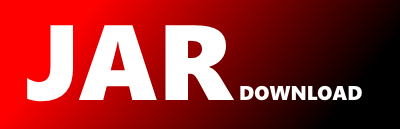
monniasza.collects.selfset.BaseMapSelfSet Maven / Gradle / Ivy
/**
*
*/
package monniasza.collects.selfset;
import java.util.Collection;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.function.Function;
import mmb.NN;
import mmb.Nil;
/**
* An basic map-based self-set
* @author oskar
* @param
* @param
* @param the type of map
*/
public class BaseMapSelfSet> implements SelfSet{
@NN private final M map;
private final boolean nullable;
@Nil private final Class type;
@NN private final Function fn;
/**
* A complex self-set constructor. Usually not used by the user
* @param map
* @param nullable is the self-set nullable
* @param type
* @param fn
*/
public BaseMapSelfSet(@NN M map, boolean nullable, Class type, Function fn) {
this.map = map;
this.nullable = nullable;
this.type = type;
this.fn = fn;
}
@Override
public int size() {
return map.size();
}
@Override
public boolean isEmpty() {
return map.isEmpty();
}
@Override
public @NN Iterator iterator() {
return map.values().iterator();
}
@Override
public Object @NN [] toArray() {
return map.values().toArray();
}
@Override
public T @NN [] toArray(T[] a) {
return map.values().toArray(a);
}
@Override
public boolean add(V e) {
return map.put(id(e), e) == null;
}
@Override
public boolean containsAll(Collection> c) {
for(Object obj: c) {
if(!test(obj)) return false;
Object id = id(obj);
if(!map.containsKey(id)) return false;
}
return true;
}
@Override
public boolean addAll(Collection extends V> c) {
boolean result = false;
for(V obj: c) {
result |= add(obj);
}
return result;
}
@Override
public boolean retainAll(Collection> c) {
AtomicBoolean changed = new AtomicBoolean();
map.entrySet().removeIf(e ->{
if(!c.contains(e.getValue())) return false;
changed.set(true);
return true;
});
return changed.get();
}
@Override
public boolean removeAll(Collection> c) {
boolean removed = false;
for(Object obj: c) {
removed |= map.remove(obj) != null;
}
return removed;
}
@Override
public void clear() {
map.clear();
}
@Override
public Set keys() {
return map.keySet();
}
@Override
public Set values() {
return this;
}
@Override
public V get(@Nil Object key) {
return map.get(key);
}
@Override
public V getOrDefault(@Nil Object key, V defalt) {
return map.getOrDefault(key, defalt);
}
@Override
public boolean removeKey(K key) {
return map.remove(key) != null;
}
@Override
public boolean containsKey(@Nil Object key) {
return map.containsKey(key);
}
@Override
public boolean test(@Nil Object o) {
if(o == null) return nullable;
Class cls = type;
if(cls == null) return true;
return cls.isInstance(o);
}
@SuppressWarnings("unchecked")
@Override
public K id(@Nil Object value) {
return fn.apply((V) value);
}
@Override
public boolean nullable() {
return nullable;
}
@Override
public Class type() {
return type;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy