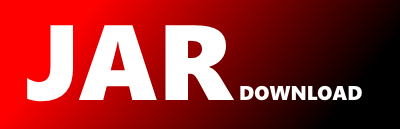
io.github.nichetoolkit.mybatis.MybatisContextHolder Maven / Gradle / Ivy
Show all versions of mybatis-toolkit-context Show documentation
package io.github.nichetoolkit.mybatis;
import io.github.nichetoolkit.mybatis.configure.MybatisCacheProperties;
import io.github.nichetoolkit.mybatis.configure.MybatisTableProperties;
import io.github.nichetoolkit.rice.ServiceIntend;
import lombok.Setter;
import lombok.extern.slf4j.Slf4j;
import org.apache.ibatis.mapping.SqlSource;
import org.apache.ibatis.session.Configuration;
import javax.annotation.Resource;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* MybatisContextHolder
* The mybatis context holder class.
* @see io.github.nichetoolkit.rice.ServiceIntend
* @see lombok.extern.slf4j.Slf4j
* @see lombok.Setter
* @author Cyan ([email protected])
* @since Jdk1.8
*/
@Slf4j
@Setter
public class MybatisContextHolder implements ServiceIntend {
/**
* tableProperties
* {@link io.github.nichetoolkit.mybatis.configure.MybatisTableProperties} The tableProperties
field.
* @see io.github.nichetoolkit.mybatis.configure.MybatisTableProperties
* @see javax.annotation.Resource
*/
@Resource
private MybatisTableProperties tableProperties;
/**
* cacheProperties
* {@link io.github.nichetoolkit.mybatis.configure.MybatisCacheProperties} The cacheProperties
field.
* @see io.github.nichetoolkit.mybatis.configure.MybatisCacheProperties
* @see javax.annotation.Resource
*/
@Resource
private MybatisCacheProperties cacheProperties;
/**
* IS_USE_ONCE
* The constant IS_USE_ONCE
field.
*/
private static boolean IS_USE_ONCE;
/**
* SQL_CACHES
* {@link java.util.Map} The SQL_CACHES
field.
* @see java.util.Map
*/
private static Map SQL_CACHES;
/**
* SQL_SOURCE_CACHES
* {@link java.util.Map} The SQL_SOURCE_CACHES
field.
* @see java.util.Map
*/
private static Map> SQL_SOURCE_CACHES;
/**
* INSTANCE
* {@link io.github.nichetoolkit.mybatis.MybatisContextHolder} The constant INSTANCE
field.
*/
private static MybatisContextHolder INSTANCE = null;
/**
* instance
* The instance method.
* @return {@link io.github.nichetoolkit.mybatis.MybatisContextHolder} The instance return object is MybatisContextHolder
type.
*/
public static MybatisContextHolder instance() {
return INSTANCE;
}
@Override
public void afterPropertiesSet() {
INSTANCE = this;
}
@Override
public void afterAutowirePropertiesSet() {
SQL_SOURCE_CACHES = new ConcurrentHashMap<>(4);
SQL_CACHES = new ConcurrentHashMap<>(this.cacheProperties.getInitSize());
IS_USE_ONCE = this.cacheProperties.isUseOnce();
}
/**
* isUseOnce
* The is use once method.
* @return boolean The is use once return object is boolean
type.
*/
public static boolean isUseOnce() {
return IS_USE_ONCE;
}
/**
* sqlScriptShow
* The sql script show method.
* @return boolean The sql script show return object is boolean
type.
*/
public static boolean sqlScriptShow() {
return INSTANCE.tableProperties.getSqlScriptShow();
}
/**
* tableProperties
* The table properties method.
* @return {@link io.github.nichetoolkit.mybatis.configure.MybatisTableProperties} The table properties return object is MybatisTableProperties
type.
* @see io.github.nichetoolkit.mybatis.configure.MybatisTableProperties
*/
public static MybatisTableProperties tableProperties() {
return INSTANCE.tableProperties;
}
/**
* cacheProperties
* The cache properties method.
* @return {@link io.github.nichetoolkit.mybatis.configure.MybatisCacheProperties} The cache properties return object is MybatisCacheProperties
type.
