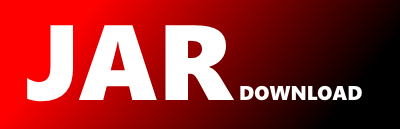
io.odysz.semantics.SemanticObject Maven / Gradle / Ivy
The newest version!
package io.odysz.semantics;
import java.io.PrintStream;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import io.odysz.anson.Anson;
import io.odysz.semantics.meta.TableMeta;
import io.odysz.transact.x.TransException;
/**
* The semantics data returned by transaction as the commitment result.
*
* @author [email protected]
*/
public class SemanticObject extends Anson {
protected HashMap props;
public HashMap props() { return props; }
/**@param prop
* @return null if the property doesn't exists
*/
public Class> getType (String prop) {
if (prop == null || props == null || !props.containsKey(prop))
return null;
Object p = props.get(prop);
return p == null
? Object.class // has key, no value
: p.getClass();
}
public boolean has(String prop) {
return props != null && props.containsKey(prop) && props.get(prop) != null;
}
public Object get(String prop) {
return props == null ? null : props.get(prop);
}
public String getString(String prop) {
return props == null ? null : (String) props.get(prop);
}
public SemanticObject data() {
return (SemanticObject) get("data");
}
public SemanticObject data(SemanticObject data) {
return put("data", data);
}
public void clear() {
props.clear();
}
public String port() {
return (String) get("port");
}
public SemanticObject code(String c) {
return put("code", c);
}
public String code() {
return (String) get("code");
}
public SemanticObject port(String port) {
return put("port", port);
}
public String msg() {
return (String) get("msg");
}
public SemanticObject msg(String msg, Object... args) {
if (args == null || args.length == 0)
return put("msg", msg);
else
return put("msg", String.format(msg, args));
}
/**Put result set (AnResultset) into "rs", which is a 3d array.
* @param resultset
* @param total
* @return this
* @throws TransException
*/
public SemanticObject rs(Object resultset, int total) throws TransException {
add("total", total);
return add("rs", resultset);
}
public Object rs(int i) {
return ((ArrayList>)get("rs")).get(i);
}
@SuppressWarnings("unchecked")
public int total(int i) {
if (get("total") == null)
return -1;
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy