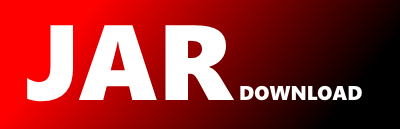
io.microsphere.util.Assert Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.microsphere.util;
import io.microsphere.collection.CollectionUtils;
import io.microsphere.collection.MapUtils;
import javax.annotation.Nullable;
import java.util.Collection;
import java.util.Map;
import java.util.function.Supplier;
/**
* The utility class for Assertion
*
* @author Mercy
* @since 1.0.0
*/
public abstract class Assert extends BaseUtils {
/**
* Assert a boolean expression, throwing an {@code IllegalArgumentException}
* if the expression evaluates to {@code false}.
* assertTrue(i > 0, "The value must be greater than zero");
*
* @param expression a boolean expression
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if {@code expression} is {@code false}
*/
public static void assertTrue(boolean expression, String message) {
if (!expression) {
throw new IllegalArgumentException(message);
}
}
/**
* Assert a boolean expression, throwing an {@code IllegalArgumentException}
* if the expression evaluates to {@code false}.
*
* assertTrue(i > 0, () -> "The value '" + i + "' must be greater than zero");
*
*
* @param expression a boolean expression
* @param messageSupplier a supplier for the exception message to use if the
* assertion fails
* @throws IllegalArgumentException if {@code expression} is {@code false}
*/
public static void assertTrue(boolean expression, Supplier messageSupplier) {
if (!expression) {
throw new IllegalArgumentException(nullSafeGet(messageSupplier));
}
}
/**
* Assert that an object is {@code null}.
* assertNull(value, "The value must be null");
*
* @param object the object to check
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if the object is not {@code null}
*/
public static void assertNull(@Nullable Object object, String message) {
if (object != null) {
throw new IllegalArgumentException(message);
}
}
/**
* Assert that an object is {@code null}.
*
* assertNull(value, () -> "The value '" + value + "' must be null");
*
*
* @param object the object to check
* @param messageSupplier a supplier for the exception message to use if the
* assertion fails
* @throws IllegalArgumentException if the object is not {@code null}
*/
public static void assertNull(@Nullable Object object, Supplier messageSupplier) {
if (object != null) {
throw new IllegalArgumentException(nullSafeGet(messageSupplier));
}
}
/**
* Assert that an object is not {@code null}.
* assertNotNull(clazz, "The class must not be null");
*
* @param object the object to check
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if the object is {@code null}
*/
public static void assertNotNull(@Nullable Object object, String message) {
if (object == null) {
throw new IllegalArgumentException(message);
}
}
/**
* Assert that an object is not {@code null}.
*
* assertNotNull(entity.getId(), () -> "ID for entity " + entity.getName() + " must not be null");
*
*
* @param object the object to check
* @param messageSupplier a supplier for the exception message to use if the
* assertion fails
* @throws IllegalArgumentException if the object is {@code null}
*/
public static void assertNotNull(@Nullable Object object, Supplier messageSupplier) {
if (object == null) {
throw new IllegalArgumentException(nullSafeGet(messageSupplier));
}
}
/**
* Assert that a string is not empty ("").
*
* assertNotEmpty(entity.getName(), "Name for entity " + entity.getName() + " must not be empty");
*
*
* @param text the text to check
* @param message the exception message to use if the
* assertion fails
*/
public static void assertNotEmpty(@Nullable String text, String message) {
if (StringUtils.isEmpty(text)) {
throw new IllegalArgumentException(message);
}
}
/**
* Assert that a string is not empty ("").
*
* assertNotEmpty(entity.getName(), () -> "Name for entity " + entity.getName() + " must not be empty");
*
*
* @param text the text to check
* @param messageSupplier a supplier for the exception message to use if the
* assertion fails
*/
public static void assertNotEmpty(@Nullable String text, Supplier messageSupplier) {
if (StringUtils.isEmpty(text)) {
throw new IllegalArgumentException(nullSafeGet(messageSupplier));
}
}
/**
* Assert that a string is not blank.
*
* assertNotBlank(entity.getName(), "Name for entity " + entity.getName() + " must not be blank");
*
*
* @param text the text to check
* @param message the exception message to use if the
* assertion fails
*/
public static void assertNotBlank(@Nullable String text, String message) {
if (StringUtils.isBlank(text)) {
throw new IllegalArgumentException(message);
}
}
/**
* Assert that a string is not blank.
*
* assertNotBlank(entity.getName(), () -> "Name for entity " + entity.getName() + " must not be blank");
*
*
* @param text the text to check
* @param messageSupplier a supplier for the exception message to use if the
* assertion fails
*/
public static void assertNotBlank(@Nullable String text, Supplier messageSupplier) {
if (StringUtils.isBlank(text)) {
throw new IllegalArgumentException(nullSafeGet(messageSupplier));
}
}
/**
* Assert that an array contains elements; that is, it must not be
* {@code null} and must contain at least one element.
* assertNotEmpty(array, "The array must contain elements");
*
* @param array the array to check
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if the object array is {@code null} or contains no elements
*/
public static void assertNotEmpty(@Nullable Object[] array, String message) {
if (ArrayUtils.isEmpty(array)) {
throw new IllegalArgumentException(message);
}
}
/**
* Assert that an array contains elements; that is, it must not be
* {@code null} and must contain at least one element.
*
* assertNotEmpty(array, () -> "The " + arrayType + " array must contain elements");
*
*
* @param array the array to check
* @param messageSupplier a supplier for the exception message to use if the
* assertion fails
* @throws IllegalArgumentException if the object array is {@code null} or contains no elements
*/
public static void assertNotEmpty(@Nullable Object[] array, Supplier messageSupplier) {
if (ArrayUtils.isEmpty(array)) {
throw new IllegalArgumentException(nullSafeGet(messageSupplier));
}
}
/**
* Assert that a collection contains elements; that is, it must not be
* {@code null} and must contain at least one element.
* assertNotEmpty(collection, "Collection must contain elements");
*
* @param collection the collection to check
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if the collection is {@code null} or
* contains no elements
*/
public static void assertNotEmpty(@Nullable Collection> collection, String message) {
if (CollectionUtils.isEmpty(collection)) {
throw new IllegalArgumentException(message);
}
}
/**
* Assert that a collection contains elements; that is, it must not be
* {@code null} and must contain at least one element.
*
* assertNotEmpty(collection, () -> "The " + collectionType + " collection must contain elements");
*
*
* @param collection the collection to check
* @param messageSupplier a supplier for the exception message to use if the
* assertion fails
* @throws IllegalArgumentException if the collection is {@code null} or
* contains no elements
*/
public static void assertNotEmpty(@Nullable Collection> collection, Supplier messageSupplier) {
if (CollectionUtils.isEmpty(collection)) {
throw new IllegalArgumentException(nullSafeGet(messageSupplier));
}
}
/**
* Assert that a Map contains entries; that is, it must not be {@code null}
* and must contain at least one entry.
* assertNotEmpty(map, "Map must contain entries");
*
* @param map the map to check
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if the map is {@code null} or contains no entries
*/
public static void assertNotEmpty(@Nullable Map, ?> map, String message) {
if (MapUtils.isEmpty(map)) {
throw new IllegalArgumentException(message);
}
}
/**
* Assert that a Map contains entries; that is, it must not be {@code null}
* and must contain at least one entry.
*
* assertNotEmpty(map, () -> "The " + mapType + " map must contain entries");
*
*
* @param map the map to check
* @param messageSupplier a supplier for the exception message to use if the
* assertion fails
* @throws IllegalArgumentException if the map is {@code null} or contains no entries
*/
public static void assertNotEmpty(@Nullable Map, ?> map, Supplier messageSupplier) {
if (MapUtils.isEmpty(map)) {
throw new IllegalArgumentException(nullSafeGet(messageSupplier));
}
}
/**
* Assert that an array contains no {@code null} elements.
* Note: Does not complain if the array is empty!
*
assertNoNullElements(array, "The array must contain non-null elements");
*
* @param array the array to check
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if the object array contains a {@code null} element
*/
public static void assertNoNullElements(@Nullable Object[] array, String message) {
if (array != null) {
for (Object element : array) {
if (element == null) {
throw new IllegalArgumentException(message);
}
}
}
}
/**
* Assert that an array contains no {@code null} elements.
* Note: Does not complain if the array is empty!
*
* assertNoNullElements(array, () -> "The " + arrayType + " array must contain non-null elements");
*
*
* @param array the array to check
* @param messageSupplier a supplier for the exception message to use if the
* assertion fails
* @throws IllegalArgumentException if the object array contains a {@code null} element
*/
public static void assertNoNullElements(@Nullable Object[] array, Supplier messageSupplier) {
if (array != null) {
for (Object element : array) {
if (element == null) {
throw new IllegalArgumentException(nullSafeGet(messageSupplier));
}
}
}
}
/**
* Assert that a elements contains no {@code null} elements.
* Note: Does not complain if the elements is empty!
*
assertNoNullElements(elements, "Elements must contain non-null elements");
*
* @param elements the elements to check
* @param message the exception message to use if the assertion fails
* @throws IllegalArgumentException if the elements contains a {@code null} element
*/
public static void assertNoNullElements(@Nullable Iterable> elements, String message) {
if (elements != null) {
for (Object element : elements) {
if (element == null) {
throw new IllegalArgumentException(message);
}
}
}
}
/**
* Assert that a elements contains no {@code null} elements.
* Note: Does not complain if the elements is empty!
*
* assertNoNullElements(elements, () -> "Collection " + collectionName + " must contain non-null elements");
*
*
* @param elements the elements to check
* @param messageSupplier a supplier for the exception message to use if the
* assertion fails
* @throws IllegalArgumentException if the elements contains a {@code null} element
*/
public static void assertNoNullElements(@Nullable Iterable> elements, Supplier messageSupplier) {
if (elements != null) {
for (Object element : elements) {
if (element == null) {
throw new IllegalArgumentException(nullSafeGet(messageSupplier));
}
}
}
}
private static String nullSafeGet(Supplier messageSupplier) {
return messageSupplier == null ? null : messageSupplier.get();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy