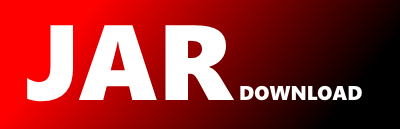
assertions.ObjectAssertions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AUTOTESTIMATIC-JAVA Show documentation
Show all versions of AUTOTESTIMATIC-JAVA Show documentation
An open-source Selenium Java-based Test automation Framework that allows you to perform multiple actions
to test a web application's functionality, behaviour, which provides easy to use syntax,
and easy to set up environment according to the needed requirements for testing
package assertions;
import org.openqa.selenium.WebDriver;
import org.testng.asserts.Assertion;
import utilities.LoggingManager;
public class ObjectAssertions {
private final Assertion assertion;
private final ThreadLocal driverThreadLocal = new ThreadLocal<>();
private final Object actualObject;
public ObjectAssertions(Assertion assertion, Object object, WebDriver driver){
this.assertion = assertion;
this.actualObject = object;
driverThreadLocal.set(driver);
}
public void contains(Object expected){
try {
assertion.assertTrue(actualObject.toString().contains(expected.toString()));
LoggingManager.info("Expected: " + expected + ", Actual: " + actualObject.toString());
}
catch (AssertionError e){
LoggingManager.error("Expected: " + expected + ", but Actual: " + actualObject.toString());
throw e;
}
}
public void doesNotContain(Object expected){
try {
assertion.assertTrue(actualObject.toString().contains(expected.toString()));
LoggingManager.info("Expected: " + expected + ", Actual: " + actualObject.toString());
}
catch (AssertionError e){
LoggingManager.error("Expected: " + expected + ", but Actual: " + actualObject.toString());
throw e;
}
}
public void isNotEqualTo(Object expected){
try {
assertion.assertTrue(!actualObject.toString().equalsIgnoreCase(expected.toString()));
LoggingManager.info("Expected: " + expected + ", Actual: " + actualObject.toString());
}
catch (AssertionError e){
LoggingManager.error("Expected: " + expected + ", but Actual: " + actualObject.toString());
throw e;
}
}
public void isEqualTo(Object expected){
try {
assertion.assertTrue(actualObject.toString().equalsIgnoreCase(expected.toString()));
LoggingManager.info("Expected: " + expected + ", Actual: " + actualObject.toString());
}
catch (AssertionError e){
LoggingManager.error("Expected: " + expected + ", but Actual: " + actualObject.toString());
throw e;
}
}
public void removeDriver(){
driverThreadLocal.remove();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy