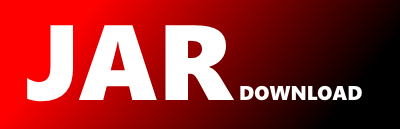
io.github.navi_cloud.shared.authentication.AuthenticationGrpc Maven / Gradle / Ivy
package io.github.navi_cloud.shared.authentication;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* Service Description
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.40.1)",
comments = "Source: AuthenticationService/UserService.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class AuthenticationGrpc {
private AuthenticationGrpc() {}
public static final String SERVICE_NAME = "io.github.navi_cloud.shared.authentication.Authentication";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getRegisterUserMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "RegisterUser",
requestType = io.github.navi_cloud.shared.authentication.CommunicationMessage.RegisterRequest.class,
responseType = io.github.navi_cloud.shared.CommonCommunication.Result.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRegisterUserMethod() {
io.grpc.MethodDescriptor getRegisterUserMethod;
if ((getRegisterUserMethod = AuthenticationGrpc.getRegisterUserMethod) == null) {
synchronized (AuthenticationGrpc.class) {
if ((getRegisterUserMethod = AuthenticationGrpc.getRegisterUserMethod) == null) {
AuthenticationGrpc.getRegisterUserMethod = getRegisterUserMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "RegisterUser"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.github.navi_cloud.shared.authentication.CommunicationMessage.RegisterRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.github.navi_cloud.shared.CommonCommunication.Result.getDefaultInstance()))
.setSchemaDescriptor(new AuthenticationMethodDescriptorSupplier("RegisterUser"))
.build();
}
}
}
return getRegisterUserMethod;
}
private static volatile io.grpc.MethodDescriptor getLoginUserMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "LoginUser",
requestType = io.github.navi_cloud.shared.authentication.CommunicationMessage.LoginRequest.class,
responseType = io.github.navi_cloud.shared.CommonCommunication.Result.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getLoginUserMethod() {
io.grpc.MethodDescriptor getLoginUserMethod;
if ((getLoginUserMethod = AuthenticationGrpc.getLoginUserMethod) == null) {
synchronized (AuthenticationGrpc.class) {
if ((getLoginUserMethod = AuthenticationGrpc.getLoginUserMethod) == null) {
AuthenticationGrpc.getLoginUserMethod = getLoginUserMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "LoginUser"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.github.navi_cloud.shared.authentication.CommunicationMessage.LoginRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.github.navi_cloud.shared.CommonCommunication.Result.getDefaultInstance()))
.setSchemaDescriptor(new AuthenticationMethodDescriptorSupplier("LoginUser"))
.build();
}
}
}
return getLoginUserMethod;
}
private static volatile io.grpc.MethodDescriptor getAuthenticateUserMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "AuthenticateUser",
requestType = io.github.navi_cloud.shared.authentication.CommunicationMessage.AuthenticationRequest.class,
responseType = io.github.navi_cloud.shared.CommonCommunication.Result.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getAuthenticateUserMethod() {
io.grpc.MethodDescriptor getAuthenticateUserMethod;
if ((getAuthenticateUserMethod = AuthenticationGrpc.getAuthenticateUserMethod) == null) {
synchronized (AuthenticationGrpc.class) {
if ((getAuthenticateUserMethod = AuthenticationGrpc.getAuthenticateUserMethod) == null) {
AuthenticationGrpc.getAuthenticateUserMethod = getAuthenticateUserMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "AuthenticateUser"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.github.navi_cloud.shared.authentication.CommunicationMessage.AuthenticationRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.github.navi_cloud.shared.CommonCommunication.Result.getDefaultInstance()))
.setSchemaDescriptor(new AuthenticationMethodDescriptorSupplier("AuthenticateUser"))
.build();
}
}
}
return getAuthenticateUserMethod;
}
private static volatile io.grpc.MethodDescriptor getRemoveUserMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "RemoveUser",
requestType = io.github.navi_cloud.shared.authentication.CommunicationMessage.AccountRemovalRequest.class,
responseType = io.github.navi_cloud.shared.CommonCommunication.Result.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRemoveUserMethod() {
io.grpc.MethodDescriptor getRemoveUserMethod;
if ((getRemoveUserMethod = AuthenticationGrpc.getRemoveUserMethod) == null) {
synchronized (AuthenticationGrpc.class) {
if ((getRemoveUserMethod = AuthenticationGrpc.getRemoveUserMethod) == null) {
AuthenticationGrpc.getRemoveUserMethod = getRemoveUserMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "RemoveUser"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.github.navi_cloud.shared.authentication.CommunicationMessage.AccountRemovalRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.github.navi_cloud.shared.CommonCommunication.Result.getDefaultInstance()))
.setSchemaDescriptor(new AuthenticationMethodDescriptorSupplier("RemoveUser"))
.build();
}
}
}
return getRemoveUserMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static AuthenticationStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public AuthenticationStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new AuthenticationStub(channel, callOptions);
}
};
return AuthenticationStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static AuthenticationBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public AuthenticationBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new AuthenticationBlockingStub(channel, callOptions);
}
};
return AuthenticationBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static AuthenticationFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public AuthenticationFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new AuthenticationFutureStub(channel, callOptions);
}
};
return AuthenticationFutureStub.newStub(factory, channel);
}
/**
*
* Service Description
*
*/
public static abstract class AuthenticationImplBase implements io.grpc.BindableService {
/**
*/
public void registerUser(io.github.navi_cloud.shared.authentication.CommunicationMessage.RegisterRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getRegisterUserMethod(), responseObserver);
}
/**
*/
public void loginUser(io.github.navi_cloud.shared.authentication.CommunicationMessage.LoginRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getLoginUserMethod(), responseObserver);
}
/**
*/
public void authenticateUser(io.github.navi_cloud.shared.authentication.CommunicationMessage.AuthenticationRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getAuthenticateUserMethod(), responseObserver);
}
/**
*/
public void removeUser(io.github.navi_cloud.shared.authentication.CommunicationMessage.AccountRemovalRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getRemoveUserMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getRegisterUserMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
