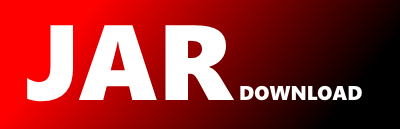
main.io.github.moonlightsuite.moonlight.domain.BoxDomain Maven / Gradle / Ivy
package io.github.moonlightsuite.moonlight.domain;
import io.github.moonlightsuite.moonlight.core.base.Box;
import io.github.moonlightsuite.moonlight.core.signal.SignalDomain;
import io.github.moonlightsuite.moonlight.core.io.DataHandler;
public class BoxDomain>
implements SignalDomain>
{
private final SignalDomain domain;
public BoxDomain(SignalDomain domain) {
this.domain = domain;
}
/**
* Unknown element: this is an element of the set that represents
* undefined areas of the signal.
* Examples of this could be 0 for real numbers,
* a third value for booleans, or the total interval for intervals.
*
* @return the element of the set representing absence of knowledge
*/
@Override
public Box any() {
return new Box<>(domain.min(), domain.max());
}
/**
* Negation function that s.t. De Morgan laws, double negation
* and inversion of the idempotent elements hold.
*
* @param x element to negate
* @return the negation of the x element
*/
@Override
public Box negation(Box x) {
return new Box<>(domain.negation(x.getEnd()),
domain.negation(x.getStart()));
}
/**
* Associative, commutative, idempotent operator that chooses a value.
*
* @param x first available value
* @param y second available value
* @return a result satisfying conjunction properties
*/
@Override
public Box conjunction(Box x, Box y) {
return new Box<>(
domain.conjunction(x.getStart(), y.getStart()),
domain.conjunction(x.getEnd(), y.getEnd()));
}
/**
* Associative, commutative operator that combines values.
*
* @param x first value to combine
* @param y second value to combine
* @return a result satisfying disjunction properties
*/
@Override
public Box disjunction(Box x, Box y) {
return new Box<>(
domain.disjunction(x.getStart(), y.getStart()),
domain.disjunction(x.getEnd(), y.getEnd()));
}
/**
* @return the infimum (aka meet) of the lattice defined over the semiring.
*/
@Override
public Box min() {
return new Box<>(domain.min(), domain.min());
}
/**
* @return the supremum (aka join) of the lattice defined over the semiring.
*/
@Override
public Box max() {
return new Box<>(domain.max(), domain.max());
}
/**
* @return an helper class to manage data parsing over the given type.
*/
@Override
public DataHandler> getDataHandler() {
return notImplemented();
}
@Override
public boolean equalTo(Box x, Box y) {
return x.equals(y);
}
@Override
public Box valueOf(boolean b) {
return notImplemented();
}
@Override
public Box valueOf(double v) {
return notImplemented();
}
@Override
public Box computeLessThan(double v1, double v2) {
return notImplemented();
}
@Override
public Box computeLessOrEqualThan(double v1, double v2) {
return notImplemented();
}
@Override
public Box computeEqualTo(double v1, double v2) {
return notImplemented();
}
@Override
public Box computeGreaterThan(double v1, double v2) {
return notImplemented();
}
@Override
public Box computeGreaterOrEqualThan(double v1, double v2) {
return notImplemented();
}
private T notImplemented() {
throw new UnsupportedOperationException("Operation not implemented.");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy