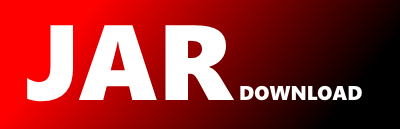
main.io.github.moonlightsuite.moonlight.offline.algorithms.ReduceOp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of moonlight-engine Show documentation
Show all versions of moonlight-engine Show documentation
MoonLight is a light-weight Java-tool for monitoring temporal, spatial and spatio-temporal properties of distributed complex systems, such as Cyber-Physical Systems and Collective Adaptive Systems.
The newest version!
/*
* MoonLight: a light-weight framework for runtime monitoring
* Copyright (C) 2018-2021
*
* See the NOTICE file distributed with this work for additional information
* regarding copyright ownership.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.moonlightsuite.moonlight.offline.algorithms;
import io.github.moonlightsuite.moonlight.core.space.DistanceStructure;
import io.github.moonlightsuite.moonlight.core.space.LocationService;
import io.github.moonlightsuite.moonlight.core.space.SpaceIterator;
import io.github.moonlightsuite.moonlight.core.space.SpatialModel;
import io.github.moonlightsuite.moonlight.offline.signal.ParallelSignalCursor1;
import io.github.moonlightsuite.moonlight.offline.signal.Signal;
import io.github.moonlightsuite.moonlight.offline.signal.mfr.MfrSignal;
import org.jetbrains.annotations.NotNull;
import java.util.Arrays;
import java.util.List;
import java.util.function.Function;
import java.util.function.IntFunction;
import java.util.stream.IntStream;
/**
* Algorithm for Reduce operator
*/
public class ReduceOp {
private final Function, R> aggregator;
private final LocationService locSvc;
private final Function, DistanceStructure> distance;
private final int size;
public ReduceOp(int size,
LocationService locSvc,
Function, DistanceStructure> distance,
Function, R> aggregator) {
this.size = size;
this.locSvc = locSvc;
this.distance = distance;
this.aggregator = aggregator;
}
public MfrSignal computeUnary(int[] definitionSet,
IntFunction> s) {
if (!locSvc.isEmpty()) {
return doCompute(s, definitionSet);
}
throw new UnsupportedOperationException("Invalid location service " +
"passed");
}
private MfrSignal doCompute(IntFunction> setSignal,
int[] definitionSet) {
return new MfrSignal<>(size, i -> reduce(setSignal.apply(i)),
definitionSet);
}
private Signal reduce(MfrSignal arg) {
var cursor = arg.getSignalCursor(true);
double t = cursor.getCurrentTime();
Signal result = new Signal<>();
var spaceItr = new SpaceIterator<>(locSvc, distance);
spaceItr.init(t);
while (!Double.isNaN(t) && isNotCompleted(cursor)) {
t = aggregateArg(arg, cursor, t, result, spaceItr);
}
return result;
}
private double aggregateArg(MfrSignal arg,
ParallelSignalCursor1 cursor,
double t,
Signal result,
SpaceIterator spaceItr) {
var spatialSignal = cursor.getCurrentValue();
aggregate(t, arg, result, spatialSignal);
double tNext = cursor.forwardTime();
spaceItr.forEach(tNext, (itT, itDs) ->
aggregate(itT, arg, result, spatialSignal)
);
t = moveSpatialModel(tNext, spaceItr);
return t;
}
private void aggregate(Double t, MfrSignal arg,
Signal result, IntFunction spatialSignal) {
var values = locationStream(arg).mapToObj(spatialSignal).toList();
R aggregated = aggregator.apply(values);
result.add(t, aggregated);
}
private IntStream locationStream(MfrSignal signal) {
return Arrays.stream(signal.getLocationsSet());
}
private Double moveSpatialModel(@NotNull Double t, SpaceIterator spaceItr) {
if (spaceItr.isNextSpaceModelAtSameTime(t)) {
spaceItr.shiftSpatialModel();
}
return t;
}
@SafeVarargs
private static boolean isNotCompleted(ParallelSignalCursor1... cursors) {
return Arrays.stream(cursors)
.map(c -> !c.isCompleted())
.reduce(true, (c1, c2) -> c1 && c2);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy