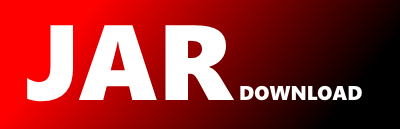
org.apache.iceberg.transforms.Transform Maven / Gradle / Ivy
Show all versions of iceberg-api Show documentation
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.iceberg.transforms;
import java.io.Serializable;
import org.apache.iceberg.expressions.BoundPredicate;
import org.apache.iceberg.expressions.UnboundPredicate;
import org.apache.iceberg.types.Type;
/**
* A transform function used for partitioning.
*
* Implementations of this interface can be used to transform values, check or types, and project
* {@link BoundPredicate predicates} to predicates on partition values.
*
* @param Java class of source values
* @param Java class of transformed values
*/
public interface Transform extends Serializable {
/**
* Transforms a value to its corresponding partition value.
*
* @param value a source value
* @return a transformed partition value
*/
T apply(S value);
/**
* Checks whether this function can be applied to the given {@link Type}.
*
* @param type a type
* @return true if this transform can be applied to the type, false otherwise
*/
boolean canTransform(Type type);
/**
* Returns the {@link Type} produced by this transform given a source type.
*
* @param sourceType a type
* @return the result type created by the apply method for the given type
*/
Type getResultType(Type sourceType);
/**
* Whether the transform preserves the order of values (is monotonic).
*
* A transform preserves order for values when for any given a and b, if a < b then apply(a) <= apply(b).
*
* @return true if the transform preserves the order of values
*/
default boolean preservesOrder() {
return false;
}
/**
* Whether ordering by this transform's result satisfies the ordering of another transform's result.
*
* For example, sorting by day(ts) will produce an ordering that is also by month(ts) or year(ts). However, sorting
* by day(ts) will not satisfy the order of hour(ts) or identity(ts).
*
* @return true if ordering by this transform is equivalent to ordering by the other transform
*/
default boolean satisfiesOrderOf(Transform, ?> other) {
return equals(other);
}
/**
* Transforms a {@link BoundPredicate predicate} to an inclusive predicate on the partition
* values produced by {@link #apply(Object)}.
*
* This inclusive transform guarantees that if pred(v) is true, then projected(apply(v)) is true.
*
* @param name the field name for partition values
* @param predicate a predicate for source values
* @return an inclusive predicate on partition values
*/
UnboundPredicate project(String name, BoundPredicate predicate);
/**
* Transforms a {@link BoundPredicate predicate} to a strict predicate on the partition values
* produced by {@link #apply(Object)}.
*
* This strict transform guarantees that if strict(apply(v)) is true, then pred(v) is also true.
*
* @param name the field name for partition values
* @param predicate a predicate for source values
* @return an inclusive predicate on partition values
*/
UnboundPredicate projectStrict(String name, BoundPredicate predicate);
/**
* Return whether this transform is the identity transform.
*
* @return true if this is an identity transform, false otherwise
*/
default boolean isIdentity() {
return false;
}
/**
* Returns a human-readable String representation of a transformed value.
*
* null values will return "null"
*
* @param value a transformed value
* @return a human-readable String representation of the value
*/
default String toHumanString(T value) {
return String.valueOf(value);
}
/**
* Return the unique transform name to check if similar transforms for the same source field
* are added multiple times in partition spec builder.
*
* @return a name used for dedup
*/
default String dedupName() {
return toString();
}
}