io.github.navi_cloud.shared.authentication.CommunicationMessage.RegisterRequest,
io.github.navi_cloud.shared.CommonCommunication.Result>(
this, METHODID_REGISTER_USER)))
.addMethod(
getLoginUserMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
io.github.navi_cloud.shared.authentication.CommunicationMessage.LoginRequest,
io.github.navi_cloud.shared.CommonCommunication.Result>(
this, METHODID_LOGIN_USER)))
.addMethod(
getAuthenticateUserMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
io.github.navi_cloud.shared.authentication.CommunicationMessage.AuthenticationRequest,
io.github.navi_cloud.shared.CommonCommunication.Result>(
this, METHODID_AUTHENTICATE_USER)))
.addMethod(
getRemoveUserMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
io.github.navi_cloud.shared.authentication.CommunicationMessage.AccountRemovalRequest,
io.github.navi_cloud.shared.CommonCommunication.Result>(
this, METHODID_REMOVE_USER)))
.build();
}
}
/**
*
* Service Description
*
*/
public static final class AuthenticationStub extends io.grpc.stub.AbstractAsyncStub {
private AuthenticationStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected AuthenticationStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new AuthenticationStub(channel, callOptions);
}
/**
*/
public void registerUser(io.github.navi_cloud.shared.authentication.CommunicationMessage.RegisterRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getRegisterUserMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void loginUser(io.github.navi_cloud.shared.authentication.CommunicationMessage.LoginRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getLoginUserMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void authenticateUser(io.github.navi_cloud.shared.authentication.CommunicationMessage.AuthenticationRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getAuthenticateUserMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void removeUser(io.github.navi_cloud.shared.authentication.CommunicationMessage.AccountRemovalRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getRemoveUserMethod(), getCallOptions()), request, responseObserver);
}
}
/**
*
* Service Description
*
*/
public static final class AuthenticationBlockingStub extends io.grpc.stub.AbstractBlockingStub {
private AuthenticationBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected AuthenticationBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new AuthenticationBlockingStub(channel, callOptions);
}
/**
*/
public io.github.navi_cloud.shared.CommonCommunication.Result registerUser(io.github.navi_cloud.shared.authentication.CommunicationMessage.RegisterRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getRegisterUserMethod(), getCallOptions(), request);
}
/**
*/
public io.github.navi_cloud.shared.CommonCommunication.Result loginUser(io.github.navi_cloud.shared.authentication.CommunicationMessage.LoginRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getLoginUserMethod(), getCallOptions(), request);
}
/**
*/
public io.github.navi_cloud.shared.CommonCommunication.Result authenticateUser(io.github.navi_cloud.shared.authentication.CommunicationMessage.AuthenticationRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getAuthenticateUserMethod(), getCallOptions(), request);
}
/**
*/
public io.github.navi_cloud.shared.CommonCommunication.Result removeUser(io.github.navi_cloud.shared.authentication.CommunicationMessage.AccountRemovalRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getRemoveUserMethod(), getCallOptions(), request);
}
}
/**
*
* Service Description
*
*/
public static final class AuthenticationFutureStub extends io.grpc.stub.AbstractFutureStub {
private AuthenticationFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected AuthenticationFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new AuthenticationFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture registerUser(
io.github.navi_cloud.shared.authentication.CommunicationMessage.RegisterRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getRegisterUserMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture loginUser(
io.github.navi_cloud.shared.authentication.CommunicationMessage.LoginRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getLoginUserMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture authenticateUser(
io.github.navi_cloud.shared.authentication.CommunicationMessage.AuthenticationRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getAuthenticateUserMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture removeUser(
io.github.navi_cloud.shared.authentication.CommunicationMessage.AccountRemovalRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getRemoveUserMethod(), getCallOptions()), request);
}
}
private static final int METHODID_REGISTER_USER = 0;
private static final int METHODID_LOGIN_USER = 1;
private static final int METHODID_AUTHENTICATE_USER = 2;
private static final int METHODID_REMOVE_USER = 3;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AuthenticationImplBase serviceImpl;
private final int methodId;
MethodHandlers(AuthenticationImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_REGISTER_USER:
serviceImpl.registerUser((io.github.navi_cloud.shared.authentication.CommunicationMessage.RegisterRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LOGIN_USER:
serviceImpl.loginUser((io.github.navi_cloud.shared.authentication.CommunicationMessage.LoginRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_AUTHENTICATE_USER:
serviceImpl.authenticateUser((io.github.navi_cloud.shared.authentication.CommunicationMessage.AuthenticationRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_REMOVE_USER:
serviceImpl.removeUser((io.github.navi_cloud.shared.authentication.CommunicationMessage.AccountRemovalRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static abstract class AuthenticationBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
AuthenticationBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return io.github.navi_cloud.shared.authentication.UserService.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("Authentication");
}
}
private static final class AuthenticationFileDescriptorSupplier
extends AuthenticationBaseDescriptorSupplier {
AuthenticationFileDescriptorSupplier() {}
}
private static final class AuthenticationMethodDescriptorSupplier
extends AuthenticationBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
AuthenticationMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (AuthenticationGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new AuthenticationFileDescriptorSupplier())
.addMethod(getRegisterUserMethod())
.addMethod(getLoginUserMethod())
.addMethod(getAuthenticateUserMethod())
.addMethod(getRemoveUserMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy