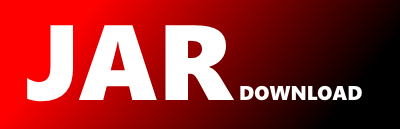
io.github.nichetoolkit.mybatis.MybatisSqlProvider Maven / Gradle / Ivy
Show all versions of mybatis-toolkit-starter Show documentation
package io.github.nichetoolkit.mybatis;
import io.github.nichetoolkit.mybatis.builder.SqlBuilder;
import io.github.nichetoolkit.mybatis.consts.EntityConstants;
import io.github.nichetoolkit.mybatis.consts.SQLConstants;
import io.github.nichetoolkit.mybatis.consts.ScriptConstants;
import io.github.nichetoolkit.mybatis.enums.DatabaseType;
import io.github.nichetoolkit.mybatis.error.MybatisParamErrorException;
import io.github.nichetoolkit.rest.RestException;
import io.github.nichetoolkit.rest.RestKey;
import io.github.nichetoolkit.rest.RestOptional;
import io.github.nichetoolkit.rest.RestReckon;
import io.github.nichetoolkit.rest.actuator.ConsumerActuator;
import io.github.nichetoolkit.rest.stream.RestCollectors;
import io.github.nichetoolkit.rest.stream.RestStream;
import io.github.nichetoolkit.rest.util.GeneralUtils;
import io.github.nichetoolkit.rest.util.OptionalUtils;
import org.apache.ibatis.builder.annotation.ProviderContext;
import org.springframework.lang.Nullable;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Collectors;
/**
* MybatisSqlProvider
* The mybatis sql provider interface.
* @author Cyan ([email protected])
* @since Jdk1.8
*/
public interface MybatisSqlProvider {
/**
* databaseType
* The database type method.
* @return {@link io.github.nichetoolkit.mybatis.enums.DatabaseType} The database type return object is DatabaseType
type.
* @see io.github.nichetoolkit.mybatis.enums.DatabaseType
*/
DatabaseType databaseType();
/**
* SELECT_SQL_SUPPLY
* {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The constant SELECT_SQL_SUPPLY
field.
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
*/
MybatisSqlSupply.SimpleSqlSupply SELECT_SQL_SUPPLY = (tablename, table, sqlBuilder) ->
SqlBuilder.sqlBuilder()
.select().append(table.sqlOfSelectColumns())
.from().append(table.tablename(tablename))
.where().append(sqlBuilder).toString();
/**
* SAVE_SQL_SUPPLY
* {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The constant SAVE_SQL_SUPPLY
field.
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
*/
MybatisSqlSupply.SimpleSqlSupply SAVE_SQL_SUPPLY = (tablename, table, sqlBuilder) ->
SqlBuilder.sqlBuilder()
.insert().append(table.tablename(tablename))
.braceLt().append(table.sqlOfInsertColumns()).braceGt()
.values().append(sqlBuilder).toString();
/**
* REMOVE_SQL_SUPPLY
* {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The constant REMOVE_SQL_SUPPLY
field.
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
*/
MybatisSqlSupply.SimpleSqlSupply REMOVE_SQL_SUPPLY = (tablename, table, sqlBuilder) ->
SqlBuilder.sqlBuilder()
.update().append(table.tablename(tablename))
.set().append(table.getLogicColumn().columnEqualsProperty())
.comma().append(table.sqlOfForceUpdateColumns())
.where().append(sqlBuilder).toString();
/**
* OPERATE_SQL_SUPPLY
* {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The constant OPERATE_SQL_SUPPLY
field.
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
*/
MybatisSqlSupply.SimpleSqlSupply OPERATE_SQL_SUPPLY = (tablename, table, sqlBuilder) ->
SqlBuilder.sqlBuilder()
.update().append(table.tablename(tablename))
.set().append(table.getOperateColumn().columnEqualsProperty())
.comma().append(table.sqlOfForceUpdateColumns())
.where().append(sqlBuilder).toString();
/**
* DELETE_SQL_SUPPLY
* {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The constant DELETE_SQL_SUPPLY
field.
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
*/
MybatisSqlSupply.SimpleSqlSupply DELETE_SQL_SUPPLY = (tablename, table, sqlBuilder) ->
SqlBuilder.sqlBuilder()
.delete().from().append(table.tablename(tablename))
.where().append(sqlBuilder).toString();
/**
* ALERT_SQL_SUPPLY
* {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.AlertSqlSupply} The constant ALERT_SQL_SUPPLY
field.
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.AlertSqlSupply
*/
MybatisSqlSupply.AlertSqlSupply ALERT_SQL_SUPPLY = (tablename, table, sqlBuilder, status) ->
SqlBuilder.sqlBuilder()
.update().append(table.tablename(tablename))
.set().append(sqlOfStatus(table, status))
.comma().append(table.sqlOfForceUpdateColumns())
.where().append(sqlBuilder).toString();
/**
* reviseParameter
* The revise parameter method.
* @param {@link java.lang.Object}
The parameter can be of any type.
* @param parameter P The parameter parameter is P
type.
* @return {@link java.lang.Object} The revise parameter return object is Object
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.Object
* @see java.lang.SuppressWarnings
* @see io.github.nichetoolkit.rest.RestException
*/
@SuppressWarnings("unchecked")
static Object reviseParameter(P parameter) throws RestException {
Class> parameterClass = parameter.getClass();
if (Map.class.isAssignableFrom(parameterClass)) {
Map param = (Map) parameter;
Optional> firstParam = param.values().stream().findFirst();
return firstParam.orElseThrow(MybatisParamErrorException::new);
} else {
return parameter;
}
}
/**
* sqlOfStatus
* The sql of status method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param table {@link io.github.nichetoolkit.mybatis.MybatisTable} The table parameter is MybatisTable
type.
* @param status S The status parameter is S
type.
* @return {@link java.lang.String} The sql of status return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see io.github.nichetoolkit.mybatis.MybatisTable
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
static String sqlOfStatus(MybatisTable table, S status) throws RestException {
SqlBuilder sqlBuilder = SqlBuilder.sqlBuilder();
if (table.isSpecialAlertness()) {
valueOfParameter(table.alertnessColumns(), status, table.getAlertnessType());
String statusSql = sqlOfColumns(status, table.alertnessColumns(), false, true);
sqlBuilder.append(statusSql);
} else {
Optional.ofNullable(table.getAlertColumn()).ifPresent(column -> {
if (RestKey.class.isAssignableFrom(status.getClass())) {
sqlBuilder.append(column.columnName()).eq().value(status);
} else {
sqlBuilder.append(column.columnEqualsProperty());
}
});
}
return sqlBuilder.toString();
}
/**
* providingOfId
* The providing of id method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param idParameter I The id parameter parameter is I
type.
* @param statusParameter S The status parameter parameter is S
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.AlertSqlSupply} The sql supply parameter is AlertSqlSupply
type.
* @return {@link java.lang.String} The providing of id return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.AlertSqlSupply
* @see io.github.nichetoolkit.rest.RestException
*/
static String providingOfId(ProviderContext providerContext, @Nullable String tablename, I idParameter, S statusParameter, MybatisSqlSupply.AlertSqlSupply sqlSupply) throws RestException {
Object status = reviseParameter(statusParameter);
return providingOfId(providerContext, tablename, idParameter, table -> {
}, (tablenameValue, tableValue, sqlBuilder) -> sqlSupply.supply(tablenameValue, tableValue, sqlBuilder, status));
}
/**
* providingOfAll
* The providing of all method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param idList {@link java.util.Collection} The id list parameter is Collection
type.
* @param statusParameter S The status parameter parameter is S
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.AlertSqlSupply} The sql supply parameter is AlertSqlSupply
type.
* @return {@link java.lang.String} The providing of all return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see java.util.Collection
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.AlertSqlSupply
* @see io.github.nichetoolkit.rest.RestException
*/
static String providingOfAll(ProviderContext providerContext, @Nullable String tablename, Collection idList, S statusParameter, MybatisSqlSupply.AlertSqlSupply sqlSupply) throws RestException {
Object status = reviseParameter(statusParameter);
return providingOfAll(providerContext, tablename, idList, table -> {
}, (tablenameValue, tableValue, sqlBuilder) -> sqlSupply.supply(tablenameValue, tableValue, sqlBuilder, status));
}
/**
* providingOfWhere
* The providing of where method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param whereSqlParameter {@link java.lang.String} The where sql parameter parameter is String
type.
* @param statusParameter S The status parameter parameter is S
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.AlertSqlSupply} The sql supply parameter is AlertSqlSupply
type.
* @return {@link java.lang.String} The providing of where return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.AlertSqlSupply
* @see io.github.nichetoolkit.rest.RestException
*/
static String providingOfWhere(ProviderContext providerContext, @Nullable String tablename, String whereSqlParameter, S statusParameter, MybatisSqlSupply.AlertSqlSupply sqlSupply) throws RestException {
Object status = reviseParameter(statusParameter);
return providingOfWhere(providerContext, tablename, whereSqlParameter, table -> {
}, (tablenameValue, tableValue, sqlBuilder) -> sqlSupply.supply(tablenameValue, tableValue, sqlBuilder, status));
}
/**
* providingOfLinkId
* The providing of link id method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param linkIdParameter L The link id parameter parameter is L
type.
* @param statusParameter S The status parameter parameter is S
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.AlertSqlSupply} The sql supply parameter is AlertSqlSupply
type.
* @return {@link java.lang.String} The providing of link id return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.AlertSqlSupply
* @see io.github.nichetoolkit.rest.RestException
*/
static String providingOfLinkId(ProviderContext providerContext, @Nullable String tablename, L linkIdParameter, S statusParameter, MybatisSqlSupply.AlertSqlSupply sqlSupply) throws RestException {
Object status = reviseParameter(statusParameter);
return providingOfLinkId(providerContext, tablename, linkIdParameter, table -> {
}, (tablenameValue, tableValue, sqlBuilder) -> sqlSupply.supply(tablenameValue, tableValue, sqlBuilder, status));
}
/**
* providingOfLinkIdAll
* The providing of link id all method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param linkIdList {@link java.util.Collection} The link id list parameter is Collection
type.
* @param statusParameter S The status parameter parameter is S
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.AlertSqlSupply} The sql supply parameter is AlertSqlSupply
type.
* @return {@link java.lang.String} The providing of link id all return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see java.util.Collection
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.AlertSqlSupply
* @see io.github.nichetoolkit.rest.RestException
*/
static String providingOfLinkIdAll(ProviderContext providerContext, @Nullable String tablename, Collection linkIdList, S statusParameter, MybatisSqlSupply.AlertSqlSupply sqlSupply) throws RestException {
Object status = reviseParameter(statusParameter);
return providingOfLinkIdAll(providerContext, tablename, linkIdList, table -> {
}, (tablenameValue, tableValue, sqlBuilder) -> sqlSupply.supply(tablenameValue, tableValue, sqlBuilder, status));
}
/**
* providingOfLinkId
* The providing of link id method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param linkIdParameter L The link id parameter parameter is L
type.
* @param tableOptional {@link io.github.nichetoolkit.rest.actuator.ConsumerActuator} The table optional parameter is ConsumerActuator
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The sql supply parameter is SimpleSqlSupply
type.
* @return {@link java.lang.String} The providing of link id return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see io.github.nichetoolkit.rest.actuator.ConsumerActuator
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
* @see java.lang.SuppressWarnings
* @see io.github.nichetoolkit.rest.RestException
*/
@SuppressWarnings("Duplicates")
static String providingOfLinkId(ProviderContext providerContext, @Nullable String tablename, L linkIdParameter, ConsumerActuator tableOptional, MybatisSqlSupply.SimpleSqlSupply sqlSupply) throws RestException {
Object linkId = reviseParameter(linkIdParameter);
return MybatisSqlScript.caching(providerContext, (table, sqlScript) -> {
tableOptional.actuate(table);
SqlBuilder sqlBuilder = SqlBuilder.sqlBuilder();
if (table.isSpecialLinkage()) {
valueOfParameter(table.linkageColumns(), linkId, table.getLinkageType());
String linkageSql = sqlOfColumns(linkId, table.getLinkageColumns(), true, true);
sqlBuilder.append(linkageSql);
} else {
Optional.ofNullable(table.getLinkColumn()).ifPresent(column -> sqlBuilder.append(column.columnEqualsProperty()));
}
return sqlSupply.supply(tablename, table, sqlBuilder);
});
}
/**
* providingOfLinkIdAll
* The providing of link id all method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param linkIdList {@link java.util.Collection} The link id list parameter is Collection
type.
* @param tableOptional {@link io.github.nichetoolkit.rest.actuator.ConsumerActuator} The table optional parameter is ConsumerActuator
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The sql supply parameter is SimpleSqlSupply
type.
* @return {@link java.lang.String} The providing of link id all return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see java.util.Collection
* @see io.github.nichetoolkit.rest.actuator.ConsumerActuator
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
* @see java.lang.SuppressWarnings
* @see io.github.nichetoolkit.rest.RestException
*/
@SuppressWarnings("Duplicates")
static String providingOfLinkIdAll(ProviderContext providerContext, @Nullable String tablename, Collection linkIdList, ConsumerActuator tableOptional, MybatisSqlSupply.SimpleSqlSupply sqlSupply) throws RestException {
return MybatisSqlScript.caching(providerContext, (table, sqlScript) -> {
tableOptional.actuate(table);
SqlBuilder sqlBuilder = SqlBuilder.sqlBuilder();
if (table.isSpecialLinkage()) {
RestStream.stream(linkIdList).forEach(linkId -> valueOfParameter(table.linkageColumns(), linkId, table.getLinkageType()));
Map> sliceOfColumnsMap = sliceOfColumns(table.linkageColumns(), linkIdList);
sqlBuilder.append(sqlOfColumns(sliceOfColumnsMap, table.linkageColumns(), sqlScript));
} else {
sqlBuilder.append(table.getIdentityColumn().columnName()).in().braceLt();
linkIdList.forEach(linkId -> sqlBuilder.value(linkId).comma());
sqlBuilder.delete(sqlBuilder.length() - 2).braceGt();
}
return sqlSupply.supply(tablename, table, sqlBuilder);
});
}
/**
* providingOfName
* The providing of name method.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param name {@link java.lang.String} The name parameter is String
type.
* @param tableOptional {@link io.github.nichetoolkit.rest.actuator.ConsumerActuator} The table optional parameter is ConsumerActuator
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The sql supply parameter is SimpleSqlSupply
type.
* @return {@link java.lang.String} The providing of name return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see io.github.nichetoolkit.rest.actuator.ConsumerActuator
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
* @see java.lang.SuppressWarnings
* @see io.github.nichetoolkit.rest.RestException
*/
@SuppressWarnings("Duplicates")
static String providingOfName(ProviderContext providerContext, @Nullable String tablename, String name, ConsumerActuator tableOptional, MybatisSqlSupply.SimpleSqlSupply sqlSupply) throws RestException {
return MybatisSqlScript.caching(providerContext, (table, sqlScript) -> {
tableOptional.actuate(table);
String nameSql = Optional.ofNullable(table.fieldColumn(EntityConstants.NAME)).map(MybatisColumn::columnEqualsProperty).orElse(ScriptConstants.NAME_EQUALS_PROPERTY);
SqlBuilder sqlBuilder = new SqlBuilder(nameSql);
Optional.ofNullable(table.getLogicColumn()).ifPresent(column -> sqlBuilder.and().append(column.columnEqualsProperty()));
return sqlSupply.supply(tablename, table, sqlBuilder);
});
}
/**
* providingOfName
* The providing of name method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param name {@link java.lang.String} The name parameter is String
type.
* @param idParameter I The id parameter parameter is I
type.
* @param tableOptional {@link io.github.nichetoolkit.rest.actuator.ConsumerActuator} The table optional parameter is ConsumerActuator
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The sql supply parameter is SimpleSqlSupply
type.
* @return {@link java.lang.String} The providing of name return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see io.github.nichetoolkit.rest.actuator.ConsumerActuator
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
* @see java.lang.SuppressWarnings
* @see io.github.nichetoolkit.rest.RestException
*/
@SuppressWarnings("Duplicates")
static String providingOfName(ProviderContext providerContext, @Nullable String tablename, String name, I idParameter, ConsumerActuator tableOptional, MybatisSqlSupply.SimpleSqlSupply sqlSupply) throws RestException {
Object identity = reviseParameter(idParameter);
return MybatisSqlScript.caching(providerContext, (table, sqlScript) -> {
tableOptional.actuate(table);
SqlBuilder sqlBuilder = new SqlBuilder();
if (table.isSpecialIdentity()) {
valueOfParameter(table.identityColumns(), identity, table.getIdentityType());
String identitySql = sqlOfColumns(identity, table.identityColumns(), true, false);
sqlBuilder.append(identitySql);
} else {
Optional.ofNullable(table.getIdentityColumn()).ifPresent(column -> sqlBuilder.append(column.columnNotEqualsProperty()));
}
String nameSql = Optional.ofNullable(table.fieldColumn(EntityConstants.NAME)).map(MybatisColumn::columnEqualsProperty).orElse(ScriptConstants.NAME_EQUALS_PROPERTY);
sqlBuilder.and().append(nameSql);
Optional.ofNullable(table.getLogicColumn()).ifPresent(column -> sqlBuilder.and().append(column.columnEqualsProperty()));
return sqlSupply.supply(tablename, table, sqlBuilder);
});
}
/**
* providingOfEntity
* The providing of entity method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param entityParameter E The entity parameter parameter is E
type.
* @param tableOptional {@link io.github.nichetoolkit.rest.actuator.ConsumerActuator} The table optional parameter is ConsumerActuator
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The sql supply parameter is SimpleSqlSupply
type.
* @return {@link java.lang.String} The providing of entity return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see io.github.nichetoolkit.rest.actuator.ConsumerActuator
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
* @see java.lang.SuppressWarnings
* @see io.github.nichetoolkit.rest.RestException
*/
@SuppressWarnings("Duplicates")
static String providingOfEntity(ProviderContext providerContext, @Nullable String tablename, E entityParameter, ConsumerActuator tableOptional, MybatisSqlSupply.SimpleSqlSupply sqlSupply) throws RestException {
Object entity = reviseParameter(entityParameter);
return MybatisSqlScript.caching(providerContext, (table, sqlScript) -> {
tableOptional.actuate(table);
SqlBuilder sqlBuilder = new SqlBuilder();
List uniqueColumns = table.uniqueColumns();
String entitySql = table.uniqueColumns().stream()
.map(column -> column.columnEqualsProperty(EntityConstants.ENTITY_PREFIX))
.collect(Collectors.joining(SQLConstants.BLANK + SQLConstants.OR + SQLConstants.BLANK));
if (uniqueColumns.size() == 1) {
sqlBuilder.append(entitySql);
} else {
sqlBuilder.braceLt().append(entitySql).braceGt();
}
Optional.ofNullable(table.getLogicColumn()).ifPresent(column -> sqlBuilder.and().append(column.columnEqualsProperty()));
return sqlSupply.supply(tablename, table, sqlBuilder);
});
}
/**
* providingOfEntity
* The providing of entity method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param entityParameter E The entity parameter parameter is E
type.
* @param idParameter I The id parameter parameter is I
type.
* @param tableOptional {@link io.github.nichetoolkit.rest.actuator.ConsumerActuator} The table optional parameter is ConsumerActuator
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The sql supply parameter is SimpleSqlSupply
type.
* @return {@link java.lang.String} The providing of entity return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see io.github.nichetoolkit.rest.actuator.ConsumerActuator
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
* @see java.lang.SuppressWarnings
* @see io.github.nichetoolkit.rest.RestException
*/
@SuppressWarnings("Duplicates")
static String providingOfEntity(ProviderContext providerContext, @Nullable String tablename, E entityParameter, I idParameter, ConsumerActuator tableOptional, MybatisSqlSupply.SimpleSqlSupply sqlSupply) throws RestException {
Object identity = reviseParameter(idParameter);
return MybatisSqlScript.caching(providerContext, (table, sqlScript) -> {
tableOptional.actuate(table);
SqlBuilder sqlBuilder = new SqlBuilder();
if (table.isSpecialIdentity()) {
valueOfParameter(table.identityColumns(), identity, table.getIdentityType());
String identitySql = sqlOfColumns(identity, table.identityColumns(), true, false);
sqlBuilder.append(identitySql);
} else {
Optional.ofNullable(table.getIdentityColumn()).ifPresent(column -> sqlBuilder.append(column.columnEqualsProperty()));
}
List uniqueColumns = table.uniqueColumns();
String entitySql = table.uniqueColumns().stream()
.map(column -> column.columnEqualsProperty(EntityConstants.ENTITY_PREFIX))
.collect(Collectors.joining(SQLConstants.BLANK + SQLConstants.OR + SQLConstants.BLANK));
if (uniqueColumns.size() == 1) {
sqlBuilder.and().append(entitySql);
} else {
sqlBuilder.and().braceLt().append(entitySql).braceGt();
}
Optional.ofNullable(table.getLogicColumn()).ifPresent(column -> sqlBuilder.and().append(column.columnEqualsProperty()));
return sqlSupply.supply(tablename, table, sqlBuilder);
});
}
/**
* providingOfSave
* The providing of save method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param entityParameter E The entity parameter parameter is E
type.
* @param tableOptional {@link io.github.nichetoolkit.rest.actuator.ConsumerActuator} The table optional parameter is ConsumerActuator
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The sql supply parameter is SimpleSqlSupply
type.
* @return {@link java.lang.String} The providing of save return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see io.github.nichetoolkit.rest.actuator.ConsumerActuator
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
* @see java.lang.SuppressWarnings
* @see io.github.nichetoolkit.rest.RestException
*/
@SuppressWarnings("Duplicates")
static String providingOfSave(ProviderContext providerContext, @Nullable String tablename, E entityParameter, ConsumerActuator tableOptional, MybatisSqlSupply.SimpleSqlSupply sqlSupply) throws RestException {
return MybatisSqlScript.caching(providerContext, (table, sqlScript) -> {
tableOptional.actuate(table);
String sqlOfInsert = table.insertColumns().stream()
.map(column -> column.variable(EntityConstants.ENTITY_PREFIX))
.collect(Collectors.joining(SQLConstants.COMMA + SQLConstants.BLANK));
SqlBuilder sqlBuilder = SqlBuilder.sqlBuilder().braceLt().append(sqlOfInsert).braceGt().append(insertOfSaveSql(tablename, table));
return sqlSupply.supply(tablename, table, sqlBuilder);
});
}
/**
* providingOfAllSave
* The providing of all save method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param entityList {@link java.util.Collection} The entity list parameter is Collection
type.
* @param tableOptional {@link io.github.nichetoolkit.rest.actuator.ConsumerActuator} The table optional parameter is ConsumerActuator
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The sql supply parameter is SimpleSqlSupply
type.
* @return {@link java.lang.String} The providing of all save return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see java.util.Collection
* @see io.github.nichetoolkit.rest.actuator.ConsumerActuator
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
* @see io.github.nichetoolkit.rest.RestException
*/
static String providingOfAllSave(ProviderContext providerContext, @Nullable String tablename, Collection entityList, ConsumerActuator tableOptional, MybatisSqlSupply.SimpleSqlSupply sqlSupply) throws RestException {
return MybatisSqlScript.caching(providerContext, (table, sqlScript) -> {
tableOptional.actuate(table);
String foreachValues = sqlScript.foreach(EntityConstants.ENTITY_LIST, EntityConstants.ENTITY, SQLConstants.COMMA + SQLConstants.BLANK, () ->
SQLConstants.BRACE_LT + table.insertColumns().stream().map(column -> column.variable(EntityConstants.ENTITY_PREFIX))
.collect(Collectors.joining(SQLConstants.COMMA + SQLConstants.BLANK)) + SQLConstants.BRACE_GT);
SqlBuilder sqlBuilder = SqlBuilder.sqlBuilder(foreachValues).append(insertOfSaveSql(tablename, table));
return sqlSupply.supply(tablename, table, sqlBuilder);
});
}
/**
* providingOfId
* The providing of id method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param idParameter I The id parameter parameter is I
type.
* @param tableOptional {@link io.github.nichetoolkit.rest.actuator.ConsumerActuator} The table optional parameter is ConsumerActuator
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The sql supply parameter is SimpleSqlSupply
type.
* @return {@link java.lang.String} The providing of id return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see io.github.nichetoolkit.rest.actuator.ConsumerActuator
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
* @see java.lang.SuppressWarnings
* @see io.github.nichetoolkit.rest.RestException
*/
@SuppressWarnings("Duplicates")
static String providingOfId(ProviderContext providerContext, @Nullable String tablename, I idParameter, ConsumerActuator tableOptional, MybatisSqlSupply.SimpleSqlSupply sqlSupply) throws RestException {
Object identity = reviseParameter(idParameter);
return MybatisSqlScript.caching(providerContext, (table, sqlScript) -> {
tableOptional.actuate(table);
SqlBuilder sqlBuilder = SqlBuilder.sqlBuilder();
if (table.isSpecialIdentity()) {
valueOfParameter(table.identityColumns(), identity, table.getIdentityType());
String identitySql = sqlOfColumns(identity, table.identityColumns(), true, true);
sqlBuilder.append(identitySql);
} else {
Optional.ofNullable(table.getIdentityColumn()).ifPresent(column -> sqlBuilder.append(column.columnEqualsProperty()));
}
return sqlSupply.supply(tablename, table, sqlBuilder);
});
}
/**
* providingOfAll
* The providing of all method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param idList {@link java.util.Collection} The id list parameter is Collection
type.
* @param tableOptional {@link io.github.nichetoolkit.rest.actuator.ConsumerActuator} The table optional parameter is ConsumerActuator
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The sql supply parameter is SimpleSqlSupply
type.
* @return {@link java.lang.String} The providing of all return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see java.util.Collection
* @see io.github.nichetoolkit.rest.actuator.ConsumerActuator
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
* @see io.github.nichetoolkit.rest.RestException
*/
static String providingOfAll(ProviderContext providerContext, @Nullable String tablename, Collection idList, ConsumerActuator tableOptional, MybatisSqlSupply.SimpleSqlSupply sqlSupply) throws RestException {
return MybatisSqlScript.caching(providerContext, (table, sqlScript) -> {
tableOptional.actuate(table);
SqlBuilder sqlBuilder = SqlBuilder.sqlBuilder();
if (table.isSpecialIdentity()) {
RestStream.stream(idList).forEach(id -> valueOfParameter(table.identityColumns(), id, table.getIdentityType()));
Map> sliceOfColumnsMap = sliceOfColumns(table.identityColumns(), idList);
sqlBuilder.append(sqlOfColumns(sliceOfColumnsMap, table.identityColumns(), sqlScript));
} else {
sqlBuilder.append(table.getIdentityColumn().columnName()).in().braceLt();
idList.forEach(id -> sqlBuilder.value(id).comma());
sqlBuilder.delete(sqlBuilder.length() - 2).braceGt();
}
return sqlSupply.supply(tablename, table, sqlBuilder);
});
}
/**
* providingOfWhere
* The providing of where method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param whereSqlParameter {@link java.lang.String} The where sql parameter parameter is String
type.
* @param tableOptional {@link io.github.nichetoolkit.rest.actuator.ConsumerActuator} The table optional parameter is ConsumerActuator
type.
* @param sqlSupply {@link io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply} The sql supply parameter is SimpleSqlSupply
type.
* @return {@link java.lang.String} The providing of where return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see io.github.nichetoolkit.rest.actuator.ConsumerActuator
* @see io.github.nichetoolkit.mybatis.MybatisSqlSupply.SimpleSqlSupply
* @see io.github.nichetoolkit.rest.RestException
*/
static String providingOfWhere(ProviderContext providerContext, @Nullable String tablename, String whereSqlParameter, ConsumerActuator tableOptional, MybatisSqlSupply.SimpleSqlSupply sqlSupply) throws RestException {
return MybatisSqlScript.caching(providerContext, (table, sqlScript) -> {
tableOptional.actuate(table);
String whereSql = whereSqlParameter;
if (whereSql.startsWith(SQLConstants.AND_MATCH)) {
whereSql = whereSql.substring(SQLConstants.AND_MATCH.length());
}
SqlBuilder sqlBuilder = SqlBuilder.sqlBuilder();
sqlBuilder.cdataLt().append(whereSql).cdataGt();
return sqlSupply.supply(tablename, table, sqlBuilder);
});
}
/**
* valueOfParameter
* The value of parameter method.
* @param mybatisColumns {@link java.util.Collection} The mybatis columns parameter is Collection
type.
* @param parameter {@link java.lang.Object} The parameter parameter is Object
type.
* @param parameterType {@link java.lang.Class} The parameter type parameter is Class
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.util.Collection
* @see java.lang.Object
* @see java.lang.Class
* @see io.github.nichetoolkit.rest.RestException
*/
static void valueOfParameter(Collection mybatisColumns, Object parameter, Class> parameterType) throws RestException {
Boolean isPresent = parameter.getClass() == parameterType;
OptionalUtils.ofFalse(isPresent, "The type of parameter is not " + parameterType.getName(), parameterType.getName(), MybatisParamErrorException::new);
Boolean logicalOr = RestStream.stream(mybatisColumns).map(MybatisColumn::getField)
.map(mybatisField -> GeneralUtils.isNotEmpty(mybatisField.get(parameter)))
.collect(RestCollectors.logicalOr());
OptionalUtils.ofFalse(logicalOr, "The field values of parameter can not all be empty, " + parameterType.getName(), MybatisParamErrorException::new);
}
/**
* sliceOfColumns
* The slice of columns method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param mybatisColumns {@link java.util.Collection} The mybatis columns parameter is Collection
type.
* @param idList {@link java.util.Collection} The id list parameter is Collection
type.
* @return {@link java.util.Map} The slice of columns return object is Map
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.util.Collection
* @see java.util.Map
* @see io.github.nichetoolkit.rest.RestException
*/
static Map> sliceOfColumns(Collection mybatisColumns, Collection idList) throws RestException {
List mybatisFields = RestStream.stream(mybatisColumns).map(MybatisColumn::getField).collect(RestCollectors.toList());
/*
* mybatisFields: {a,b,c}, index: 0,1,2 indexValue: 1,2,4
* 0: {}, 1: {a}, 2: {b}, 3: {a,b}, 4: {c} 5: {a,c}, 6: {b,c}, 7: {a,b,c}
*/
return RestStream.stream(idList).collect(RestCollectors.groupingBy(id -> {
int indexValue = 0;
for (int index = 0; index < mybatisFields.size(); index++) {
MybatisField mybatisField = mybatisFields.get(index);
if (GeneralUtils.isValid(mybatisField.get(id))) {
indexValue = indexValue | -(-1 << index);
}
}
return indexValue;
}));
}
/**
* sqlOfColumns
* The sql of columns method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param parameter I The parameter parameter is I
type.
* @param mybatisColumns {@link java.util.Collection} The mybatis columns parameter is Collection
type.
* @param andOrComma boolean The and or comma parameter is boolean
type.
* @param isEquals boolean The is equals parameter is boolean
type.
* @return {@link java.lang.String} The sql of columns return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.util.Collection
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
static String sqlOfColumns(I parameter, Collection mybatisColumns, boolean andOrComma, boolean isEquals) throws RestException {
SqlBuilder sqlBuilder = new SqlBuilder();
boolean isNotFirstValue = false;
for (MybatisColumn mybatisColumn : mybatisColumns) {
String columnName = mybatisColumn.columnName();
MybatisField mybatisField = mybatisColumn.getField();
Object fieldValue = mybatisField.get(parameter);
if (GeneralUtils.isNotEmpty(fieldValue)) {
if (RestKey.class.isAssignableFrom(fieldValue.getClass())) {
fieldValue = ((RestKey>) fieldValue).getKey();
}
if (isNotFirstValue) {
if (andOrComma) {
sqlBuilder.and();
} else {
sqlBuilder.comma();
}
} else {
isNotFirstValue = true;
}
sqlBuilder.append(columnName);
if (isEquals) {
sqlBuilder.eq();
} else {
sqlBuilder.neq();
}
sqlBuilder.value(fieldValue);
}
}
sqlBuilder.deleteLastChar();
return sqlBuilder.toString();
}
/**
* sqlOfColumns
* The sql of columns method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param columnsSliceMap {@link java.util.Map} The columns slice map parameter is Map
type.
* @param columns {@link java.util.List} The columns parameter is List
type.
* @param sqlScript {@link io.github.nichetoolkit.mybatis.MybatisSqlScript} The sql script parameter is MybatisSqlScript
type.
* @return {@link java.lang.String} The sql of columns return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.util.Map
* @see java.util.List
* @see io.github.nichetoolkit.mybatis.MybatisSqlScript
* @see java.lang.String
* @see java.lang.SuppressWarnings
* @see io.github.nichetoolkit.rest.RestException
*/
/*
* mybatisFields: {a,b,c}, index: 0,1,2 indexValue: 1,2,4
* 0: {}, 1: {a}, 2: {b}, 3: {a,b}, 4: {c} 5: {a,c}, 6: {b,c}, 7: {a,b,c}
* SELECT template_pk1, template_pk2, name, description, time, update_time, create_time, logic_sign
* FROM ntr_template WHERE 1=1 AND ((template_pk1) IN (('1' )) OR (template_pk2) IN (('3' )))
*/
@SuppressWarnings("Duplicates")
static String sqlOfColumns(Map> columnsSliceMap, List columns, MybatisSqlScript sqlScript) throws RestException {
if (GeneralUtils.isEmpty(columnsSliceMap)) {
return SqlBuilder.EMPTY;
}
SqlBuilder sqlBuilder = new SqlBuilder(SQLConstants.BRACE_LT);
for (Map.Entry> entry : columnsSliceMap.entrySet()) {
Integer key = entry.getKey();
List valueList = entry.getValue();
if (GeneralUtils.isNotEmpty(key) && GeneralUtils.isNotEmpty(valueList)) {
List indices = RestReckon.denexNumber(key);
if (GeneralUtils.isNotEmpty(indices)) {
List mybatisColumns = RestStream.stream(indices)
.map(index -> columns.get(index.intValue())).collect(RestCollectors.toList());
boolean isMultiColumns = mybatisColumns.size() > 1;
boolean isSingleValue = valueList.size() == 1;
if (isSingleValue) {
if (isMultiColumns) {
sqlBuilder.braceLt();
}
boolean isNotFirst = false;
I value = valueList.get(0);
for (MybatisColumn mybatisColumn : mybatisColumns) {
MybatisField mybatisField = mybatisColumn.getField();
Object fieldValue = mybatisField.get(value);
sqlBuilder.eq(mybatisColumn.columnName(), fieldValue, isNotFirst ? true : null);
isNotFirst = true;
}
if (isMultiColumns) {
sqlBuilder.braceGt();
}
} else {
String fieldSql = RestStream.stream(mybatisColumns).map(MybatisColumn::columnName).collect(RestCollectors.joining(SQLConstants.COMMA));
if (isMultiColumns) {
sqlBuilder.braceLt().append(fieldSql).braceGt().in().braceLt();
} else {
sqlBuilder.append(fieldSql).in().braceLt();
}
RestStream.stream(valueList).forEach(value -> {
if (isMultiColumns) {
sqlBuilder.braceLt();
}
RestStream.stream(mybatisColumns).map(MybatisColumn::getField).forEach(field -> {
Object indexValue = field.get(value);
sqlBuilder.value(indexValue).comma();
});
sqlBuilder.deleteLastChar();
if (isMultiColumns) {
sqlBuilder.braceGt().comma();
} else {
sqlBuilder.comma();
}
});
sqlBuilder.deleteLastChar().braceGt();
}
}
sqlBuilder.or();
}
}
sqlBuilder.delete(sqlBuilder.length() - 4).braceGt();
return sqlBuilder.toString();
}
/**
* insertOfSaveSql
* The insert of save sql method.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param table {@link io.github.nichetoolkit.mybatis.MybatisTable} The table parameter is MybatisTable
type.
* @return {@link java.lang.String} The insert of save sql return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see java.lang.String
* @see org.springframework.lang.Nullable
* @see io.github.nichetoolkit.mybatis.MybatisTable
* @see io.github.nichetoolkit.rest.RestException
*/
static String insertOfSaveSql(@Nullable String tablename, MybatisTable table) throws RestException {
SqlBuilder sqlBuilder = SqlBuilder.sqlBuilder();
RestOptional> optionalColumns = RestOptional.ofEmptyable(table.updateColumns());
sqlBuilder.onConflict().braceLt();
if (table.isSpecialIdentity()) {
sqlBuilder.append(table.sqlOfIdentityColumns());
} else {
sqlBuilder.append(table.getIdentityColumn().columnName());
}
sqlBuilder.braceGt();
if (optionalColumns.isPresent()) {
sqlBuilder.doUpdate();
} else {
sqlBuilder.doNothing();
}
optionalColumns.ifEmptyPresent(updateColumns -> {
String collect = RestStream.stream(updateColumns).map(column -> column.excluded(table.tablename(tablename)))
.collect(RestCollectors.joining(SQLConstants.COMMA + SQLConstants.BLANK + SQLConstants.LINEFEED));
sqlBuilder.set().append(collect);
});
return sqlBuilder.toString();
}
}