* @see io.github.nichetoolkit.mybatis.configure.MybatisCacheProperties
*/
public static MybatisCacheProperties cacheProperties() {
return INSTANCE.cacheProperties;
}
/**
* putSqlCache
* The put sql cache method.
* @param cacheKey {@link java.lang.String} The cache key parameter is String
type.
* @param sqlCache {@link io.github.nichetoolkit.mybatis.MybatisSqlCache} The sql cache parameter is MybatisSqlCache
type.
* @see java.lang.String
* @see io.github.nichetoolkit.mybatis.MybatisSqlCache
* @return {@link io.github.nichetoolkit.mybatis.MybatisSqlCache} The put sql cache return object is MybatisSqlCache
type.
*/
public static MybatisSqlCache putSqlCache(String cacheKey, MybatisSqlCache sqlCache) {
return SQL_CACHES.put(cacheKey,sqlCache);
}
/**
* getSqlCache
* The get sql cache getter method.
* @param cacheKey {@link java.lang.String} The cache key parameter is String
type.
* @see java.lang.String
* @see io.github.nichetoolkit.mybatis.MybatisSqlCache
* @return {@link io.github.nichetoolkit.mybatis.MybatisSqlCache} The get sql cache return object is MybatisSqlCache
type.
*/
public static MybatisSqlCache getSqlCache(String cacheKey) {
return SQL_CACHES.get(cacheKey);
}
/**
* containsKey
* The contains key method.
* @param cacheKey {@link java.lang.String} The cache key parameter is String
type.
* @see java.lang.String
* @return boolean The contains key return object is boolean
type.
*/
public static boolean containsKey(String cacheKey) {
return SQL_CACHES.containsKey(cacheKey);
}
/**
* containsKey
* The contains key method.
* @param configuration {@link org.apache.ibatis.session.Configuration} The configuration parameter is Configuration
type.
* @see org.apache.ibatis.session.Configuration
* @return boolean The contains key return object is boolean
type.
*/
public static boolean containsKey(Configuration configuration) {
return SQL_SOURCE_CACHES.containsKey(configuration);
}
/**
* containsKey
* The contains key method.
* @param configuration {@link org.apache.ibatis.session.Configuration} The configuration parameter is Configuration
type.
* @param cacheKey {@link java.lang.String} The cache key parameter is String
type.
* @see org.apache.ibatis.session.Configuration
* @see java.lang.String
* @return boolean The contains key return object is boolean
type.
*/
public static boolean containsKey(Configuration configuration, String cacheKey) {
return SQL_SOURCE_CACHES.get(configuration).containsKey(cacheKey);
}
/**
* computeIfAbsent
* The compute if absent method.
* @param configuration {@link org.apache.ibatis.session.Configuration} The configuration parameter is Configuration
type.
* @see org.apache.ibatis.session.Configuration
* @see java.util.Map
* @return {@link java.util.Map} The compute if absent return object is Map
type.
*/
public static Map computeIfAbsent(Configuration configuration) {
return SQL_SOURCE_CACHES.computeIfAbsent(configuration, k -> new HashMap<>());
}
/**
* getSqlSource
* The get sql source getter method.
* @param configuration {@link org.apache.ibatis.session.Configuration} The configuration parameter is Configuration
type.
* @see org.apache.ibatis.session.Configuration
* @see java.util.Map
* @return {@link java.util.Map} The get sql source return object is Map
type.
*/
public static Map getSqlSource(Configuration configuration) {
return SQL_SOURCE_CACHES.get(configuration);
}
/**
* getSqlSource
* The get sql source getter method.
* @param configuration {@link org.apache.ibatis.session.Configuration} The configuration parameter is Configuration
type.
* @param cacheKey {@link java.lang.String} The cache key parameter is String
type.
* @see org.apache.ibatis.session.Configuration
* @see java.lang.String
* @see org.apache.ibatis.mapping.SqlSource
* @return {@link org.apache.ibatis.mapping.SqlSource} The get sql source return object is SqlSource
type.
*/
public static SqlSource getSqlSource(Configuration configuration, String cacheKey) {
return SQL_SOURCE_CACHES.get(configuration).get(cacheKey);
}
}