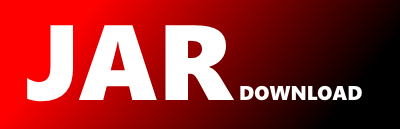
zserio.extension.doc.DocClassicToHtmlConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zserio Show documentation
Show all versions of zserio Show documentation
Zserio Serialization Framework Compiler
The newest version!
package zserio.extension.doc;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Set;
/**
* Converter for the classic documentation comment text into HTML format.
*
* The classic documentation comment text can contain HTML tags. These tags must be identified and passed
* through without escaping.
*/
final class DocClassicToHtmlConverter
{
public static String convert(String text)
{
final StringBuilder output = new StringBuilder();
for (int i = 0; i < text.length(); i++)
{
final String htmlTag = findHtmlTag(text, i);
if (htmlTag != null)
{
// this is HTML tag, skip escaping
output.append(htmlTag);
i += htmlTag.length() - 1;
}
else
{
// this is normal character to escape
output.append(escapeForHtml(text.charAt(i)));
}
}
return output.toString();
}
private static String escapeForHtml(char character)
{
switch (character)
{
case '<':
return "<";
case '>':
return ">";
case '&':
return "&";
case '"':
return """;
default:
return Character.toString(character);
}
}
private static String findHtmlTag(String text, int startIndex)
{
if (text.charAt(startIndex) != '<')
return null;
StringBuilder foundTagBuilder = new StringBuilder("<");
String foundTag = null;
boolean foundStartWithParams = false;
for (int i = startIndex + 1; i < text.length(); ++i)
{
final char character = text.charAt(i);
if (!foundStartWithParams && character == ' ')
{
// start tag with parameters
if (!startHtmlTagList.contains(foundTagBuilder.toString() + ">"))
break;
foundTagBuilder.append(character);
foundStartWithParams = true;
}
else
{
foundTagBuilder.append(character);
if (character == '>')
{
if (foundStartWithParams)
{
// start tag with parameters
foundTag = foundTagBuilder.toString();
}
else
{
// start tag without parameters or end tag
final String foundString = foundTagBuilder.toString();
if (startHtmlTagList.contains(foundString) || endHtmlTagList.contains(foundString))
foundTag = foundString;
}
break;
}
}
}
return foundTag;
}
private static final String[] startHtmlTags = new String[] {
"",
"",
"",
"",
"",
"