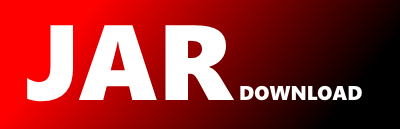
fr.profi.mzdb.util.threading.Executor Maven / Gradle / Ivy
/**
* Class launching several threads with runnable or callables
* size of runnable array can be greater than the number of cores
*/
package fr.profi.mzdb.util.threading;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
/**
*
* @author marco
*
*/
public class Executor {
private ExecutorService pool;
private Callable
© 2015 - 2024 Weber Informatics LLC | Privacy Policy