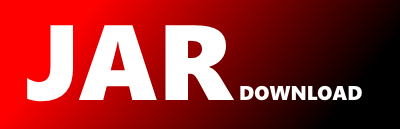
mmb.engine.CatchingEvent Maven / Gradle / Ivy
/**
*
*/
package mmb.engine;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.concurrent.CopyOnWriteArrayList;
import java.util.function.Consumer;
import com.pploder.events.Event;
import mmb.engine.debug.Debugger;
/**
* An implementation of an event, which catches and reports exceptions thrown by listeners
* @author oskar (catch exceptions, nullability)
* @author Philipp Ploder (original implementation)
* @param The argument type to be passed to the listeners. (copied from {@link Event})
* @since MMB 0.5-pre5
*/
@SuppressWarnings({"null"})
public class CatchingEvent implements Event {
private Debugger debug;
private String msg;
/**
* Creates a catching event
* @param debug debugger which prints errors
* @param msg error message
*/
public CatchingEvent(Debugger debug, String msg) {
this.debug = debug;
this.msg = msg;
}
private final List> listeners = new CopyOnWriteArrayList<>();
@Override
public void addListener(Consumer listener) throws NullPointerException {
synchronized (listeners) {
listeners.add(listener);
}
}
@Override
public void addAllListeners(Consumer... listeners) throws NullPointerException {
addAllListeners(Arrays.asList(listeners));
}
@Override
public void addAllListeners(Collection> listeners) throws NullPointerException {
if (!listeners.isEmpty()) {
if (listeners.stream().anyMatch(Objects::isNull)) {
throw new NullPointerException("At least one of the given listeners is a null-reference");
}
synchronized (this.listeners) {
this.listeners.addAll(listeners);
}
}
}
@Override
public void removeListener(Consumer listener) throws NullPointerException {
synchronized (listeners) {
listeners.remove(listener);
}
}
@Override
public void removeAllOccurrences(Consumer listener) throws NullPointerException {
synchronized (listeners) {
listeners.removeIf(listener::equals);
}
}
@Override
public void trigger(T t) {
listeners.forEach(listener -> {
try {
listener.accept(t);
}catch(Exception e) {
debug.stacktraceError(e, msg);
}
});
}
public void clear() {
listeners.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy