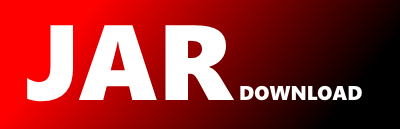
io.github.nichetoolkit.mybatis.MybatisEntityClassFinder Maven / Gradle / Ivy
Show all versions of mybatis-toolkit-context Show documentation
package io.github.nichetoolkit.mybatis;
import io.github.nichetoolkit.rest.reflect.DefaultGenericArrayType;
import io.github.nichetoolkit.rest.reflect.DefaultParameterizedType;
import io.github.nichetoolkit.rest.reflect.DefaultWildcardType;
import io.github.nichetoolkit.rest.reflect.RestGenericTypes;
import org.springframework.lang.NonNull;
import org.springframework.lang.Nullable;
import java.lang.reflect.Method;
import java.lang.reflect.Type;
import java.util.*;
import java.util.function.Predicate;
/**
* MybatisEntityClassFinder
* The mybatis entity class finder class.
* @author Cyan ([email protected])
* @see io.github.nichetoolkit.mybatis.MybatisClassFinder
* @since Jdk1.8
*/
public abstract class MybatisEntityClassFinder implements MybatisClassFinder {
@Override
public Optional> findEntity(@NonNull Class> mapperType, @Nullable Method mapperMethod) {
/* 先判断返回值 */
Optional> optionalClass;
if (mapperMethod != null) {
optionalClass = entityTypeByReturnType(mapperType, mapperMethod);
if (optionalClass.isPresent()) {
return optionalClass;
}
/* 再判断参数 */
optionalClass = entityTypeByParamTypes(mapperType, mapperMethod);
if (optionalClass.isPresent()) {
return optionalClass;
}
/* 最后从接口泛型中获取 */
optionalClass = entityTypeByMapperMethod(mapperType, mapperMethod);
if (optionalClass.isPresent()) {
return optionalClass;
}
}
return entityTypeByMapperType(mapperType);
}
@Override
public Optional> findIdentity(@NonNull Class> mapperType, @Nullable Method mapperMethod, @NonNull Class> entityType) {
/* entityType 泛型寻找 */
Optional> optionalClass;
if (mapperMethod != null) {
/* 判断参数 */
optionalClass = identityTypeByParamTypes(mapperType, mapperMethod);
if (optionalClass.isPresent()) {
return optionalClass;
}
}
optionalClass = identityTypeByEntityType(entityType);
if (optionalClass.isPresent()) {
return optionalClass;
}
/* mapperType 泛型寻找 */
return identityTypeByMapperType(mapperType);
}
public Optional> findLinkage(@NonNull Class> mapperType, @Nullable Method mapperMethod, @NonNull Class> entityType) {
/* entityType 泛型寻找 */
Optional> optionalClass;
if (mapperMethod != null) {
/* 判断参数 */
optionalClass = linkageTypeByParamTypes(mapperType, mapperMethod);
if (optionalClass.isPresent()) {
return optionalClass;
}
}
optionalClass = linkageTypeByEntityType(entityType);
if (optionalClass.isPresent()) {
return optionalClass;
}
/* mapperType 泛型寻找 */
return linkageTypeByMapperType(mapperType);
}
public Optional> findAlertness(@NonNull Class> mapperType, @Nullable Method mapperMethod, @NonNull Class> entityType) {
/* entityType 泛型寻找 */
Optional> optionalClass;
if (mapperMethod != null) {
/* 判断参数 */
optionalClass = alertnessTypeByParamTypes(mapperType, mapperMethod);
if (optionalClass.isPresent()) {
return optionalClass;
}
}
optionalClass = alertnessTypeByEntityType(entityType);
if (optionalClass.isPresent()) {
return optionalClass;
}
/* mapperType 泛型寻找 */
return alertnessTypeByMapperType(mapperType);
}
/**
* entityTypeByReturnType
* The entity type by return type method.
* @param mapperType {@link java.lang.Class} The mapper type parameter is Class
type.
* @param mapperMethod {@link java.lang.reflect.Method} The mapper method parameter is Method
type.
* @return {@link java.util.Optional} The entity type by return type return object is Optional
type.
* @see java.lang.Class
* @see java.lang.reflect.Method
* @see java.util.Optional
*/
protected Optional> entityTypeByReturnType(Class> mapperType, Method mapperMethod) {
Class> returnType = MybatisGenericTypes.resolveMapperReturnType(mapperMethod, mapperType);
return isEntity(returnType) ? Optional.of(returnType) : Optional.empty();
}
/**
* entityTypeByParamTypes
* The entity type by param types method.
* @param mapperType {@link java.lang.Class} The mapper type parameter is Class
type.
* @param mapperMethod {@link java.lang.reflect.Method} The mapper method parameter is Method
type.
* @return {@link java.util.Optional} The entity type by param types return object is Optional
type.
* @see java.lang.Class
* @see java.lang.reflect.Method
* @see java.util.Optional
*/
protected Optional> entityTypeByParamTypes(Class> mapperType, Method mapperMethod) {
return classOfByTypes(RestGenericTypes.resolveParamTypes(mapperMethod, mapperType), this::isEntity);
}
/**
* entityTypeByMapperMethod
* The entity type by mapper method method.
* @param mapperType {@link java.lang.Class} The mapper type parameter is Class
type.
* @param mapperMethod {@link java.lang.reflect.Method} The mapper method parameter is Method
type.
* @return {@link java.util.Optional} The entity type by mapper method return object is Optional
type.
* @see java.lang.Class
* @see java.lang.reflect.Method
* @see java.util.Optional
*/
protected Optional> entityTypeByMapperMethod(Class> mapperType, Method mapperMethod) {
return classOfByTypes(RestGenericTypes.resolveMethodTypes(mapperMethod, mapperType), this::isEntity);
}
/**
* identityTypeByEntityType
* The identity type by entity type method.
* @param entityType {@link java.lang.Class} The entity type parameter is Class
type.
* @return {@link java.util.Optional} The identity type by entity type return object is Optional
type.
* @see java.lang.Class
* @see java.util.Optional
*/
protected Optional> identityTypeByEntityType(Class> entityType) {
return classOfByTypes(RestGenericTypes.resolveSuperclassTypes(entityType), this::isIdentity);
}
/**
* identityTypeByParamTypes
* The identity type by param types method.
* @param mapperType {@link java.lang.Class} The mapper type parameter is Class
type.
* @param mapperMethod {@link java.lang.reflect.Method} The mapper method parameter is Method
type.
* @return {@link java.util.Optional} The identity type by param types return object is Optional
type.
* @see java.lang.Class
* @see java.lang.reflect.Method
* @see java.util.Optional
*/
protected Optional> identityTypeByParamTypes(Class> mapperType, Method mapperMethod) {
return classOfByTypes(RestGenericTypes.resolveParamTypes(mapperMethod, mapperType), this::isIdentity);
}
/**
* linkageTypeByEntityType
* The linkage type by entity type method.
* @param entityType {@link java.lang.Class} The entity type parameter is Class
type.
* @return {@link java.util.Optional} The linkage type by entity type return object is Optional
type.
* @see java.lang.Class
* @see java.util.Optional
*/
protected Optional> linkageTypeByEntityType(Class> entityType) {
return classOfByTypes(RestGenericTypes.resolveSuperclassTypes(entityType), this::isLinkage);
}
/**
* linkageTypeByParamTypes
* The linkage type by param types method.
* @param mapperType {@link java.lang.Class} The mapper type parameter is Class
type.
* @param mapperMethod {@link java.lang.reflect.Method} The mapper method parameter is Method
type.
* @return {@link java.util.Optional} The linkage type by param types return object is Optional
type.
* @see java.lang.Class
* @see java.lang.reflect.Method
* @see java.util.Optional
*/
protected Optional> linkageTypeByParamTypes(Class> mapperType, Method mapperMethod) {
return classOfByTypes(RestGenericTypes.resolveParamTypes(mapperMethod, mapperType), this::isLinkage);
}
/**
* alertnessTypeByEntityType
* The alertness type by entity type method.
* @param entityType {@link java.lang.Class} The entity type parameter is Class
type.
* @return {@link java.util.Optional} The alertness type by entity type return object is Optional
type.
* @see java.lang.Class
* @see java.util.Optional
*/
protected Optional> alertnessTypeByEntityType(Class> entityType) {
return classOfByTypes(RestGenericTypes.resolveSuperclassTypes(entityType), this::isAlertness);
}
/**
* alertnessTypeByParamTypes
* The alertness type by param types method.
* @param mapperType {@link java.lang.Class} The mapper type parameter is Class
type.
