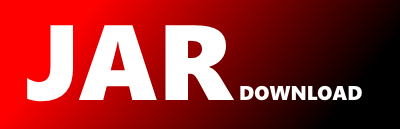
mmb.engine.mbmachine.Machine Maven / Gradle / Ivy
/**
*
*/
package mmb.engine.mbmachine;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.Rectangle;
import mmb.beans.Saver;
import mmb.engine.worlds.MapProxy;
import mmb.engine.worlds.world.World;
/**
* @author oskar
* A machine is an entity that is run in real time, in contrast to blocks that require update events and/or triggers.
*
* The following beans are supported by default:
*
* - ActionListener - machine activation
* - InventoryContainer - inventory
* - BlockInterface - connect to other machines
* - SignalListener - detect control signals
*
*
* 0.5 planned:
*
*
* 0.6 planned:
*
* - Vehicle - able to move on roads and tracks
* - InventoryContainer - inventory
* - BlockInterface - connect to other machines
*
*/
public interface Machine extends Saver{
//[start] positioning
public default void setPos(Point p) {
setPos(p.x, p.y);
}
/**
* Set machine's position
* @param x X coordinate
* @param y Y coordinate
*/
public void setPos(int x, int y);
/**
* Set machine's X position
* @param x X coordinate
*/
public void setX(int x);
/**
* Set machine's Y position
* @param y Y coordinate
*/
public void setY(int y);
/**
* @return X coordinate
*/
public int posX();
/**
* @return Y coordinate
*/
public int posY();
/**
* Returns the location of this {@code Machine} in a new {@link Point}
* @return location of this {@code Machine}
*/
public default Point pos() {
return new Point(posX(), posY());
}
public int sizeX();
public int sizeY();
public String id();
/**
* Returns the size of this {@code Machine} in a new {@link Dimension}
* @return size of this {@code Machine}
*/
public default Dimension size() {
return new Dimension(sizeX(), sizeY());
}
/**
* Returns the size of this {@code Machine} in a new {@link Rectangle}
* @return size of this {@code Machine}
*/
public default Rectangle bounds() {
return new Rectangle(posX(), posY(), sizeX(), sizeY());
}
//[end]
//[start] world
public World getMap();
public void setMap(World map);
//[end]
//[start] runtime
/**
* Handles start-up.
*
Does not run when block is placed.
*
To handle placement, set onPlace variable
*
*
Event: world loading
*
Purpose: initialize block
*
Exception handling: If exception occurs, a block is not properly initialized and error is logged into:
*
* - log file, which remains on a server
* - error statement, sent to registered server users and newsletter subscribers
*
*/
public void onStartup();
/**
* This function is run when the data is provided to the block.
*
To handle placement, set onPlace variable
*
*
Event: world loading
*
Purpose: initialize block data
*
Exception handling: If exception occurs, a block is not properly initialized and error is logged into:
*
* - log file, which remains on a server
* - error statement, sent to registered server users and newsletter subscribers
*
*/
public void onDataLoaded();
/**
* Run an update on this machine for every tick
*
NOTE: design this function carefully to prevent logspam
*
*
Event: tick update (50 times per second)
*
Purpose: run normal updates
*
Exception handling: If exception occurs, block is not properly updated and error is logged and chatted
*
* - to a player, if action was requested by a player or theirs block,
* - or public, if action was requested by unclaimed or server owned block
*
* @param proxy the map's proxy
*/
public void onUpdate(MapProxy proxy);
/**
* Handles the machine being placed.
*
* - Event: block placement
* - Purpose: correctly place blocks
* -
* Exception handling: If exception occurs, block is not placed and error is logged and chatted
*
* - to a player, if action was requested by a player or theirs block,
* - or public, if action was requested by unclaimed or server owned block
*
*
*
*/
public void onPlace();
/**
* Handles the machine being mined.
*
* - Event: block mining
* - Purpose: correctly mine blocks
* -
* Exception handling: If exception occurs, block is not removed and error is logged and chatted
*
* - to a player, if action was requested by a player or theirs block,
* - or public, if action was requested by unclaimed or server owned block
*
*
*
*/
public void onRemove();
/**
* Prepares a block for server shutdown.
*
Does not run when block is broken.
*
To handle block removal,set onRemove variable
*
*
Event: server shutdown
*
Purpose: finalize block before disposal
*
Exception handling: If exception occurs, block is not properly finalized and error is logged into:
*
* - log file, which remains on a server
* - error statement, sent to registered server users and newsletter subscribers
*
*/
public void onShutdown();
//[end]
/**
* Render a machine
*
* The graphics context is isolated from main graphics context
* @param g graphics context
*/
public void render(Graphics g);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy