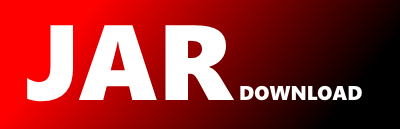
assertions.Assertions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AUTOTESTIMATIC-JAVA Show documentation
Show all versions of AUTOTESTIMATIC-JAVA Show documentation
An open-source Selenium Java-based Test automation Framework that allows you to perform multiple actions
to test a web application's functionality, behaviour, which provides easy to use syntax,
and easy to set up environment according to the needed requirements for testing
package assertions;
import org.openqa.selenium.By;
import org.openqa.selenium.NoSuchElementException;
import org.openqa.selenium.TimeoutException;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.FluentWait;
import org.testng.asserts.Assertion;
public class Assertions {
private final Assertion assertion;
private final ThreadLocal driverThreadLocal = new ThreadLocal<>();
private final FluentWait driverWait;
public Assertions(WebDriver driver, FluentWait wait){
driverThreadLocal.set(driver);
assertion = new Assertion();
this.driverWait = wait;
}
public ElementAssertions element(By by){
try{
this.driverWait.until(ExpectedConditions.visibilityOfElementLocated(by));
}
catch (TimeoutException exception){
throw new NoSuchElementException("Element doesn't exist");
}
return new ElementAssertions(this.assertion, by, driverThreadLocal.get());
}
public BrowserAssertions browser(){
return new BrowserAssertions(this.assertion, driverThreadLocal.get());
}
public ObjectAssertions object(Object object) {
return new ObjectAssertions(this.assertion, object, driverThreadLocal.get());
}
public void removeDriver(){
driverThreadLocal.remove();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy