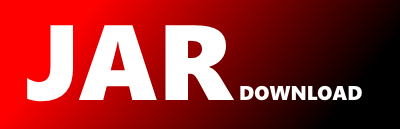
io.microsphere.lang.function.ThrowableFunction Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.microsphere.lang.function;
import java.util.Objects;
import java.util.function.BiFunction;
import java.util.function.Function;
import static java.util.Objects.requireNonNull;
/**
* {@link Function} with {@link Throwable}
*
* @param the source type
* @param the return type
* @see Function
* @see Throwable
* @since 1.0.0
*/
@FunctionalInterface
public interface ThrowableFunction {
/**
* Applies this function to the given argument.
*
* @param t the function argument
* @return the function result
* @throws Throwable if met with any error
*/
R apply(T t) throws Throwable;
/**
* Executes {@link #apply(T)} with {@link #handleException(T, Throwable) the default exception handling}
*
* @param t the function argument
* @return the function result
*/
default R execute(T t) throws RuntimeException {
return execute(t, this::handleException);
}
/**
* Executes {@link #apply(T)} with the customized exception handling
*
* @param t the function argument
* @param exceptionHandler the handler to handle the function argument and
* the exception that the {@link #apply(T)} method throws
* @return the function result
*/
default R execute(T t, BiFunction exceptionHandler) throws RuntimeException {
R result = null;
try {
result = apply(t);
} catch (Throwable e) {
result = exceptionHandler.apply(t, e);
}
return result;
}
/**
* Handle any exception that the {@link #apply(T)} method throws
*
* @param t the value to be consumed
* @param failure the instance of {@link Throwable}
*/
default R handleException(T t, Throwable failure) {
throw new RuntimeException(failure);
}
/**
* Returns a composed throwable-function that first applies the {@code before}
* throwable-function to its input, and then applies this throwable-function to the result.
* If evaluation of either throwable-function throws an exception, it is relayed to
* the caller of the composed throwable-function.
*
* @param the type of input to the {@code before} throwable-function, and to the
* composed throwable-function
* @param before the throwable-function to apply before this throwable-function is applied
* @return a composed throwable-function that first applies the {@code before}
* throwable-function and then applies this throwable-function
* @throws NullPointerException if before is null
* @see #andThen(ThrowableFunction)
*/
default ThrowableFunction compose(ThrowableFunction super V, ? extends T> before) {
Objects.requireNonNull(before);
return (V v) -> apply(before.apply(v));
}
/**
* Returns a composed throwable-function that first applies this throwable-function to
* its input, and then applies the {@code after} throwable-function to the result.
* If evaluation of either throwable-function throws an exception, it is relayed to
* the caller of the composed throwable-function.
*
* @param the type of output of the {@code after} throwable-function, and of the
* composed throwable-function
* @param after the throwable-function to apply after this throwable-function is applied
* @return a composed throwable-function that first applies this throwable-function and then
* applies the {@code after} throwable-function
* @throws NullPointerException if after is null
* @see #compose(ThrowableFunction)
*/
default ThrowableFunction andThen(ThrowableFunction super R, ? extends V> after) {
Objects.requireNonNull(after);
return (T t) -> after.apply(apply(t));
}
/**
* Executes {@link ThrowableFunction} with {@link #handleException(T, Throwable) the default exception handling}
*
* @param t the throwable-function argument
* @param function {@link ThrowableFunction}
* @param the source type
* @param the return type
* @return the result after execution
* @throws NullPointerException if function
is null
*/
static R execute(T t, ThrowableFunction function) throws NullPointerException {
requireNonNull(function, "The function must not be null");
return function.execute(t);
}
/**
* Executes {@link ThrowableFunction} with the customized exception handling
*
* @param t the function argument
* @param exceptionHandler the handler to handle the function argument and
* the exception that the {@link #apply(T)} method throws
* @param function {@link ThrowableFunction}
* @param the source type
* @param the return type
* @return the result after execution
* @throws NullPointerException if function
and exceptionHandler
is null
*/
static R execute(T t, ThrowableFunction function, BiFunction exceptionHandler)
throws NullPointerException {
requireNonNull(function, "The function must not be null");
requireNonNull(exceptionHandler, "The exceptionHandler must not be null");
return function.execute(t, exceptionHandler);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy