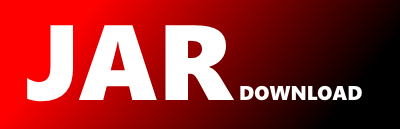
io.github.nichetoolkit.mybatis.provider.PostgresSaveProvider Maven / Gradle / Ivy
Show all versions of mybatis-toolkit-starter Show documentation
package io.github.nichetoolkit.mybatis.provider;
import io.github.nichetoolkit.mybatis.MybatisSqlProvider;
import io.github.nichetoolkit.mybatis.MybatisTable;
import io.github.nichetoolkit.mybatis.enums.DatabaseType;
import io.github.nichetoolkit.mybatis.error.MybatisParamErrorException;
import io.github.nichetoolkit.mybatis.error.MybatisTableErrorException;
import io.github.nichetoolkit.rest.RestException;
import io.github.nichetoolkit.rest.actuator.ConsumerActuator;
import io.github.nichetoolkit.rest.util.OptionalUtils;
import lombok.extern.slf4j.Slf4j;
import org.apache.ibatis.builder.annotation.ProviderContext;
import org.springframework.stereotype.Component;
import java.util.Collection;
/**
* PostgresSaveProvider
* The postgres save provider class.
* @author Cyan ([email protected])
* @see io.github.nichetoolkit.mybatis.MybatisSqlProvider
* @see lombok.extern.slf4j.Slf4j
* @see org.springframework.stereotype.Component
* @since Jdk1.8
*/
@Slf4j
@Component
public class PostgresSaveProvider implements MybatisSqlProvider {
@Override
public DatabaseType databaseType() {
return DatabaseType.POSTGRESQL;
}
/**
* save
* The save method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param entity E The entity parameter is E
type.
* @return {@link java.lang.String} The save return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String save(ProviderContext providerContext, E entity) throws RestException {
return saveDynamic(providerContext, null, entity);
}
/**
* saveDynamic
* The save dynamic method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param entity E The entity parameter is E
type.
* @return {@link java.lang.String} The save dynamic return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String saveDynamic(ProviderContext providerContext, String tablename, E entity) throws RestException {
OptionalUtils.ofEmpty(entity, "The entity param of 'save' method cannot be empty!", message -> new MybatisParamErrorException("save", "entity", message));
String insertColumns = "The insert columns of table with 'save' method cannot be empty!";
ConsumerActuator tableOptional = table -> OptionalUtils.ofEmpty(table.insertColumns(), insertColumns, log,
message -> new MybatisTableErrorException("save", "insertColumns", message));
return MybatisSqlProvider.providingOfSave(providerContext, tablename, entity, tableOptional, SAVE_SQL_SUPPLY);
}
/**
* saveAll
* The save all method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param entityList {@link java.util.Collection} The entity list parameter is Collection
type.
* @return {@link java.lang.String} The save all return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.util.Collection
* @see java.lang.String
* @see io.github.nichetoolkit.rest.RestException
*/
public static String saveAll(ProviderContext providerContext, Collection entityList) throws RestException {
return saveDynamicAll(providerContext, null, entityList);
}
/**
* saveDynamicAll
* The save dynamic all method.
* @param {@link java.lang.Object} The parameter can be of any type.
* @param providerContext {@link org.apache.ibatis.builder.annotation.ProviderContext} The provider context parameter is ProviderContext
type.
* @param tablename {@link java.lang.String} The tablename parameter is String
type.
* @param entityList {@link java.util.Collection} The entity list parameter is Collection
type.
* @return {@link java.lang.String} The save dynamic all return object is String
type.
* @throws RestException {@link io.github.nichetoolkit.rest.RestException} The rest exception is RestException
type.
* @see org.apache.ibatis.builder.annotation.ProviderContext
* @see java.lang.String
* @see java.util.Collection
* @see io.github.nichetoolkit.rest.RestException
*/
public static String saveDynamicAll(ProviderContext providerContext, String tablename, Collection entityList) throws RestException {
OptionalUtils.ofEmpty(entityList, "The entity list param of 'saveAll' method cannot be empty!", message -> new MybatisParamErrorException("saveAll", "entityList", message));
String insertColumns = "The insert columns of table with 'save' method cannot be empty!";
ConsumerActuator tableOptional = table -> OptionalUtils.ofEmpty(table.insertColumns(), insertColumns, log,
message -> new MybatisTableErrorException("saveAll", "insertColumns", message));
return MybatisSqlProvider.providingOfAllSave(providerContext, tablename, entityList, tableOptional, SAVE_SQL_SUPPLY);
}
}