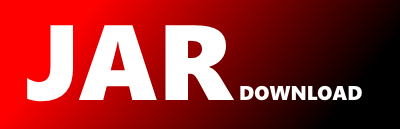
main.io.github.moonlightsuite.moonlight.util.Stopwatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of moonlight-engine Show documentation
Show all versions of moonlight-engine Show documentation
MoonLight is a light-weight Java-tool for monitoring temporal, spatial and spatio-temporal properties of distributed complex systems, such as Cyber-Physical Systems and Collective Adaptive Systems.
The newest version!
package io.github.moonlightsuite.moonlight.util;
import io.github.moonlightsuite.moonlight.core.base.Pair;
import java.util.HashMap;
import java.util.Map;
import java.util.UUID;
/**
* EXPERIMENTAL: Utility class for tracking execution time.
*/
public class Stopwatch {
private static final Map> sessions = new HashMap<>();
private final UUID sessionId;
private Stopwatch(UUID id) {
sessionId = id;
}
public static Stopwatch start() {
UUID id = UUID.randomUUID();
Long start = System.currentTimeMillis();
sessions.put(id, new Pair<>(start, null));
return new Stopwatch(id);
}
public long stop() {
Long start = sessions.remove(sessionId).getFirst();
Long end = System.currentTimeMillis();
sessions.put(sessionId, new Pair<>(start, end));
return end - start;
}
public static long getDuration(UUID sessionId) {
Pair session = sessions.get(sessionId);
if(session != null && session.getSecond() != null) {
return session.getSecond() - session.getFirst();
} else
throw new UnsupportedOperationException("The requested session " +
"never started or never " +
"ended");
}
public long getDuration() {
Pair session = sessions.get(sessionId);
if(session != null && session.getSecond() != null) {
return session.getSecond() - session.getFirst();
} else
throw new UnsupportedOperationException("The requested session " +
"never ended");
}
public static Map> getSessions() {
return new HashMap<>(sessions);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy