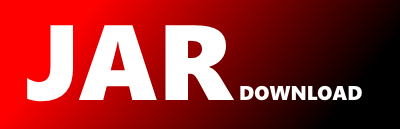
io.github.nichetoolkit.mybatis.provider.PostgresAlertProvider Maven / Gradle / Ivy
Show all versions of mybatis-toolkit-starter Show documentation
package io.github.nichetoolkit.mybatis.provider;
import io.github.nichetoolkit.mybatis.MybatisSqlProvider;
import io.github.nichetoolkit.mybatis.enums.DatabaseType;
import io.github.nichetoolkit.mybatis.error.MybatisParamErrorException;
import io.github.nichetoolkit.rest.RestException;
import io.github.nichetoolkit.rest.util.OptionalUtils;
import lombok.extern.slf4j.Slf4j;
import org.apache.ibatis.builder.annotation.ProviderContext;
import org.springframework.stereotype.Component;
import java.util.Collection;
/**
* PostgresAlertProvider
* The postgres alert provider class.
* @author Cyan ([email protected])
* @see io.github.nichetoolkit.mybatis.MybatisSqlProvider
* @see lombok.extern.slf4j.Slf4j
* @see org.springframework.stereotype.Component
* @since Jdk1.8
*/
@Slf4j
@Component
public class PostgresAlertProvider implements MybatisSqlProvider {
@Override
public DatabaseType databaseType() {
return DatabaseType.POSTGRESQL;
}
/**
* alertById
* The alert by id method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param id I The id parameter is I
type.
* @param status S The status parameter is S
type.
* @return {@link java.lang.String} The alert by id return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String alertById(ProviderContext providerContext, I id, S status) throws RestException {
return alertDynamicById(providerContext, null, id, status);
}
/**
* alertDynamicById
* The alert dynamic by id method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param id I The id parameter is I
type.
* @param status S The status parameter is S
type.
* @return {@link java.lang.String} The alert dynamic by id return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String alertDynamicById(ProviderContext providerContext, String tablename, I id, S status) throws RestException {
OptionalUtils.ofEmpty(id, "The id param of 'alertById' method cannot be empty!", message -> new MybatisParamErrorException("alertById", "id", message));
OptionalUtils.ofEmpty(status, "The status param of 'alertById' method cannot be empty!", message -> new MybatisParamErrorException("alertById", "status", message));
return MybatisSqlProvider.providingOfId(providerContext, tablename, id, status, ALERT_SQL_SUPPLY);
}
/**
* alertAll
* The alert all method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param idList {@link java.util.Collection} The id list parameter is Collection
type.
* @param status S The status parameter is S
type.
* @return {@link java.lang.String} The alert all return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.util.Collection
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String alertAll(ProviderContext providerContext, Collection idList, S status) throws RestException {
return alertDynamicAll(providerContext, null, idList, status);
}
/**
* alertDynamicAll
* The alert dynamic all method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param idList {@link java.util.Collection} The id list parameter is Collection
type.
* @param status S The status parameter is S
type.
* @return {@link java.lang.String} The alert dynamic all return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see java.util.Collection
* @see io.github.nichetoolkit.rest.RestException
*/
public static String alertDynamicAll(ProviderContext providerContext, String tablename, Collection idList, S status) throws RestException {
OptionalUtils.ofEmpty(idList, "The id list param of 'alertAll' method cannot be empty!", message -> new MybatisParamErrorException("alertAll", "idList", message));
OptionalUtils.ofEmpty(status, "The status param of 'alertAll' method cannot be empty!", message -> new MybatisParamErrorException("alertAll", "status", message));
return MybatisSqlProvider.providingOfAll(providerContext, tablename, idList, status, ALERT_SQL_SUPPLY);
}
/**
* alertAllByWhere
* The alert all by where method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param whereSql {@link java.lang.String} The where sql parameter is String
type.
* @param status S The status parameter is S
type.
* @return {@link java.lang.String} The alert all by where return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String alertAllByWhere(ProviderContext providerContext, String whereSql, S status) throws RestException {
return alertDynamicAllByWhere(providerContext, null, whereSql, status);
}
/**
* alertDynamicAllByWhere
* The alert dynamic all by where method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param whereSql {@link java.lang.String} The where sql parameter is String
type.
* @param status S The status parameter is S
type.
* @return {@link java.lang.String} The alert dynamic all by where return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String alertDynamicAllByWhere(ProviderContext providerContext, String tablename, String whereSql, S status) throws RestException {
OptionalUtils.ofEmpty(whereSql, "The where sql param of 'alertAllByWhere' method cannot be empty!", message -> new MybatisParamErrorException("alertAllByWhere", "whereSql", message));
OptionalUtils.ofEmpty(status, "The status param of 'alertAllByWhere' method cannot be empty!", message -> new MybatisParamErrorException("alertAllByWhere", "status", message));
return MybatisSqlProvider.providingOfWhere(providerContext, tablename, whereSql, status, ALERT_SQL_SUPPLY);
}
}