return this.commonAffinities;
}
public A withCommonAffinities(Affinity commonAffinities) {
this.commonAffinities = commonAffinities;
return (A) this;
}
public boolean hasCommonAffinities() {
return this.commonAffinities != null;
}
public A addToCommonTolerations(int index,Toleration item) {
if (this.commonTolerations == null) {this.commonTolerations = new ArrayList();}
this.commonTolerations.add(index, item);
return (A)this;
}
public A setToCommonTolerations(int index,Toleration item) {
if (this.commonTolerations == null) {this.commonTolerations = new ArrayList();}
this.commonTolerations.set(index, item); return (A)this;
}
public A addToCommonTolerations(io.fabric8.kubernetes.api.model.Toleration... items) {
if (this.commonTolerations == null) {this.commonTolerations = new ArrayList();}
for (Toleration item : items) {this.commonTolerations.add(item);} return (A)this;
}
public A addAllToCommonTolerations(Collection items) {
if (this.commonTolerations == null) {this.commonTolerations = new ArrayList();}
for (Toleration item : items) {this.commonTolerations.add(item);} return (A)this;
}
public A removeFromCommonTolerations(io.fabric8.kubernetes.api.model.Toleration... items) {
if (this.commonTolerations == null) return (A)this;
for (Toleration item : items) { this.commonTolerations.remove(item);} return (A)this;
}
public A removeAllFromCommonTolerations(Collection items) {
if (this.commonTolerations == null) return (A)this;
for (Toleration item : items) { this.commonTolerations.remove(item);} return (A)this;
}
public List getCommonTolerations() {
return this.commonTolerations;
}
public Toleration getCommonToleration(int index) {
return this.commonTolerations.get(index);
}
public Toleration getFirstCommonToleration() {
return this.commonTolerations.get(0);
}
public Toleration getLastCommonToleration() {
return this.commonTolerations.get(commonTolerations.size() - 1);
}
public Toleration getMatchingCommonToleration(Predicate predicate) {
for (Toleration item : commonTolerations) {
if (predicate.test(item)) {
return item;
}
}
return null;
}
public boolean hasMatchingCommonToleration(Predicate predicate) {
for (Toleration item : commonTolerations) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withCommonTolerations(List commonTolerations) {
if (commonTolerations != null) {
this.commonTolerations = new ArrayList();
for (Toleration item : commonTolerations) {
this.addToCommonTolerations(item);
}
} else {
this.commonTolerations = null;
}
return (A) this;
}
public A withCommonTolerations(io.fabric8.kubernetes.api.model.Toleration... commonTolerations) {
if (this.commonTolerations != null) {
this.commonTolerations.clear();
_visitables.remove("commonTolerations");
}
if (commonTolerations != null) {
for (Toleration item : commonTolerations) {
this.addToCommonTolerations(item);
}
}
return (A) this;
}
public boolean hasCommonTolerations() {
return this.commonTolerations != null && !this.commonTolerations.isEmpty();
}
public A addNewCommonToleration(String effect,String key,String operator,Long tolerationSeconds,String value) {
return (A)addToCommonTolerations(new Toleration(effect, key, operator, tolerationSeconds, value));
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
MicrocksSpecFluent that = (MicrocksSpecFluent) o;
if (!java.util.Objects.equals(version, that.version)) return false;
if (!java.util.Objects.equals(microcks, that.microcks)) return false;
if (!java.util.Objects.equals(postman, that.postman)) return false;
if (!java.util.Objects.equals(keycloak, that.keycloak)) return false;
if (!java.util.Objects.equals(features, that.features)) return false;
if (!java.util.Objects.equals(commonLabels, that.commonLabels)) return false;
if (!java.util.Objects.equals(commonAnnotations, that.commonAnnotations)) return false;
if (!java.util.Objects.equals(commonAffinities, that.commonAffinities)) return false;
if (!java.util.Objects.equals(commonTolerations, that.commonTolerations)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(version, microcks, postman, keycloak, features, commonLabels, commonAnnotations, commonAffinities, commonTolerations, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (version != null) { sb.append("version:"); sb.append(version + ","); }
if (microcks != null) { sb.append("microcks:"); sb.append(microcks + ","); }
if (postman != null) { sb.append("postman:"); sb.append(postman + ","); }
if (keycloak != null) { sb.append("keycloak:"); sb.append(keycloak + ","); }
if (features != null) { sb.append("features:"); sb.append(features + ","); }
if (commonLabels != null && !commonLabels.isEmpty()) { sb.append("commonLabels:"); sb.append(commonLabels + ","); }
if (commonAnnotations != null && !commonAnnotations.isEmpty()) { sb.append("commonAnnotations:"); sb.append(commonAnnotations + ","); }
if (commonAffinities != null) { sb.append("commonAffinities:"); sb.append(commonAffinities + ","); }
if (commonTolerations != null && !commonTolerations.isEmpty()) { sb.append("commonTolerations:"); sb.append(commonTolerations); }
sb.append("}");
return sb.toString();
}
public class MicrocksNested extends MicrocksServiceSpecFluent> implements Nested{
MicrocksNested(MicrocksServiceSpec item) {
this.builder = new MicrocksServiceSpecBuilder(this, item);
}
MicrocksServiceSpecBuilder builder;
public N and() {
return (N) MicrocksSpecFluent.this.withMicrocks(builder.build());
}
public N endMicrocks() {
return and();
}
}
public class PostmanNested extends PostmanRuntimeSpecFluent> implements Nested{
PostmanNested(PostmanRuntimeSpec item) {
this.builder = new PostmanRuntimeSpecBuilder(this, item);
}
PostmanRuntimeSpecBuilder builder;
public N and() {
return (N) MicrocksSpecFluent.this.withPostman(builder.build());
}
public N endPostman() {
return and();
}
}
public class KeycloakNested extends KeycloakSpecFluent> implements Nested{
KeycloakNested(KeycloakSpec item) {
this.builder = new KeycloakSpecBuilder(this, item);
}
KeycloakSpecBuilder builder;
public N and() {
return (N) MicrocksSpecFluent.this.withKeycloak(builder.build());
}
public N endKeycloak() {
return and();
}
}
public class FeaturesNested extends FeaturesSpecFluent> implements Nested{
FeaturesNested(FeaturesSpec item) {
this.builder = new FeaturesSpecBuilder(this, item);
}
FeaturesSpecBuilder builder;
public N and() {
return (N) MicrocksSpecFluent.this.withFeatures(builder.build());
}
public N endFeatures() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy