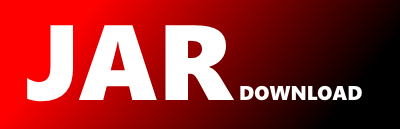
io.github.msdk.rawdata.peakinvestigator.PeakInvestigatorMsSpectrum Maven / Gradle / Ivy
/*
* (C) Copyright 2015-2017 by MSDK Development Team
*
* This software is dual-licensed under either
*
* (a) the terms of the GNU Lesser General Public License version 2.1 as published by the Free
* Software Foundation
*
* or (per the licensee's choosing)
*
* (b) the terms of the Eclipse Public License v1.0 as published by the Eclipse Foundation.
*/
package io.github.msdk.rawdata.peakinvestigator;
import java.util.TreeMap;
import com.google.common.collect.Range;
import io.github.msdk.datamodel.msspectra.MsSpectrum;
import io.github.msdk.datamodel.msspectra.MsSpectrumType;
import io.github.msdk.util.tolerances.MzTolerance;
/**
* This class is used provide m/z-dependent mass tolerances from PeakInvestigator by decorating an
* existing instance of MsSpectrum.
*
* @author plusik
* @version $Id: $Id
*/
public class PeakInvestigatorMsSpectrum implements MsSpectrum {
private final MsSpectrum spectrum;
private final TreeMap errors;
private double multiplier = 1.0;
/**
*
* Constructor for PeakInvestigatorMsSpectrum.
*
*
* @param spectrum a {@link io.github.msdk.datamodel.msspectra.MsSpectrum} object.
* @param errors a {@link java.util.TreeMap} object.
*/
public PeakInvestigatorMsSpectrum(MsSpectrum spectrum, TreeMap errors) {
this.spectrum = spectrum;
this.errors = errors;
}
/**
*
* Getter for the field multiplier
.
*
*
* @return a double.
*/
public double getMultiplier() {
return this.multiplier;
}
/**
*
* Setter for the field multiplier
.
*
*
* @param multiplier a double.
*/
public void setMultiplier(double multiplier) {
this.multiplier = multiplier;
}
/** {@inheritDoc} */
@Override
public MsSpectrumType getSpectrumType() {
return MsSpectrumType.CENTROIDED;
}
/** {@inheritDoc} */
@Override
public Integer getNumberOfDataPoints() {
return spectrum.getNumberOfDataPoints();
}
/** {@inheritDoc} */
@Override
public double[] getMzValues() {
return spectrum.getMzValues();
}
/** {@inheritDoc} */
@Override
public float[] getIntensityValues() {
return spectrum.getIntensityValues();
}
/** {@inheritDoc} */
@Override
public Float getTIC() {
return spectrum.getTIC();
}
/** {@inheritDoc} */
@Override
public Range getMzRange() {
return spectrum.getMzRange();
}
/**
*
* getToleranceRange.
*
*
* @param mzValue a {@link java.lang.Double} object.
* @return a {@link com.google.common.collect.Range} object.
*/
public Range getToleranceRange(Double mzValue) {
Error error = errors.get(mzValue);
double mzError = multiplier * error.MZ_ERROR;
if (error.MIN_ERROR > mzError) {
mzError = error.MIN_ERROR;
}
return Range.closed(mzValue - mzError, mzValue + mzError);
}
/**
*
* getError.
*
*
* @param mzValue a {@link java.lang.Double} object.
* @return a {@link io.github.msdk.rawdata.peakinvestigator.PeakInvestigatorMsSpectrum.Error}
* object.
*/
public Error getError(Double mzValue) {
return errors.get(mzValue);
}
public static class Error {
public final double MZ_ERROR;
public final float INTENSITY_ERROR;
public final double MIN_ERROR;
public Error(double mzError, float intensityError, double minError) {
MZ_ERROR = mzError;
INTENSITY_ERROR = intensityError;
MIN_ERROR = minError;
}
}
/** {@inheritDoc} */
@Override
public MzTolerance getMzTolerance() {
// TODO Auto-generated method stub
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy