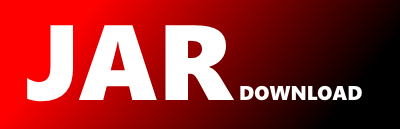
io.github.molumn.catrix.common.Interactions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of catrix-api Show documentation
Show all versions of catrix-api Show documentation
Kotlin/JVM library for terminal command development
package io.github.molumn.catrix.common
import com.github.ajalt.mordant.rendering.TextColors
import com.github.ajalt.mordant.rendering.TextColors.*
import com.github.ajalt.mordant.rendering.TextStyle
import com.github.ajalt.mordant.rendering.TextStyles
import com.github.ajalt.mordant.rendering.TextStyles.*
import io.github.molumn.catrix.common.exceptions.CatrixIntentionalException
interface Interactive {
companion object : Interactive by Interactions()
fun shutdown() {
throw CatrixIntentionalException()
}
fun getColor() : TextStyle?
fun getBackground() : TextStyle?
fun getStyles() : TextStyle?
fun getDepth() : Int
fun up()
fun down()
fun printDepth()
fun group(group: Interactive.() -> Interactive) : Interactive
fun send(message: String) : Interactive
fun react(message: String) : Interactive
fun trace(message: String) : Interactive
fun info(message: String) : Interactive
fun warn(message: String) : Interactive
fun error(message: String) : Interactive
fun fatal(message: String)
fun color(r: Int, g: Int, b: Int) : Interactive
fun hsv(h: Int, s: Int, v: Int) : Interactive
fun styles(style: TextStyle) : Interactive
fun backgroundC(r: Int, g: Int, b: Int) : Interactive
fun backgroundH(h: Int, s: Int, v: Int) : Interactive
fun bold(message: String? = null) : Interactive
fun dim(message: String? = null) : Interactive
fun italic(message: String? = null) : Interactive
fun inverse(message: String? = null) : Interactive
fun underline(message: String? = null) : Interactive
fun strikethrough(message: String? = null) : Interactive
fun input(message: String = " >> ") : String?
fun enter() : Interactive
/**
* return to default status [depth: 0]
*/
fun home() : Interactive
fun reset() : Interactive
}
class Interactions : Interactive {
private var color1: TextStyle? = white
override fun getColor(): TextStyle? = color1
private var background: TextStyle? = null
override fun getBackground(): TextStyle? = background
private var styles1: TextStyle? = null
override fun getStyles(): TextStyle? = styles1
private var depth: Int = 0
override fun getDepth(): Int = depth
override fun up() {
depth -= 1
}
override fun down() {
depth += 1
}
override fun printDepth() {
for (i in (0 until depth)) {
print(" ")
}
}
override fun group(group: Interactive.() -> Interactive): Interactive {
down()
group(this)
up()
return this
}
override fun send(message: String): Interactive {
printDepth()
println(
message.run {
color1?.let { color ->
background?.let { background ->
(color on background)(this)
} ?: color(this)
} ?: background?.let { background ->
background(this)
} ?: message
}
)
return this
}
override fun react(message: String): Interactive {
printDepth()
print(" => ")
println(
message.run {
color1?.let { color ->
background?.let { background ->
(color on background)(this)
} ?: color(this)
} ?: background?.let { background ->
background(this)
} ?: message
}
)
return this
}
override fun trace(message: String): Interactive {
printDepth()
println(gray(message))
return this
}
override fun info(message: String): Interactive {
printDepth()
println((white + bold)(message))
return this
}
override fun warn(message: String): Interactive {
printDepth()
println((yellow + bold)(message))
return this
}
override fun error(message: String): Interactive {
printDepth()
println((red + bold)(message))
return this
}
override fun fatal(message: String) {
printDepth()
println((bold + brightRed on red)(message))
}
override fun color(r: Int, g: Int, b: Int): Interactive {
color1 = TextColors.Companion.rgb(r, g, b)
return this
}
override fun hsv(h: Int, s: Int, v: Int): Interactive {
color1 = TextColors.Companion.hsv(h, s, v)
return this
}
override fun styles(style: TextStyle): Interactive {
styles1 = style
return this
}
override fun backgroundC(r: Int, g: Int, b: Int): Interactive {
background = TextColors.Companion.rgb(r, g, b)
return this
}
override fun backgroundH(h: Int, s: Int, v: Int): Interactive {
color1 = TextColors.Companion.hsv(h, s, v)
return this
}
override fun bold(message: String?): Interactive {
message?.let {
send(TextStyles.bold(message))
} ?: run { styles1 = styles1?.let { it + bold } ?: bold.style }
return this
}
override fun dim(message: String?): Interactive {
message?.let {
send(TextStyles.dim(message))
} ?: run { styles1 = styles1?.let { it + dim } ?: dim.style }
return this
}
override fun italic(message: String?): Interactive {
message?.let {
send(TextStyles.italic(message))
} ?: run { styles1 = styles1?.let { it + italic } ?: italic.style }
return this
}
override fun inverse(message: String?): Interactive {
message?.let {
send(TextStyles.inverse(message))
} ?: run { styles1 = styles1?.let { it + inverse } ?: inverse.style }
return this
}
override fun underline(message: String?): Interactive {
message?.let {
send(TextStyles.underline(message))
} ?: run { styles1 = styles1?.let { it + underline } ?: underline.style }
return this
}
override fun strikethrough(message: String?): Interactive {
message?.let {
send(TextStyles.strikethrough(message))
} ?: run { styles1 = styles1?.let { it + strikethrough } ?: strikethrough.style }
return this
}
override fun input(message: String): String? {
print(message)
return readLine()
}
override fun enter(): Interactive {
println()
return this
}
override fun home(): Interactive {
depth = 0
println()
return this
}
override fun reset(): Interactive {
color1 = null
background = null
styles1 = null
return this
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy