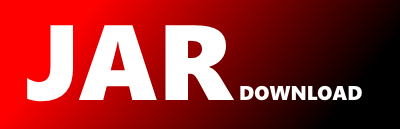
io.github.monun.kommand.loader.LibraryLoader.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kommand-api Show documentation
Show all versions of kommand-api Show documentation
Kotlin DSL for PaperMC commands
/*
* Kommand
* Copyright (C) 2021 Monun
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package io.github.monun.kommand.loader
import org.bukkit.Bukkit
import java.lang.reflect.InvocationTargetException
object LibraryLoader {
@Suppress("UNCHECKED_CAST")
fun loadImplement(type: Class, vararg initArgs: Any? = emptyArray()): T {
val packageName = type.`package`.name
val className = "${type.simpleName}Impl"
val parameterTypes = initArgs.map { it?.javaClass }.toTypedArray()
return try {
val internalClass =
Class.forName("$packageName.internal.$className", true, type.classLoader).asSubclass(type)
val constructor = kotlin.runCatching {
internalClass.getConstructor(*parameterTypes)
}.getOrNull() ?: throw UnsupportedOperationException("${type.name} does not have Constructor for [${parameterTypes.joinToString()}]")
constructor.newInstance(*initArgs) as T
} catch (exception: ClassNotFoundException) {
throw UnsupportedOperationException("${type.name} a does not have implement", exception)
} catch (exception: IllegalAccessException) {
throw UnsupportedOperationException("${type.name} constructor is not visible")
} catch (exception: InstantiationException) {
throw UnsupportedOperationException("${type.name} is abstract class")
} catch (exception: InvocationTargetException) {
throw UnsupportedOperationException(
"${type.name} has an error occurred while creating the instance",
exception
)
}
}
@Suppress("UNCHECKED_CAST")
fun loadNMS(type: Class, vararg initArgs: Any? = emptyArray()): T {
val packageName = type.`package`.name
val className = "NMS${type.simpleName}"
val parameterTypes = initArgs.map {
it?.javaClass
}.toTypedArray()
val candidates = ArrayList(2)
candidates.add("$packageName.$libraryVersion.$className")
val lastDot = packageName.lastIndexOf('.')
if (lastDot > 0) {
val superPackageName = packageName.substring(0, lastDot)
val subPackageName = packageName.substring(lastDot + 1)
candidates.add("$superPackageName.$libraryVersion.$subPackageName.$className")
}
return try {
val nmsClass = candidates.firstNotNullOfOrNull { candidate ->
try {
Class.forName(candidate, true, type.classLoader).asSubclass(type)
} catch (exception: ClassNotFoundException) {
null
}
} ?: throw ClassNotFoundException("Not found nms library class: $candidates")
val constructor = kotlin.runCatching {
nmsClass.getConstructor(*parameterTypes)
}.getOrNull() ?: throw UnsupportedOperationException("${type.name} does not have Constructor for [${parameterTypes.joinToString()}]")
constructor.newInstance(*initArgs) as T
} catch (exception: ClassNotFoundException) {
throw UnsupportedOperationException(
"${type.name} does not support this version: $libraryVersion",
exception
)
} catch (exception: IllegalAccessException) {
throw UnsupportedOperationException("${type.name} constructor is not visible")
} catch (exception: InstantiationException) {
throw UnsupportedOperationException("${type.name} is abstract class")
} catch (exception: InvocationTargetException) {
throw UnsupportedOperationException(
"${type.name} has an error occurred while creating the instance",
exception
)
}
}
val bukkitVersion by lazy {
with("v\\d+_\\d+_R\\d+".toPattern().matcher(Bukkit.getServer()::class.java.`package`.name)) {
when {
find() -> group()
else -> throw NoSuchElementException("No such bukkit version exists")
}
}
}
val minecraftVersion by lazy {
with("(?<=\\(MC: )[\\d.]+?(?=\\))".toPattern().matcher(Bukkit.getVersion())) {
when {
find() -> group()
else -> throw NoSuchElementException("No such minecraft version exists")
}
}
}
val libraryVersion by lazy { "v${minecraftVersion.replace('.', '_')}" }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy