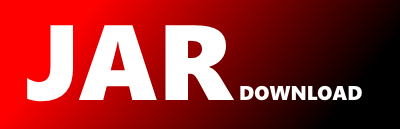
main.io.github.moonlightsuite.moonlight.offline.signal.mfr.MfrSignal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of moonlight-engine Show documentation
Show all versions of moonlight-engine Show documentation
MoonLight is a light-weight Java-tool for monitoring temporal, spatial and spatio-temporal properties of distributed complex systems, such as Cyber-Physical Systems and Collective Adaptive Systems.
The newest version!
package io.github.moonlightsuite.moonlight.offline.signal.mfr;
import io.github.moonlightsuite.moonlight.offline.algorithms.BooleanOp;
import io.github.moonlightsuite.moonlight.offline.signal.ParallelSignalCursor1;
import io.github.moonlightsuite.moonlight.offline.signal.STSignal;
import io.github.moonlightsuite.moonlight.offline.signal.Signal;
import io.github.moonlightsuite.moonlight.offline.signal.SignalCursor;
import java.util.Arrays;
import java.util.function.*;
import java.util.stream.IntStream;
public class MfrSignal extends STSignal {
private final int[] locations;
public MfrSignal(int size, IntFunction> generator) {
super(size, generator);
this.locations = IntStream.range(1, size).toArray();
}
public MfrSignal(int size, IntFunction> generator,
int[] locations) {
super(size, generator);
this.locations = locations;
}
private static boolean contains(int target, int[] elements) {
for (int element : elements) {
if (element == target) {
return true;
}
}
return false;
}
public T getValueAt(int location, double time) {
return getSignalAtLocation(location).getValueAt(time);
}
@Override
public Signal getSignalAtLocation(int location) {
var position = hasLocation(location);
if (position >= 0) {
return getSignals().get(position);
}
var error = "The signal is not defined at the given location";
throw new IllegalArgumentException(error);
}
private int hasLocation(int location) {
return Arrays.binarySearch(locations, location);
}
public int[] getLocationsSet() {
return locations;
}
@Override
public MfrSignal apply(Function f) {
return new MfrSignal<>(getNumberOfLocations(),
i -> partialFunction(i, j -> mappedSignal(j, f)), locations);
}
private A partialFunction(int location, IntFunction partialValue) {
if (hasLocation(location) >= 0) {
return partialValue.apply(location);
} else {
var error = "Signal undefined at location " + location + "!";
//throw new IllegalArgumentException(error);
// TODO: we should handle this in some way
return null;
}
}
private Signal mappedSignal(int l, Function f) {
BooleanOp booleanOp = new BooleanOp<>();
return booleanOp.applyUnary(getSignalAtLocation(l), f);
}
public MfrSignal filter(Predicate p) {
return new MfrSignal<>(getNumberOfLocations(),
i -> partialFunction(i, j -> filteredSignal(i, p)), locations);
}
private Signal filteredSignal(int l, Predicate p) {
BooleanOp booleanOp = new BooleanOp<>();
return booleanOp.filterUnary(getSignalAtLocation(l), p);
}
@Override
public ParallelSignalCursor1 getSignalCursor(boolean forward) {
IntFunction> cursor =
l -> getSignalAtLocation(l).getIterator(forward);
return new MfrCursor<>(locations, cursor);
}
public MfrSignal combine(
BinaryOperator f,
STSignal s,
int[] locations) {
checkSize(s.getNumberOfLocations());
return new MfrSignal<>(getNumberOfLocations(),
i -> binaryOpSignal(i, f, this, s),
locations);
}
private static Signal binaryOpSignal(int i,
BiFunction f,
STSignal s1,
STSignal s2) {
BooleanOp booleanOp = new BooleanOp<>();
return booleanOp.applyBinary(s1.getSignalAtLocation(i), f,
s2.getSignalAtLocation(i));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy