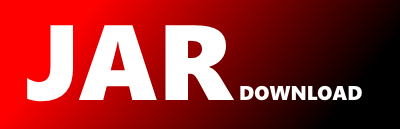
io.github.nichetoolkit.mybatis.provider.PostgresAlertLinkProvider Maven / Gradle / Ivy
Show all versions of mybatis-toolkit-starter Show documentation
package io.github.nichetoolkit.mybatis.provider;
import io.github.nichetoolkit.mybatis.MybatisSqlProvider;
import io.github.nichetoolkit.mybatis.enums.DatabaseType;
import io.github.nichetoolkit.mybatis.error.MybatisParamErrorException;
import io.github.nichetoolkit.rest.RestException;
import io.github.nichetoolkit.rest.util.OptionalUtils;
import lombok.extern.slf4j.Slf4j;
import org.apache.ibatis.builder.annotation.ProviderContext;
import org.springframework.stereotype.Component;
import java.util.Collection;
/**
* PostgresAlertLinkProvider
* The postgres alert link provider class.
* @author Cyan ([email protected])
* @see io.github.nichetoolkit.mybatis.MybatisSqlProvider
* @see lombok.extern.slf4j.Slf4j
* @see org.springframework.stereotype.Component
* @since Jdk1.8
*/
@Slf4j
@Component
public class PostgresAlertLinkProvider implements MybatisSqlProvider {
@Override
public DatabaseType databaseType() {
return DatabaseType.POSTGRESQL;
}
/**
* alertByLinkId
* The alert by link id method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param linkId L The link id parameter is L
type.
* @param status S The status parameter is S
type.
* @return {@link java.lang.String} The alert by link id return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String alertByLinkId(ProviderContext providerContext, L linkId, S status) throws RestException {
return alertDynamicByLinkId(providerContext, null, linkId, status);
}
/**
* alertDynamicByLinkId
* The alert dynamic by link id method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param linkId L The link id parameter is L
type.
* @param status S The status parameter is S
type.
* @return {@link java.lang.String} The alert dynamic by link id return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String alertDynamicByLinkId(ProviderContext providerContext, String tablename, L linkId, S status) throws RestException {
OptionalUtils.ofEmpty(linkId, "The link id param of 'alertByLinkId' method cannot be empty!", message -> new MybatisParamErrorException("alertByLinkId", "linkId", message));
OptionalUtils.ofEmpty(status, "The status param of 'alertByLinkId' method cannot be empty!", message -> new MybatisParamErrorException("alertByLinkId", "status", message));
return MybatisSqlProvider.providingOfLinkId(providerContext, tablename, linkId, status, ALERT_SQL_SUPPLY);
}
/**
* alertAllByLinkIds
* The alert all by link ids method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param linkIdList {@link java.util.Collection} The link id list parameter is Collection
type.
* @param status S The status parameter is S
type.
* @return {@link java.lang.String} The alert all by link ids return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.util.Collection
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String alertAllByLinkIds(ProviderContext providerContext, Collection linkIdList, S status) throws RestException {
return alertDynamicAllByLinkIds(providerContext, null, linkIdList, status);
}
/**
* alertDynamicAllByLinkIds
* The alert dynamic all by link ids method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param linkIdList {@link java.util.Collection} The link id list parameter is Collection
type.
* @param status S The status parameter is S
type.
* @return {@link java.lang.String} The alert dynamic all by link ids return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see java.util.Collection
* @see io.github.nichetoolkit.rest.RestException
*/
public static String alertDynamicAllByLinkIds(ProviderContext providerContext, String tablename, Collection linkIdList, S status) throws RestException {
OptionalUtils.ofEmpty(linkIdList, "The link id list param of 'alertAllByLinkIds' method cannot be empty!", message -> new MybatisParamErrorException("alertAllByLinkIds", "linkIdList", message));
OptionalUtils.ofEmpty(status, "The status param of 'alertAllByLinkIds' method cannot be empty!", message -> new MybatisParamErrorException("alertAllByLinkIds", "status", message));
return MybatisSqlProvider.providingOfLinkIdAll(providerContext, tablename, linkIdList, status, ALERT_SQL_SUPPLY);
}
}