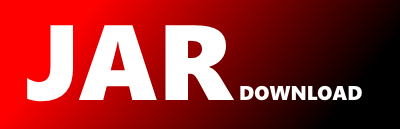
io.github.novareseller.boot.utils.JsonUtils Maven / Gradle / Ivy
package io.github.novareseller.boot.utils;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.text.SimpleDateFormat;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.TimeZone;
public class JsonUtils {
private static final ObjectMapper mapper;
private static final TypeReference> mapTypeRef = new TypeReference>() {};
static {
mapper = createDefault();
}
public static ObjectMapper createDefault() {
TimeZone tz = TimeZone.getTimeZone("UTC");
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss.SSS'Z'");
formatter.setTimeZone(tz);
ObjectMapper mapper = new ObjectMapper();
mapper.setDateFormat(formatter);
mapper.configure(JsonGenerator.Feature.QUOTE_FIELD_NAMES, true);
mapper.configure(JsonParser.Feature.ALLOW_UNQUOTED_FIELD_NAMES, true);
mapper.configure(JsonParser.Feature.ALLOW_SINGLE_QUOTES, true);
mapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
mapper.enable(DeserializationFeature.USE_BIG_DECIMAL_FOR_FLOATS);
mapper.disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
return mapper;
}
public static String json(Object object) throws Exception {
return mapper.writeValueAsString(object);
}
public static String jsonWithoutError(Object object) {
try {
return mapper.writeValueAsString(object);
} catch ( Exception ex ) {
return null;
}
}
public static T parse(String json, Class type) throws Exception {
if ( json == null || json.isEmpty() ) {
return null;
}
return mapper.readValue(json, type);
}
public static Map parseAsMap(String json) throws Exception {
if ( json == null || json.isEmpty() ) {
return null;
}
return mapper.readValue(json, mapTypeRef);
}
public static T convertBean(Map map, Class clazz) {
if ( map == null || map.isEmpty() ) {
return null;
}
return mapper.convertValue(map, clazz);
}
public static T convertBean(Map map, TypeReference typeReference) {
if ( map == null || map.isEmpty() ) {
return null;
}
return mapper.convertValue(map, typeReference);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy