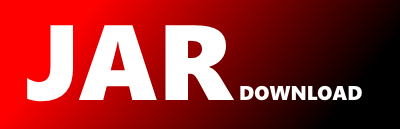
io.github.nichetoolkit.mybatis.provider.PostgresOperateProvider Maven / Gradle / Ivy
Show all versions of mybatis-toolkit-starter Show documentation
package io.github.nichetoolkit.mybatis.provider;
import io.github.nichetoolkit.mybatis.MybatisSqlProvider;
import io.github.nichetoolkit.mybatis.MybatisTable;
import io.github.nichetoolkit.mybatis.enums.DatabaseType;
import io.github.nichetoolkit.mybatis.error.MybatisParamErrorException;
import io.github.nichetoolkit.mybatis.error.MybatisTableErrorException;
import io.github.nichetoolkit.rest.RestException;
import io.github.nichetoolkit.rest.actuator.ConsumerActuator;
import io.github.nichetoolkit.rest.util.OptionalUtils;
import lombok.extern.slf4j.Slf4j;
import org.apache.ibatis.builder.annotation.ProviderContext;
import org.springframework.stereotype.Component;
import java.util.Collection;
/**
* PostgresOperateProvider
* The postgres operate provider class.
* @author Cyan ([email protected])
* @see io.github.nichetoolkit.mybatis.MybatisSqlProvider
* @see lombok.extern.slf4j.Slf4j
* @see org.springframework.stereotype.Component
* @since Jdk1.8
*/
@Slf4j
@Component
public class PostgresOperateProvider implements MybatisSqlProvider {
@Override
public DatabaseType databaseType() {
return DatabaseType.POSTGRESQL;
}
/**
* operateById
* The operate by id method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param id I The id parameter is I
type.
* @param operate {@link java.lang.Integer} The operate parameter is Integer
type.
* @return {@link java.lang.String} The operate by id return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.Integer
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String operateById(ProviderContext providerContext, I id, Integer operate) throws RestException {
return operateDynamicById(providerContext, null, id, operate);
}
/**
* operateDynamicById
* The operate dynamic by id method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param id I The id parameter is I
type.
* @param operate {@link java.lang.Integer} The operate parameter is Integer
type.
* @return {@link java.lang.String} The operate dynamic by id return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see java.lang.Integer
* @see io.github.nichetoolkit.rest.RestException
*/
public static String operateDynamicById(ProviderContext providerContext, String tablename, I id, Integer operate) throws RestException {
OptionalUtils.ofEmpty(id, "the id param of 'operateById' method cannot be empty!", message -> new MybatisTableErrorException("operateById", "id", message));
OptionalUtils.ofEmpty(operate, "the operate param of 'operateById' method cannot be empty!", message -> new MybatisParamErrorException("operateById", "operate", message));
String operateColumn = "The operate column of table with 'operateById' method cannot be empty!";
ConsumerActuator tableOptional = table ->
OptionalUtils.ofEmpty(table.getOperateColumn(), operateColumn, log, message -> new MybatisTableErrorException("operateById", "operateColumn", message));
return MybatisSqlProvider.providingOfId(providerContext, tablename, id, tableOptional, OPERATE_SQL_SUPPLY);
}
/**
* operateAll
* The operate all method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param idList {@link java.util.Collection} The id list parameter is Collection
type.
* @param operate {@link java.lang.Integer} The operate parameter is Integer
type.
* @return {@link java.lang.String} The operate all return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.util.Collection
* @see java.lang.Integer
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String operateAll(ProviderContext providerContext, Collection idList, Integer operate) throws RestException {
return operateDynamicAll(providerContext, null, idList, operate);
}
/**
* operateDynamicAll
* The operate dynamic all method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param idList {@link java.util.Collection} The id list parameter is Collection
type.
* @param operate {@link java.lang.Integer} The operate parameter is Integer
type.
* @return {@link java.lang.String} The operate dynamic all return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see java.util.Collection
* @see java.lang.Integer
* @see io.github.nichetoolkit.rest.RestException
*/
public static String operateDynamicAll(ProviderContext providerContext, String tablename, Collection idList, Integer operate) throws RestException {
OptionalUtils.ofEmpty(idList, "The id list param of 'operateAll' method cannot be empty!", message -> new MybatisParamErrorException("operateAll", "idList", message));
OptionalUtils.ofEmpty(operate, "The operate param of 'operateAll' method cannot be empty!", message -> new MybatisParamErrorException("operateAll", "operate", message));
String operateColumn = "The operate column of table with 'operateAll' method cannot be empty!";
ConsumerActuator tableOptional = table ->
OptionalUtils.ofEmpty(table.getOperateColumn(), operateColumn, log, message -> new MybatisTableErrorException("operateAll", "operateColumn", message));
return MybatisSqlProvider.providingOfAll(providerContext, tablename, idList, tableOptional, OPERATE_SQL_SUPPLY);
}
/**
* operateAllByWhere
* The operate all by where method.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param whereSql {@link java.lang.String} The where sql parameter is String
type.
* @param operate {@link java.lang.Integer} The operate parameter is Integer
type.
* @return {@link java.lang.String} The operate all by where return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see java.lang.Integer
* @see io.github.nichetoolkit.rest.RestException
*/
public static String operateAllByWhere(ProviderContext providerContext, String whereSql, Integer operate) throws RestException {
return operateDynamicAllByWhere(providerContext, null, whereSql, operate);
}
/**
* operateDynamicAllByWhere
* The operate dynamic all by where method.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param whereSql {@link java.lang.String} The where sql parameter is String
type.
* @param operate {@link java.lang.Integer} The operate parameter is Integer
type.
* @return {@link java.lang.String} The operate dynamic all by where return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see java.lang.Integer
* @see io.github.nichetoolkit.rest.RestException
*/
public static String operateDynamicAllByWhere(ProviderContext providerContext, String tablename, String whereSql, Integer operate) throws RestException {
OptionalUtils.ofEmpty(whereSql, "The where sql param of 'operateAllByWhere' method cannot be empty!", message -> new MybatisParamErrorException("operateAllByWhere", "whereSql", message));
OptionalUtils.ofEmpty(operate, "The operate param of 'operateAllByWhere' method cannot be empty!", message -> new MybatisParamErrorException("operateAllByWhere", "operate", message));
String operateColumn = "The operate column of table with 'operateAllByWhere' method cannot be empty!";
ConsumerActuator tableOptional = table ->
OptionalUtils.ofEmpty(table.getOperateColumn(), operateColumn, log, message -> new MybatisTableErrorException("operateAllByWhere", "operateColumn", message));
return MybatisSqlProvider.providingOfWhere(providerContext, tablename, whereSql, tableOptional, OPERATE_SQL_SUPPLY);
}
}