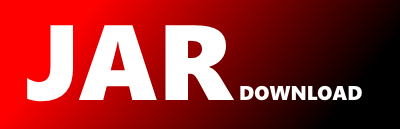
io.github.nichetoolkit.mybatis.MybatisSqlScript Maven / Gradle / Ivy
Show all versions of mybatis-toolkit-context Show documentation
package io.github.nichetoolkit.mybatis;
import io.github.nichetoolkit.rice.consts.EntityConstants;
import io.github.nichetoolkit.rice.consts.SQLConstants;
import io.github.nichetoolkit.rice.consts.ScriptConstants;
import io.github.nichetoolkit.mybatis.error.MybatisAssertErrorException;
import io.github.nichetoolkit.rest.RestException;
import io.github.nichetoolkit.rest.actuator.SupplierActuator;
import io.github.nichetoolkit.rest.util.OptionalUtils;
import org.apache.ibatis.builder.annotation.ProviderContext;
/**
* MybatisSqlScript
* The mybatis sql script interface.
* @author Cyan ([email protected])
* @see io.github.nichetoolkit.mybatis.MybatisOrder
* @since Jdk1.8
*/
public interface MybatisSqlScript extends MybatisOrder {
/**
* caching
* The caching method.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param sqlScript {@link io.github.nichetoolkit.mybatis.MybatisSqlScript} The sql script parameter is MybatisSqlScript
type.
* @return {@link java.lang.String} The caching return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
static String caching(ProviderContext providerContext, MybatisSqlScript sqlScript) throws RestException {
MybatisTable table = MybatisFactory.createTable(providerContext.getMapperType(), providerContext.getMapperMethod());
return MybatisSqlSourceCaching.cache(providerContext, table, () -> {
MybatisSqlScript restSqlScript = MybatisSqlScriptResolver.ofResolve(providerContext, table, sqlScript);
String sql = restSqlScript.sql(table);
return String.format(ScriptConstants.SCRIPT_LABEL, sql);
});
}
/**
* caching
* The caching method.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param sqlScript {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.SimpleSqlScript} The sql script parameter is SimpleSqlScript
type.
* @return {@link java.lang.String} The caching return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.SimpleSqlScript
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
static String caching(ProviderContext providerContext, MybatisSqlScript.SimpleSqlScript sqlScript) throws RestException {
return caching(providerContext, (MybatisSqlScript) sqlScript);
}
/**
* where
* The where method.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The where return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String where(LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.WHERE_LABEL, content.withLinefeed());
}
/**
* sql
* The sql method.
* @param table {@link io.github.nichetoolkit.mybatis.MybatisTable} The table parameter is MybatisTable
type.
* @return {@link java.lang.String} The sql return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see io.github.nichetoolkit.mybatis.MybatisTable
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
String sql(MybatisTable table) throws RestException;
/**
* choose
* The choose method.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The choose return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String choose(LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.CHOOSE_LABEL, content.withLinefeed());
}
/**
* otherwise
* The otherwise method.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The otherwise return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String otherwise(LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.OTHERWISE_LABEL, content.withLinefeed());
}
/**
* set
* The set method.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The set return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String set(LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.SET_LABEL, content.withLinefeed());
}
/**
* ifTest
* The if test method.
* @param test {@link java.lang.String} The test parameter is String
type.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The if test return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see io.github.nichetoolkit.rest.RestException
*/
default String ifTest(String test, LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.IF_TEST_LABEL, test, content.withLinefeed());
}
/**
* ifParameterNotNull
* The if parameter not null method.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The if parameter not null return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String ifParameterNotNull(LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.IF_TEST_PARAM_LABEL, content.withLinefeed());
}
/**
* parameterNotNull
* The parameter not null method.
* @param message {@link java.lang.String} The message parameter is String
type.
* @return {@link java.lang.String} The parameter not null return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String parameterNotNull(String message) throws RestException {
return variableNotNull(ScriptConstants.PARAM, message);
}
/**
* variableIsTrue
* The variable is true method.
* @param variable {@link java.lang.String} The variable parameter is String
type.
* @param message {@link java.lang.String} The message parameter is String
type.
* @return {@link java.lang.String} The variable is true return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String variableIsTrue(String variable, String message) throws RestException {
OptionalUtils.ofFalse(Boolean.parseBoolean(variable), message, error -> new MybatisAssertErrorException("isTrue", "variable", error));
return variable;
}
/**
* variableIsFalse
* The variable is false method.
* @param variable {@link java.lang.String} The variable parameter is String
type.
* @param message {@link java.lang.String} The message parameter is String
type.
* @return {@link java.lang.String} The variable is false return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String variableIsFalse(String variable, String message) throws RestException {
OptionalUtils.ofTrue(Boolean.parseBoolean(variable), message, error -> new MybatisAssertErrorException("isFalse", "variable", error));
return variable;
}
/**
* variableNotNull
* The variable not null method.
* @param variable {@link java.lang.String} The variable parameter is String
type.
* @param message {@link java.lang.String} The message parameter is String
type.
* @return {@link java.lang.String} The variable not null return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String variableNotNull(String variable, String message) throws RestException {
OptionalUtils.ofNull(variable, message, error -> new MybatisAssertErrorException("notNull", "variable", error));
return variable;
}
/**
* variableNotEmpty
* The variable not empty method.
* @param variable {@link java.lang.String} The variable parameter is String
type.
* @param message {@link java.lang.String} The message parameter is String
type.
* @return {@link java.lang.String} The variable not empty return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String variableNotEmpty(String variable, String message) throws RestException {
OptionalUtils.ofEmpty(variable, message, error -> new MybatisAssertErrorException("notEmpty", "variable", error));
return variable;
}
/**
* whenTest
* The when test method.
* @param test {@link java.lang.String} The test parameter is String
type.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The when test return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see io.github.nichetoolkit.rest.RestException
*/
default String whenTest(String test, LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.WHEN_TEST_LABEL, test, content.withLinefeed());
}
/**
* trim
* The trim method.
* @param prefix {@link java.lang.String} The prefix parameter is String
type.
* @param suffix {@link java.lang.String} The suffix parameter is String
type.
* @param prefixOverrides {@link java.lang.String} The prefix overrides parameter is String
type.
* @param suffixOverrides {@link java.lang.String} The suffix overrides parameter is String
type.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The trim return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see io.github.nichetoolkit.rest.RestException
*/
default String trim(String prefix, String suffix, String prefixOverrides, String suffixOverrides, LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.TRIM_LABEL, prefix, prefixOverrides, suffixOverrides, suffix, content.withLinefeed());
}
/**
* trimPrefixOverrides
* The trim prefix overrides method.
* @param prefix {@link java.lang.String} The prefix parameter is String
type.
* @param suffix {@link java.lang.String} The suffix parameter is String
type.
* @param prefixOverrides {@link java.lang.String} The prefix overrides parameter is String
type.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The trim prefix overrides return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see io.github.nichetoolkit.rest.RestException
*/
default String trimPrefixOverrides(String prefix, String suffix, String prefixOverrides, LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.TRIM_PREFIX_OVERRIDE_LABEL, prefix, prefixOverrides, suffix, content.withLinefeed());
}
/**
* trimSuffixOverrides
* The trim suffix overrides method.
* @param prefix {@link java.lang.String} The prefix parameter is String
type.
* @param suffix {@link java.lang.String} The suffix parameter is String
type.
* @param suffixOverrides {@link java.lang.String} The suffix overrides parameter is String
type.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The trim suffix overrides return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see io.github.nichetoolkit.rest.RestException
*/
default String trimSuffixOverrides(String prefix, String suffix, String suffixOverrides, LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.TRIM_SUFFIX_OVERRIDE_LABEL, prefix, suffixOverrides, suffix, content.withLinefeed());
}
/**
* foreach
* The foreach method.
* @param collection {@link java.lang.String} The collection parameter is String
type.
* @param item {@link java.lang.String} The item parameter is String
type.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The foreach return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see io.github.nichetoolkit.rest.RestException
*/
default String foreach(String collection, String item, LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.FOREACH_LABEL, collection, item, content.withLinefeed());
}
/**
* foreach
* The foreach method.
* @param collection {@link java.lang.String} The collection parameter is String
type.
* @param item {@link java.lang.String} The item parameter is String
type.
* @param separator {@link java.lang.String} The separator parameter is String
type.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The foreach return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see io.github.nichetoolkit.rest.RestException
*/
default String foreach(String collection, String item, String separator, LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.FOREACH_SEPARATOR_LABEL, collection, item, separator, content.withLinefeed());
}
/**
* foreach
* The foreach method.
* @param collection {@link java.lang.String} The collection parameter is String
type.
* @param item {@link java.lang.String} The item parameter is String
type.
* @param separator {@link java.lang.String} The separator parameter is String
type.
* @param open {@link java.lang.String} The open parameter is String
type.
* @param close {@link java.lang.String} The close parameter is String
type.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The foreach return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see io.github.nichetoolkit.rest.RestException
*/
default String foreach(String collection, String item, String separator, String open, String close, LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.FOREACH_BRACE_LABEL, collection, item, open, close, separator, content.withLinefeed());
}
/**
* foreach
* The foreach method.
* @param collection {@link java.lang.String} The collection parameter is String
type.
* @param item {@link java.lang.String} The item parameter is String
type.
* @param separator {@link java.lang.String} The separator parameter is String
type.
* @param open {@link java.lang.String} The open parameter is String
type.
* @param close {@link java.lang.String} The close parameter is String
type.
* @param index {@link java.lang.String} The index parameter is String
type.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The foreach return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see io.github.nichetoolkit.rest.RestException
*/
default String foreach(String collection, String item, String separator, String open, String close, String index, LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.FOREACH_INDEX_LABEL, collection, item, index, open, close, separator, content.withLinefeed());
}
/**
* foreachOfIdList
* The foreach of id list method.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The foreach of id list return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String foreachOfIdList(LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.FOREACH_INDEX_LABEL, EntityConstants.IDENTITY_LIST, EntityConstants.IDENTITY, EntityConstants.INDEX, SQLConstants.BRACE_LT, SQLConstants.BRACE_GT, SQLConstants.COMMA + SQLConstants.BLANK, content.withLinefeed());
}
/**
* foreachOfLinkIdList
* The foreach of link id list method.
* @param content {@link io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier} The content parameter is LinefeedSupplier
type.
* @return {@link java.lang.String} The foreach of link id list return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript.LinefeedSupplier
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String foreachOfLinkIdList(LinefeedSupplier content) throws RestException {
return String.format(ScriptConstants.FOREACH_INDEX_LABEL, EntityConstants.LINK_ID_LIST, EntityConstants.LINK_ID, EntityConstants.INDEX, SQLConstants.BRACE_LT, SQLConstants.BRACE_GT, SQLConstants.COMMA + SQLConstants.BLANK, content.withLinefeed());
}
/**
* bind
* The bind method.
* @param name {@link java.lang.String} The name parameter is String
type.
* @param value {@link java.lang.String} The value parameter is String
type.
* @return {@link java.lang.String} The bind return object is String
type.
* @see java.lang.String
*/
default String bind(String name, String value) {
return String.format(ScriptConstants.BIND_LABEL, name, value);
}
/**
* LinefeedSupplier
* The linefeed supplier interface.
* @author Cyan ([email protected])
* @see io.github.nichetoolkit.rest.actuator.SupplierActuator
* @since Jdk1.8
*/
interface LinefeedSupplier extends SupplierActuator {
/**
* withLinefeed
* The with linefeed method.
* @return {@link java.lang.String} The with linefeed return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
default String withLinefeed() throws RestException {
String linefeed = get();
if (!linefeed.isEmpty() && linefeed.charAt(0) == SQLConstants.LINEFEED.charAt(0)) {
return linefeed;
}
return SQLConstants.LINEFEED + linefeed;
}
}
/**
* SimpleSqlScript
* The simple sql script interface.
* @author Cyan ([email protected])
* @since Jdk1.8
*/
interface SimpleSqlScript extends MybatisSqlScript {
@Override
default String sql(MybatisTable table) throws RestException {
return sql(table, this);
}
/**
* sql
* The sql method.
* @param table {@link io.github.nichetoolkit.mybatis.MybatisTable} The table parameter is MybatisTable
type.
* @param sqlScript {@link io.github.nichetoolkit.mybatis.MybatisSqlScript} The sql script parameter is MybatisSqlScript
type.
* @return {@link java.lang.String} The sql return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see io.github.nichetoolkit.mybatis.MybatisTable
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
String sql(MybatisTable table, MybatisSqlScript sqlScript) throws RestException;
}
}