* @param mapperMethod {@link java.lang.reflect.Method} The mapper method parameter is Method
type.
* @return {@link java.util.Optional} The alertness type by param types return object is Optional
type.
* @see java.lang.Class
* @see java.lang.reflect.Method
* @see java.util.Optional
*/
protected Optional> alertnessTypeByParamTypes(Class> mapperType, Method mapperMethod) {
return classOfByTypes(RestGenericTypes.resolveParamTypes(mapperMethod, mapperType), this::isAlertness);
}
/**
* entityTypeByMapperType
* The entity type by mapper type method.
* @param mapperType {@link java.lang.Class} The mapper type parameter is Class
type.
* @return {@link java.util.Optional} The entity type by mapper type return object is Optional
type.
* @see java.lang.Class
* @see java.util.Optional
*/
protected Optional> entityTypeByMapperType(Class> mapperType) {
return classOfByTypes(RestGenericTypes.resolveInterfaceTypes(mapperType), this::isEntity);
}
/**
* identityTypeByMapperType
* The identity type by mapper type method.
* @param mapperType {@link java.lang.Class} The mapper type parameter is Class
type.
* @return {@link java.util.Optional} The identity type by mapper type return object is Optional
type.
* @see java.lang.Class
* @see java.util.Optional
*/
protected Optional> identityTypeByMapperType(Class> mapperType) {
return classOfByTypes(RestGenericTypes.resolveInterfaceTypes(mapperType), this::isIdentity);
}
/**
* linkageTypeByMapperType
* The linkage type by mapper type method.
* @param mapperType {@link java.lang.Class} The mapper type parameter is Class
type.
* @return {@link java.util.Optional} The linkage type by mapper type return object is Optional
type.
* @see java.lang.Class
* @see java.util.Optional
*/
protected Optional> linkageTypeByMapperType(Class> mapperType) {
return classOfByTypes(RestGenericTypes.resolveInterfaceTypes(mapperType), this::isLinkage);
}
/**
* alertnessTypeByMapperType
* The alertness type by mapper type method.
* @param mapperType {@link java.lang.Class} The mapper type parameter is Class
type.
* @return {@link java.util.Optional} The alertness type by mapper type return object is Optional
type.
* @see java.lang.Class
* @see java.util.Optional
*/
protected Optional> alertnessTypeByMapperType(Class> mapperType) {
return classOfByTypes(RestGenericTypes.resolveInterfaceTypes(mapperType), this::isAlertness);
}
/**
* classOfByType
* The class of by type method.
* @param type {@link java.lang.reflect.Type} The type parameter is Type
type.
* @param predicate {@link java.util.function.Predicate} The predicate parameter is Predicate
type.
* @return {@link java.util.Optional} The class of by type return object is Optional
type.
* @see java.lang.reflect.Type
* @see java.util.function.Predicate
* @see java.util.Optional
*/
protected Optional> classOfByType(Type type, Predicate> predicate) {
if (type instanceof Class) {
Class> clazz = (Class>) type;
if (predicate.test(clazz)) {
return Optional.of((Class>) type);
}
} else if (type instanceof DefaultParameterizedType) {
return classOfByTypes(((DefaultParameterizedType) type).getActualTypeArguments(), predicate);
} else if (type instanceof DefaultWildcardType) {
Optional> optionalClass = classOfByTypes(((DefaultWildcardType) type).getLowerBounds(), predicate);
if (optionalClass.isPresent()) {
return optionalClass;
}
return classOfByTypes(((DefaultWildcardType) type).getUpperBounds(), predicate);
} else if (type instanceof DefaultGenericArrayType) {
return classOfByType(((DefaultGenericArrayType) type).getGenericComponentType(), predicate);
}
return Optional.empty();
}
/**
* classOfByTypes
* The class of by types method.
* @param types {@link java.lang.reflect.Type} The types parameter is Type
type.
* @param predicate {@link java.util.function.Predicate} The predicate parameter is Predicate
type.
* @return {@link java.util.Optional} The class of by types return object is Optional
type.
* @see java.lang.reflect.Type
* @see java.util.function.Predicate
* @see java.util.Optional
*/
protected Optional> classOfByTypes(Type[] types, Predicate> predicate) {
for (Type type : types) {
Optional> optionalClass = classOfByType(type, predicate);
if (optionalClass.isPresent()) {
return optionalClass;
}
}
return Optional.empty();
}
}