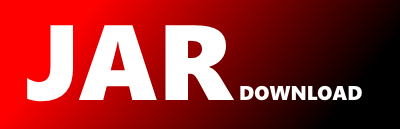
com.epam.reportportal.service.tree.TestItemTree Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of client-java Show documentation
Show all versions of client-java Show documentation
A application used as an example on how to set up pushing its components to the Central Repository .
The newest version!
/*
* Copyright 2019 EPAM Systems
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.epam.reportportal.service.tree;
import com.epam.reportportal.listeners.ItemStatus;
import com.epam.reportportal.listeners.ItemType;
import com.epam.ta.reportportal.ws.model.OperationCompletionRS;
import io.reactivex.Maybe;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.Collections;
import java.util.Map;
import java.util.Objects;
import java.util.concurrent.ConcurrentHashMap;
/**
* Tree for holding test items structure to provide {@link TestItemLeaf} retrieving by {@link ItemTreeKey}
* for API calls using {@link ItemTreeReporter}.
*
* @author Ivan Budayeu
*/
public class TestItemTree {
private Maybe launchId;
private final Map testItems;
public TestItemTree() {
this.testItems = new ConcurrentHashMap<>();
}
/**
* Create a Test Item Leaf for a tree of Test Items
*
* @param itemId an ID of the leaf
* @return a leaf object
*/
public static TestItemTree.TestItemLeaf createTestItemLeaf(Maybe itemId) {
return new TestItemTree.TestItemLeaf(itemId);
}
/**
* Create a Test Item Leaf for a tree of Test Items
*
* @param parentId an ID of a parent Test Item (leaf)
* @param itemId an ID of the leaf
* @return a leaf object
*/
public static TestItemTree.TestItemLeaf createTestItemLeaf(Maybe parentId, Maybe itemId) {
return new TestItemTree.TestItemLeaf(parentId, itemId);
}
/**
* Create a Test Item Leaf for a tree of Test Items
*
* @param itemId an ID of the leaf
* @param childItems child leaf elements
* @return a leaf object
*/
public static TestItemTree.TestItemLeaf createTestItemLeaf(Maybe itemId,
Map childItems) {
return new TestItemTree.TestItemLeaf(itemId, Collections.emptyMap(), childItems);
}
/**
* Create a Test Item Leaf for a tree of Test Items
*
* @param itemId an ID of the leaf
* @param childItems child leaf elements
* @param attributes leaf attributes
* @return a leaf object
*/
public static TestItemTree.TestItemLeaf createTestItemLeaf(Maybe itemId,
Map childItems, Map attributes) {
return new TestItemTree.TestItemLeaf(itemId, attributes, childItems);
}
/**
* Create a Test Item Leaf for a tree of Test Items
*
* @param parentId an ID of a parent Test Item (leaf)
* @param itemId an ID of the leaf
* @param childItems child leaf elements
* @return a leaf object
*/
public static TestItemTree.TestItemLeaf createTestItemLeaf(Maybe parentId, Maybe itemId,
Map childItems) {
return new TestItemTree.TestItemLeaf(parentId, itemId, Collections.emptyMap(), childItems);
}
/**
* Create a Test Item Leaf for a tree of Test Items
*
* @param parentId an ID of a parent Test Item (leaf)
* @param itemId an ID of the leaf
* @param childItems child leaf elements
* @param attributes leaf attributes
* @return a leaf object
*/
public static TestItemTree.TestItemLeaf createTestItemLeaf(Maybe parentId, Maybe itemId,
Map childItems, Map attributes) {
return new TestItemTree.TestItemLeaf(parentId, itemId, attributes, childItems);
}
public Maybe getLaunchId() {
return launchId;
}
public void setLaunchId(Maybe launchId) {
this.launchId = launchId;
}
public Map getTestItems() {
return testItems;
}
/**
* Key for test items structure storing in the {@link TestItemTree}
*/
public static final class ItemTreeKey {
private final String name;
private final int hash;
private ItemTreeKey(String name, int hash) {
this.name = name;
this.hash = hash;
}
private ItemTreeKey(String name) {
this(name, name != null ? name.hashCode() : 0);
}
public String getName() {
return name;
}
public int getHash() {
return hash;
}
public static ItemTreeKey of(String name) {
return new ItemTreeKey(name);
}
public static ItemTreeKey of(String name, int hash) {
return new ItemTreeKey(name, hash);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ItemTreeKey that = (ItemTreeKey) o;
if (hash != that.hash) {
return false;
}
return Objects.equals(name, that.name);
}
@Override
public int hashCode() {
int result = name != null ? name.hashCode() : 0;
result = 31 * result + hash;
return result;
}
}
/**
* Class represents test item with links on parent and descendants. Contains item id and finish response promises for reporting
* using {@link ItemTreeReporter}.
* Finish response is required to provide correct request ordering:
* 1) default finish response from agent
* 2) callback requests that will be sent only after default finish response is returned or if it's NULL
*/
public static class TestItemLeaf {
@Nullable
private Maybe parentId;
@Nullable
private Maybe finishResponse;
private final Maybe itemId;
private final Map childItems = new ConcurrentHashMap<>();
private final Map attributes = new ConcurrentHashMap<>();
private ItemStatus status;
private ItemType type;
private TestItemLeaf(Maybe itemId) {
this.itemId = itemId;
}
private TestItemLeaf(Maybe itemId, Map attributes) {
this(itemId);
this.attributes.putAll(attributes);
}
private TestItemLeaf(Maybe itemId, Map attributes, Map childItems) {
this(itemId, attributes);
this.childItems.putAll(childItems);
}
private TestItemLeaf(@Nullable Maybe parentId, Maybe itemId) {
this(itemId);
this.parentId = parentId;
}
private TestItemLeaf(@Nullable Maybe parentId, Maybe itemId, Map attributes) {
this(itemId, attributes);
this.parentId = parentId;
}
private TestItemLeaf(@Nullable Maybe parentId, Maybe itemId, Map attributes,
Map childItems) {
this(itemId, parentId, attributes);
this.childItems.putAll(childItems);
}
@Nullable
public Maybe getParentId() {
return parentId;
}
public void setParentId(@Nullable Maybe parentId) {
this.parentId = parentId;
}
@Nullable
public Maybe getFinishResponse() {
return finishResponse;
}
public void setFinishResponse(@Nullable Maybe finishResponse) {
this.finishResponse = finishResponse;
}
public Maybe getItemId() {
return itemId;
}
@Nonnull
public Map getChildItems() {
return childItems;
}
public ItemStatus getStatus() {
return status;
}
public void setStatus(ItemStatus status) {
this.status = status;
}
public ItemType getType() {
return type;
}
public void setType(ItemType type) {
this.type = type;
}
@Nullable
@SuppressWarnings("unchecked")
public T getAttribute(String key) {
return (T) attributes.get(key);
}
@Nullable
public Object setAttribute(String key, Object value) {
return attributes.put(key, value);
}
@Nullable
public Object clearAttribute(String key) {
return attributes.remove(key);
}
public Map getAttributes() {
return Collections.unmodifiableMap(attributes);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy