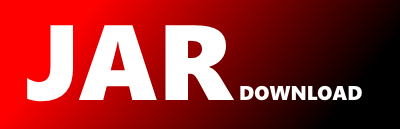
com.epam.reportportal.service.launch.SecondaryLaunch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of client-java Show documentation
Show all versions of client-java Show documentation
A application used as an example on how to set up pushing its components to the Central Repository .
The newest version!
/*
* Copyright 2020 EPAM Systems
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.epam.reportportal.service.launch;
import com.epam.reportportal.listeners.ListenerParameters;
import com.epam.reportportal.service.Launch;
import com.epam.reportportal.service.LaunchIdLock;
import com.epam.reportportal.service.LaunchLoggingContext;
import com.epam.reportportal.service.ReportPortalClient;
import com.epam.reportportal.utils.Waiter;
import com.epam.ta.reportportal.ws.model.FinishExecutionRQ;
import com.epam.ta.reportportal.ws.model.launch.LaunchResource;
import io.reactivex.Completable;
import io.reactivex.Maybe;
import io.reactivex.disposables.Disposable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.annotation.Nonnull;
import java.util.Queue;
import java.util.concurrent.Callable;
import java.util.concurrent.ConcurrentLinkedQueue;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.TimeUnit;
/**
* The class represents a {@link Launch} which reports into existing one and never starts its own.
*/
public class SecondaryLaunch extends AbstractJoinedLaunch {
private static final Logger LOGGER = LoggerFactory.getLogger(SecondaryLaunch.class);
private final ReportPortalClient client;
public SecondaryLaunch(ReportPortalClient rpClient, ListenerParameters parameters, Maybe launch,
ExecutorService executorService, LaunchIdLock launchIdLock, String instanceUuid) {
super(rpClient, parameters, launch, executorService, launchIdLock, instanceUuid);
client = rpClient;
}
private void waitForLaunchStart() {
new Waiter("Wait for Launch start").pollingEvery(1, TimeUnit.SECONDS).timeoutFail().till(new Callable() {
private volatile Boolean result = null;
private final Queue disposables = new ConcurrentLinkedQueue<>();
@Override
public Boolean call() {
if (result == null) {
disposables.add(launch.subscribe(uuid -> {
Maybe maybeRs = client.getLaunchByUuid(uuid);
if (maybeRs != null) {
disposables.add(maybeRs.subscribe(
launchResource -> result = Boolean.TRUE,
throwable -> LOGGER.debug("Unable to get a Launch: " + throwable.getLocalizedMessage(), throwable)
));
} else {
LOGGER.debug("RP Client returned 'null' response on get Launch by UUID call");
}
}));
} else {
Disposable disposable;
while ((disposable = disposables.poll()) != null) {
disposable.dispose();
}
}
return result;
}
});
}
@Nonnull
@Override
public Maybe start() {
waitForLaunchStart();
return super.start();
}
@Override
public void finish(final FinishExecutionRQ rq) {
QUEUE.getUnchecked(launch).addToQueue(LaunchLoggingContext.complete());
Throwable throwable = Completable.concat(QUEUE.getUnchecked(this.launch).getChildren())
.timeout(getParameters().getReportingTimeout(), TimeUnit.SECONDS)
.blockingGet();
if (throwable != null) {
LOGGER.error("Unable to finish secondary launch in ReportPortal", throwable);
}
// ignore super call, since only primary launch should finish it
stopRunning();
lock.finishInstanceUuid(uuid);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy