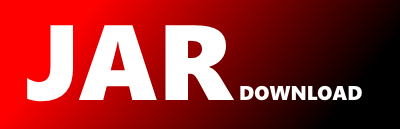
io.github.nichetoolkit.mybatis.provider.PostgresRemoveProvider Maven / Gradle / Ivy
Show all versions of mybatis-toolkit-starter Show documentation
package io.github.nichetoolkit.mybatis.provider;
import io.github.nichetoolkit.mybatis.MybatisSqlProvider;
import io.github.nichetoolkit.mybatis.MybatisTable;
import io.github.nichetoolkit.mybatis.enums.DatabaseType;
import io.github.nichetoolkit.mybatis.error.MybatisParamErrorException;
import io.github.nichetoolkit.mybatis.error.MybatisTableErrorException;
import io.github.nichetoolkit.rest.RestException;
import io.github.nichetoolkit.rest.actuator.ConsumerActuator;
import io.github.nichetoolkit.rest.util.OptionalUtils;
import lombok.extern.slf4j.Slf4j;
import org.apache.ibatis.builder.annotation.ProviderContext;
import org.springframework.stereotype.Component;
import java.util.Collection;
/**
* PostgresRemoveProvider
* The postgres remove provider class.
* @author Cyan ([email protected])
* @see io.github.nichetoolkit.mybatis.MybatisSqlProvider
* @see lombok.extern.slf4j.Slf4j
* @see org.springframework.stereotype.Component
* @since Jdk1.8
*/
@Slf4j
@Component
public class PostgresRemoveProvider implements MybatisSqlProvider {
@Override
public DatabaseType databaseType() {
return DatabaseType.POSTGRESQL;
}
/**
* removeById
* The remove by id method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param id I The id parameter is I
type.
* @param logic {@link java.lang.Object} The logic parameter is Object
type.
* @return {@link java.lang.String} The remove by id return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.Object
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String removeById(ProviderContext providerContext, I id, Object logic) throws RestException {
return removeDynamicById(providerContext, null, id, logic);
}
/**
* removeDynamicById
* The remove dynamic by id method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param id I The id parameter is I
type.
* @param logic {@link java.lang.Object} The logic parameter is Object
type.
* @return {@link java.lang.String} The remove dynamic by id return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see java.lang.Object
* @see io.github.nichetoolkit.rest.RestException
*/
public static String removeDynamicById(ProviderContext providerContext, String tablename, I id, Object logic) throws RestException {
OptionalUtils.ofEmpty(id, "The id param of 'removeById' method cannot be empty!", message -> new MybatisTableErrorException("removeById", "id", message));
OptionalUtils.ofEmpty(logic, "The logic param of 'removeById' method cannot be empty!", message -> new MybatisParamErrorException("removeById", "logic", message));
String logicColumn = "The logic column of table with 'removeById' method cannot be empty!";
ConsumerActuator tableOptional = table ->
OptionalUtils.ofEmpty(table.getLogicColumn(), logicColumn, log, message -> new MybatisTableErrorException("removeById", "logicColumn", message));
return MybatisSqlProvider.providingOfId(providerContext, tablename, id, tableOptional, REMOVE_SQL_SUPPLY);
}
/**
* removeAll
* The remove all method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param idList {@link java.util.Collection} The id list parameter is Collection
type.
* @param logic {@link java.lang.Object} The logic parameter is Object
type.
* @return {@link java.lang.String} The remove all return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.util.Collection
* @see java.lang.Object
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String removeAll(ProviderContext providerContext, Collection idList, Object logic) throws RestException {
return removeDynamicAll(providerContext, null, idList, logic);
}
/**
* removeDynamicAll
* The remove dynamic all method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param idList {@link java.util.Collection} The id list parameter is Collection
type.
* @param logic {@link java.lang.Object} The logic parameter is Object
type.
* @return {@link java.lang.String} The remove dynamic all return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see java.util.Collection
* @see java.lang.Object
* @see io.github.nichetoolkit.rest.RestException
*/
public static String removeDynamicAll(ProviderContext providerContext, String tablename, Collection idList, Object logic) throws RestException {
OptionalUtils.ofEmpty(idList, "The id list param of 'removeAll' method cannot be empty!", message -> new MybatisParamErrorException("removeAll", "idList", message));
OptionalUtils.ofEmpty(logic, "The logic param of 'removeAll' method cannot be empty!", message -> new MybatisParamErrorException("removeAll", "logic", message));
String logicColumn = "The logic column of table with 'removeAll' method cannot be empty!";
ConsumerActuator tableOptional = table ->
OptionalUtils.ofEmpty(table.getLogicColumn(), logicColumn, log, message -> new MybatisTableErrorException("removeAll", "logicColumn", message));
return MybatisSqlProvider.providingOfAll(providerContext, tablename, idList, tableOptional, REMOVE_SQL_SUPPLY);
}
/**
* removeAllByWhere
* The remove all by where method.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param whereSql {@link java.lang.String} The where sql parameter is String
type.
* @param logic {@link java.lang.Object} The logic parameter is Object
type.
* @return {@link java.lang.String} The remove all by where return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see java.lang.Object
* @see io.github.nichetoolkit.rest.RestException
*/
public static String removeAllByWhere(ProviderContext providerContext, String whereSql,Object logic) throws RestException {
return removeDynamicAllByWhere(providerContext, null, whereSql, logic);
}
/**
* removeDynamicAllByWhere
* The remove dynamic all by where method.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param whereSql {@link java.lang.String} The where sql parameter is String
type.
* @param logic {@link java.lang.Object} The logic parameter is Object
type.
* @return {@link java.lang.String} The remove dynamic all by where return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see java.lang.Object
* @see io.github.nichetoolkit.rest.RestException
*/
public static String removeDynamicAllByWhere(ProviderContext providerContext,String tablename, String whereSql, Object logic) throws RestException {
OptionalUtils.ofEmpty(whereSql, "The where sql param of 'removeAllByWhere' method cannot be empty!", message -> new MybatisParamErrorException("removeAllByWhere", "whereSql", message));
OptionalUtils.ofEmpty(logic, "The logic param of 'removeAllByWhere' method cannot be empty!", message -> new MybatisParamErrorException("removeAllByWhere", "logic", message));
String logicColumn = "The logic column of table with 'removeAllByWhere' method cannot be empty!";
ConsumerActuator tableOptional = table ->
OptionalUtils.ofEmpty(table.getLogicColumn(), logicColumn, log, message -> new MybatisTableErrorException("removeAllByWhere", "logicColumn", message));
return MybatisSqlProvider.providingOfWhere(providerContext, tablename, whereSql, tableOptional, REMOVE_SQL_SUPPLY);
}
}