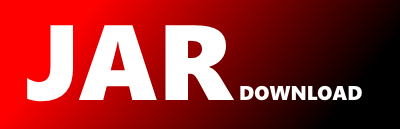
fr.profi.mzdb.util.iterator.BufferedIterator Maven / Gradle / Ivy
package fr.profi.mzdb.util.iterator;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.NoSuchElementException;
public class BufferedIterator implements Iterator {
private Iterator source;
private int max;
private LinkedList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy