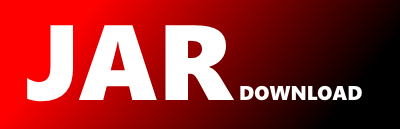
main.io.github.moonlightsuite.moonlight.SpatialTemporalMonitorDefinition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of moonlight-engine Show documentation
Show all versions of moonlight-engine Show documentation
MoonLight is a light-weight Java-tool for monitoring temporal, spatial and spatio-temporal properties of distributed complex systems, such as Cyber-Physical Systems and Collective Adaptive Systems.
The newest version!
package io.github.moonlightsuite.moonlight;
import io.github.moonlightsuite.moonlight.core.signal.SignalDomain;
import io.github.moonlightsuite.moonlight.offline.monitoring.spatialtemporal.SpatialTemporalMonitor;
import io.github.moonlightsuite.moonlight.core.base.MoonLightRecord;
import io.github.moonlightsuite.moonlight.offline.signal.RecordHandler;
import java.util.Arrays;
/**
* Instances of this class are used to represent a definition for a temporal monitor. Each definition
* has a name and a set of parameters that are used to build the temporal monitor.
*/
public class SpatialTemporalMonitorDefinition {
/**
* Monitor name.
*/
private final String name;
/**
* Monitor arguments.
*/
private final RecordHandler arguments;
/**
* Record handler describing monitored signal.
*/
private final RecordHandler signalRecordHandler;
/**
* Record handler describing monitored signal.
*/
private final RecordHandler edgeRecordHandler;
/**
* Producer used to build the temporal monitor.
*/
private final SpatialTemporalMonitorProducer producer;
/**
* Create a new definition.
*
* @param name monitor name.
* @param arguments monitor arguments.
* @param signalRecordHandler record handler describing monitored signal.
* @param producer producer used to build the monitor.
*/
public SpatialTemporalMonitorDefinition(String name, RecordHandler arguments, RecordHandler signalRecordHandler, RecordHandler edgeRecordHandler, SpatialTemporalMonitorProducer producer) {
this.name = name;
this.arguments = arguments;
this.signalRecordHandler = signalRecordHandler;
this.producer = producer;
this.edgeRecordHandler = edgeRecordHandler;
}
/**
* Return the monitor name.
* @return the monitor name.
*/
public String getName() {
return name;
}
/**
* Return the monitor arguments.
*
* @return the monitor arguments.
*/
public RecordHandler getArguments() {
return arguments;
}
/**
* Return the producer used to build the monitor.
*
* @return the producer used to build the monitor.
*/
public SpatialTemporalMonitorProducer getProducer() {
return producer;
}
/**
* Create a new temporal monitor built by using the given arguments and based on the given signal domain.
*
* @param domain signal domain used for the monitor.
* @param arguments argument used to build the monitor.
* @param data type used in the resulting signal.
* @return temporal monitor built by using the given arguments and based on the given signal domain.
*/
public SpatialTemporalMonitor getMonitor(SignalDomain domain, MoonLightRecord arguments) {
return producer.apply(domain,arguments);
}
public SpatialTemporalMonitor getMonitorFromString(SignalDomain domain, String[] values) {
return getMonitor(domain, arguments.fromStringArray(values));
}
public SpatialTemporalMonitor getMonitorFromDouble(SignalDomain domain, double[] values) {
return getMonitor(domain, evalArgumentFromDoubleArray(values));
}
private MoonLightRecord evalArgumentFromDoubleArray(double[] values) {
if (this.arguments == null) {
return null;
}
if (values == null) {
values = new double[0];
}
return this.arguments.fromDoubleArray(values);
}
public SpatialTemporalMonitor getMonitorFromObject(SignalDomain domain, Object[] values) {
return getMonitor(domain, arguments.fromObjectArray(values));
}
public RecordHandler getSignalRecordHandler() {
return signalRecordHandler;
}
public RecordHandler getEdgeRecordHandler() { return edgeRecordHandler; }
public String getInfo() {
return name+ Arrays.toString( arguments.getVariables() )+
"\n Signal: "+ Arrays.deepToString(signalRecordHandler.getVariables())+
"\n Edges: "+ Arrays.deepToString(edgeRecordHandler.getVariables());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy