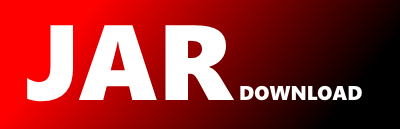
com.google.ortools.constraintsolver.ConstraintSolverParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-or-tools Show documentation
Show all versions of google-or-tools Show documentation
A project to publish Google OR-Tools as a dependable dependency in Maven.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ortools/constraint_solver/solver_parameters.proto
package com.google.ortools.constraintsolver;
/**
*
* Solver parameters.
*
*
* Protobuf type {@code operations_research.ConstraintSolverParameters}
*/
public final class ConstraintSolverParameters extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:operations_research.ConstraintSolverParameters)
ConstraintSolverParametersOrBuilder {
private static final long serialVersionUID = 0L;
// Use ConstraintSolverParameters.newBuilder() to construct.
private ConstraintSolverParameters(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConstraintSolverParameters() {
compressTrail_ = 0;
trailBlockSize_ = 0;
arraySplitSize_ = 0;
storeNames_ = false;
nameCastVariables_ = false;
nameAllVariables_ = false;
profilePropagation_ = false;
profileFile_ = "";
profileLocalSearch_ = false;
printLocalSearchProfile_ = false;
tracePropagation_ = false;
traceSearch_ = false;
printModel_ = false;
printModelStats_ = false;
printAddedConstraints_ = false;
exportFile_ = "";
disableSolve_ = false;
useCompactTable_ = false;
useSmallTable_ = false;
useSatTable_ = false;
ac4RTableThreshold_ = 0;
useMddTable_ = false;
useCumulativeEdgeFinder_ = false;
useCumulativeTimeTable_ = false;
useCumulativeTimeTableSync_ = false;
useSequenceHighDemandTasks_ = false;
useAllPossibleDisjunctions_ = false;
maxEdgeFinderSize_ = 0;
diffnUseCumulative_ = false;
useElementRmq_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConstraintSolverParameters(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
compressTrail_ = rawValue;
break;
}
case 16: {
trailBlockSize_ = input.readInt32();
break;
}
case 24: {
arraySplitSize_ = input.readInt32();
break;
}
case 32: {
storeNames_ = input.readBool();
break;
}
case 40: {
nameCastVariables_ = input.readBool();
break;
}
case 48: {
nameAllVariables_ = input.readBool();
break;
}
case 56: {
profilePropagation_ = input.readBool();
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
profileFile_ = s;
break;
}
case 72: {
tracePropagation_ = input.readBool();
break;
}
case 80: {
traceSearch_ = input.readBool();
break;
}
case 88: {
printModel_ = input.readBool();
break;
}
case 96: {
printModelStats_ = input.readBool();
break;
}
case 104: {
printAddedConstraints_ = input.readBool();
break;
}
case 114: {
java.lang.String s = input.readStringRequireUtf8();
exportFile_ = s;
break;
}
case 120: {
disableSolve_ = input.readBool();
break;
}
case 128: {
profileLocalSearch_ = input.readBool();
break;
}
case 136: {
printLocalSearchProfile_ = input.readBool();
break;
}
case 800: {
useCompactTable_ = input.readBool();
break;
}
case 808: {
useSmallTable_ = input.readBool();
break;
}
case 816: {
useSatTable_ = input.readBool();
break;
}
case 824: {
ac4RTableThreshold_ = input.readInt32();
break;
}
case 832: {
useMddTable_ = input.readBool();
break;
}
case 840: {
useCumulativeEdgeFinder_ = input.readBool();
break;
}
case 848: {
useCumulativeTimeTable_ = input.readBool();
break;
}
case 856: {
useSequenceHighDemandTasks_ = input.readBool();
break;
}
case 864: {
useAllPossibleDisjunctions_ = input.readBool();
break;
}
case 872: {
maxEdgeFinderSize_ = input.readInt32();
break;
}
case 880: {
diffnUseCumulative_ = input.readBool();
break;
}
case 888: {
useElementRmq_ = input.readBool();
break;
}
case 896: {
useCumulativeTimeTableSync_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.constraintsolver.SolverParameters.internal_static_operations_research_ConstraintSolverParameters_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.constraintsolver.SolverParameters.internal_static_operations_research_ConstraintSolverParameters_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.constraintsolver.ConstraintSolverParameters.class, com.google.ortools.constraintsolver.ConstraintSolverParameters.Builder.class);
}
/**
*
* Internal parameters of the solver.
*
*
* Protobuf enum {@code operations_research.ConstraintSolverParameters.TrailCompression}
*/
public enum TrailCompression
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NO_COMPRESSION = 0;
*/
NO_COMPRESSION(0),
/**
* COMPRESS_WITH_ZLIB = 1;
*/
COMPRESS_WITH_ZLIB(1),
UNRECOGNIZED(-1),
;
/**
* NO_COMPRESSION = 0;
*/
public static final int NO_COMPRESSION_VALUE = 0;
/**
* COMPRESS_WITH_ZLIB = 1;
*/
public static final int COMPRESS_WITH_ZLIB_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TrailCompression valueOf(int value) {
return forNumber(value);
}
public static TrailCompression forNumber(int value) {
switch (value) {
case 0: return NO_COMPRESSION;
case 1: return COMPRESS_WITH_ZLIB;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TrailCompression> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TrailCompression findValueByNumber(int number) {
return TrailCompression.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.ortools.constraintsolver.ConstraintSolverParameters.getDescriptor().getEnumTypes().get(0);
}
private static final TrailCompression[] VALUES = values();
public static TrailCompression valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private TrailCompression(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:operations_research.ConstraintSolverParameters.TrailCompression)
}
public static final int COMPRESS_TRAIL_FIELD_NUMBER = 1;
private int compressTrail_;
/**
*
* This parameter indicates if the solver should compress the trail
* during the search. No compression means that the solver will be faster,
* but will use more memory.
*
*
* .operations_research.ConstraintSolverParameters.TrailCompression compress_trail = 1;
*/
public int getCompressTrailValue() {
return compressTrail_;
}
/**
*
* This parameter indicates if the solver should compress the trail
* during the search. No compression means that the solver will be faster,
* but will use more memory.
*
*
* .operations_research.ConstraintSolverParameters.TrailCompression compress_trail = 1;
*/
public com.google.ortools.constraintsolver.ConstraintSolverParameters.TrailCompression getCompressTrail() {
com.google.ortools.constraintsolver.ConstraintSolverParameters.TrailCompression result = com.google.ortools.constraintsolver.ConstraintSolverParameters.TrailCompression.valueOf(compressTrail_);
return result == null ? com.google.ortools.constraintsolver.ConstraintSolverParameters.TrailCompression.UNRECOGNIZED : result;
}
public static final int TRAIL_BLOCK_SIZE_FIELD_NUMBER = 2;
private int trailBlockSize_;
/**
*
* This parameter indicates the default size of a block of the trail.
* Compression applies at the block level.
*
*
* int32 trail_block_size = 2;
*/
public int getTrailBlockSize() {
return trailBlockSize_;
}
public static final int ARRAY_SPLIT_SIZE_FIELD_NUMBER = 3;
private int arraySplitSize_;
/**
*
* When a sum/min/max operation is applied on a large array, this
* array is recursively split into blocks of size 'array_split_size'.
*
*
* int32 array_split_size = 3;
*/
public int getArraySplitSize() {
return arraySplitSize_;
}
public static final int STORE_NAMES_FIELD_NUMBER = 4;
private boolean storeNames_;
/**
*
* This parameters indicates if the solver should store the names of
* the objets it manages.
*
*
* bool store_names = 4;
*/
public boolean getStoreNames() {
return storeNames_;
}
public static final int NAME_CAST_VARIABLES_FIELD_NUMBER = 5;
private boolean nameCastVariables_;
/**
*
* Create names for cast variables.
*
*
* bool name_cast_variables = 5;
*/
public boolean getNameCastVariables() {
return nameCastVariables_;
}
public static final int NAME_ALL_VARIABLES_FIELD_NUMBER = 6;
private boolean nameAllVariables_;
/**
*
* Should anonymous variables be given a name.
*
*
* bool name_all_variables = 6;
*/
public boolean getNameAllVariables() {
return nameAllVariables_;
}
public static final int PROFILE_PROPAGATION_FIELD_NUMBER = 7;
private boolean profilePropagation_;
/**
*
* Activate propagation profiling.
*
*
* bool profile_propagation = 7;
*/
public boolean getProfilePropagation() {
return profilePropagation_;
}
public static final int PROFILE_FILE_FIELD_NUMBER = 8;
private volatile java.lang.Object profileFile_;
/**
*
* Export propagation profiling data to file.
*
*
* string profile_file = 8;
*/
public java.lang.String getProfileFile() {
java.lang.Object ref = profileFile_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
profileFile_ = s;
return s;
}
}
/**
*
* Export propagation profiling data to file.
*
*
* string profile_file = 8;
*/
public com.google.protobuf.ByteString
getProfileFileBytes() {
java.lang.Object ref = profileFile_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
profileFile_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROFILE_LOCAL_SEARCH_FIELD_NUMBER = 16;
private boolean profileLocalSearch_;
/**
*
* Activate local search profiling.
*
*
* bool profile_local_search = 16;
*/
public boolean getProfileLocalSearch() {
return profileLocalSearch_;
}
public static final int PRINT_LOCAL_SEARCH_PROFILE_FIELD_NUMBER = 17;
private boolean printLocalSearchProfile_;
/**
*
* Print local search profiling data after solving.
*
*
* bool print_local_search_profile = 17;
*/
public boolean getPrintLocalSearchProfile() {
return printLocalSearchProfile_;
}
public static final int TRACE_PROPAGATION_FIELD_NUMBER = 9;
private boolean tracePropagation_;
/**
*
* Activate propagate tracing.
*
*
* bool trace_propagation = 9;
*/
public boolean getTracePropagation() {
return tracePropagation_;
}
public static final int TRACE_SEARCH_FIELD_NUMBER = 10;
private boolean traceSearch_;
/**
*
* Trace search.
*
*
* bool trace_search = 10;
*/
public boolean getTraceSearch() {
return traceSearch_;
}
public static final int PRINT_MODEL_FIELD_NUMBER = 11;
private boolean printModel_;
/**
*
* Print the model before solving.
*
*
* bool print_model = 11;
*/
public boolean getPrintModel() {
return printModel_;
}
public static final int PRINT_MODEL_STATS_FIELD_NUMBER = 12;
private boolean printModelStats_;
/**
*
* Print model statistics before solving.
*
*
* bool print_model_stats = 12;
*/
public boolean getPrintModelStats() {
return printModelStats_;
}
public static final int PRINT_ADDED_CONSTRAINTS_FIELD_NUMBER = 13;
private boolean printAddedConstraints_;
/**
*
* Print added constraints.
*
*
* bool print_added_constraints = 13;
*/
public boolean getPrintAddedConstraints() {
return printAddedConstraints_;
}
public static final int EXPORT_FILE_FIELD_NUMBER = 14;
private volatile java.lang.Object exportFile_;
/**
*
* Export model to file.
*
*
* string export_file = 14;
*/
public java.lang.String getExportFile() {
java.lang.Object ref = exportFile_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
exportFile_ = s;
return s;
}
}
/**
*
* Export model to file.
*
*
* string export_file = 14;
*/
public com.google.protobuf.ByteString
getExportFileBytes() {
java.lang.Object ref = exportFile_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
exportFile_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DISABLE_SOLVE_FIELD_NUMBER = 15;
private boolean disableSolve_;
/**
* bool disable_solve = 15;
*/
public boolean getDisableSolve() {
return disableSolve_;
}
public static final int USE_COMPACT_TABLE_FIELD_NUMBER = 100;
private boolean useCompactTable_;
/**
*
* Control the implementation of the table constraint.
*
*
* bool use_compact_table = 100;
*/
public boolean getUseCompactTable() {
return useCompactTable_;
}
public static final int USE_SMALL_TABLE_FIELD_NUMBER = 101;
private boolean useSmallTable_;
/**
* bool use_small_table = 101;
*/
public boolean getUseSmallTable() {
return useSmallTable_;
}
public static final int USE_SAT_TABLE_FIELD_NUMBER = 102;
private boolean useSatTable_;
/**
* bool use_sat_table = 102;
*/
public boolean getUseSatTable() {
return useSatTable_;
}
public static final int AC4R_TABLE_THRESHOLD_FIELD_NUMBER = 103;
private int ac4RTableThreshold_;
/**
* int32 ac4r_table_threshold = 103;
*/
public int getAc4RTableThreshold() {
return ac4RTableThreshold_;
}
public static final int USE_MDD_TABLE_FIELD_NUMBER = 104;
private boolean useMddTable_;
/**
* bool use_mdd_table = 104;
*/
public boolean getUseMddTable() {
return useMddTable_;
}
public static final int USE_CUMULATIVE_EDGE_FINDER_FIELD_NUMBER = 105;
private boolean useCumulativeEdgeFinder_;
/**
*
* Control the propagation of the cumulative constraint.
*
*
* bool use_cumulative_edge_finder = 105;
*/
public boolean getUseCumulativeEdgeFinder() {
return useCumulativeEdgeFinder_;
}
public static final int USE_CUMULATIVE_TIME_TABLE_FIELD_NUMBER = 106;
private boolean useCumulativeTimeTable_;
/**
* bool use_cumulative_time_table = 106;
*/
public boolean getUseCumulativeTimeTable() {
return useCumulativeTimeTable_;
}
public static final int USE_CUMULATIVE_TIME_TABLE_SYNC_FIELD_NUMBER = 112;
private boolean useCumulativeTimeTableSync_;
/**
* bool use_cumulative_time_table_sync = 112;
*/
public boolean getUseCumulativeTimeTableSync() {
return useCumulativeTimeTableSync_;
}
public static final int USE_SEQUENCE_HIGH_DEMAND_TASKS_FIELD_NUMBER = 107;
private boolean useSequenceHighDemandTasks_;
/**
* bool use_sequence_high_demand_tasks = 107;
*/
public boolean getUseSequenceHighDemandTasks() {
return useSequenceHighDemandTasks_;
}
public static final int USE_ALL_POSSIBLE_DISJUNCTIONS_FIELD_NUMBER = 108;
private boolean useAllPossibleDisjunctions_;
/**
* bool use_all_possible_disjunctions = 108;
*/
public boolean getUseAllPossibleDisjunctions() {
return useAllPossibleDisjunctions_;
}
public static final int MAX_EDGE_FINDER_SIZE_FIELD_NUMBER = 109;
private int maxEdgeFinderSize_;
/**
* int32 max_edge_finder_size = 109;
*/
public int getMaxEdgeFinderSize() {
return maxEdgeFinderSize_;
}
public static final int DIFFN_USE_CUMULATIVE_FIELD_NUMBER = 110;
private boolean diffnUseCumulative_;
/**
*
* Control the propagation of the diffn constraint.
*
*
* bool diffn_use_cumulative = 110;
*/
public boolean getDiffnUseCumulative() {
return diffnUseCumulative_;
}
public static final int USE_ELEMENT_RMQ_FIELD_NUMBER = 111;
private boolean useElementRmq_;
/**
*
* Control the implementation of the element constraint.
*
*
* bool use_element_rmq = 111;
*/
public boolean getUseElementRmq() {
return useElementRmq_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (compressTrail_ != com.google.ortools.constraintsolver.ConstraintSolverParameters.TrailCompression.NO_COMPRESSION.getNumber()) {
output.writeEnum(1, compressTrail_);
}
if (trailBlockSize_ != 0) {
output.writeInt32(2, trailBlockSize_);
}
if (arraySplitSize_ != 0) {
output.writeInt32(3, arraySplitSize_);
}
if (storeNames_ != false) {
output.writeBool(4, storeNames_);
}
if (nameCastVariables_ != false) {
output.writeBool(5, nameCastVariables_);
}
if (nameAllVariables_ != false) {
output.writeBool(6, nameAllVariables_);
}
if (profilePropagation_ != false) {
output.writeBool(7, profilePropagation_);
}
if (!getProfileFileBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, profileFile_);
}
if (tracePropagation_ != false) {
output.writeBool(9, tracePropagation_);
}
if (traceSearch_ != false) {
output.writeBool(10, traceSearch_);
}
if (printModel_ != false) {
output.writeBool(11, printModel_);
}
if (printModelStats_ != false) {
output.writeBool(12, printModelStats_);
}
if (printAddedConstraints_ != false) {
output.writeBool(13, printAddedConstraints_);
}
if (!getExportFileBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, exportFile_);
}
if (disableSolve_ != false) {
output.writeBool(15, disableSolve_);
}
if (profileLocalSearch_ != false) {
output.writeBool(16, profileLocalSearch_);
}
if (printLocalSearchProfile_ != false) {
output.writeBool(17, printLocalSearchProfile_);
}
if (useCompactTable_ != false) {
output.writeBool(100, useCompactTable_);
}
if (useSmallTable_ != false) {
output.writeBool(101, useSmallTable_);
}
if (useSatTable_ != false) {
output.writeBool(102, useSatTable_);
}
if (ac4RTableThreshold_ != 0) {
output.writeInt32(103, ac4RTableThreshold_);
}
if (useMddTable_ != false) {
output.writeBool(104, useMddTable_);
}
if (useCumulativeEdgeFinder_ != false) {
output.writeBool(105, useCumulativeEdgeFinder_);
}
if (useCumulativeTimeTable_ != false) {
output.writeBool(106, useCumulativeTimeTable_);
}
if (useSequenceHighDemandTasks_ != false) {
output.writeBool(107, useSequenceHighDemandTasks_);
}
if (useAllPossibleDisjunctions_ != false) {
output.writeBool(108, useAllPossibleDisjunctions_);
}
if (maxEdgeFinderSize_ != 0) {
output.writeInt32(109, maxEdgeFinderSize_);
}
if (diffnUseCumulative_ != false) {
output.writeBool(110, diffnUseCumulative_);
}
if (useElementRmq_ != false) {
output.writeBool(111, useElementRmq_);
}
if (useCumulativeTimeTableSync_ != false) {
output.writeBool(112, useCumulativeTimeTableSync_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (compressTrail_ != com.google.ortools.constraintsolver.ConstraintSolverParameters.TrailCompression.NO_COMPRESSION.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, compressTrail_);
}
if (trailBlockSize_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, trailBlockSize_);
}
if (arraySplitSize_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, arraySplitSize_);
}
if (storeNames_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, storeNames_);
}
if (nameCastVariables_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, nameCastVariables_);
}
if (nameAllVariables_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, nameAllVariables_);
}
if (profilePropagation_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, profilePropagation_);
}
if (!getProfileFileBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, profileFile_);
}
if (tracePropagation_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, tracePropagation_);
}
if (traceSearch_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, traceSearch_);
}
if (printModel_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, printModel_);
}
if (printModelStats_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(12, printModelStats_);
}
if (printAddedConstraints_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, printAddedConstraints_);
}
if (!getExportFileBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, exportFile_);
}
if (disableSolve_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(15, disableSolve_);
}
if (profileLocalSearch_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(16, profileLocalSearch_);
}
if (printLocalSearchProfile_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(17, printLocalSearchProfile_);
}
if (useCompactTable_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(100, useCompactTable_);
}
if (useSmallTable_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(101, useSmallTable_);
}
if (useSatTable_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(102, useSatTable_);
}
if (ac4RTableThreshold_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(103, ac4RTableThreshold_);
}
if (useMddTable_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(104, useMddTable_);
}
if (useCumulativeEdgeFinder_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(105, useCumulativeEdgeFinder_);
}
if (useCumulativeTimeTable_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(106, useCumulativeTimeTable_);
}
if (useSequenceHighDemandTasks_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(107, useSequenceHighDemandTasks_);
}
if (useAllPossibleDisjunctions_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(108, useAllPossibleDisjunctions_);
}
if (maxEdgeFinderSize_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(109, maxEdgeFinderSize_);
}
if (diffnUseCumulative_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(110, diffnUseCumulative_);
}
if (useElementRmq_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(111, useElementRmq_);
}
if (useCumulativeTimeTableSync_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(112, useCumulativeTimeTableSync_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ortools.constraintsolver.ConstraintSolverParameters)) {
return super.equals(obj);
}
com.google.ortools.constraintsolver.ConstraintSolverParameters other = (com.google.ortools.constraintsolver.ConstraintSolverParameters) obj;
boolean result = true;
result = result && compressTrail_ == other.compressTrail_;
result = result && (getTrailBlockSize()
== other.getTrailBlockSize());
result = result && (getArraySplitSize()
== other.getArraySplitSize());
result = result && (getStoreNames()
== other.getStoreNames());
result = result && (getNameCastVariables()
== other.getNameCastVariables());
result = result && (getNameAllVariables()
== other.getNameAllVariables());
result = result && (getProfilePropagation()
== other.getProfilePropagation());
result = result && getProfileFile()
.equals(other.getProfileFile());
result = result && (getProfileLocalSearch()
== other.getProfileLocalSearch());
result = result && (getPrintLocalSearchProfile()
== other.getPrintLocalSearchProfile());
result = result && (getTracePropagation()
== other.getTracePropagation());
result = result && (getTraceSearch()
== other.getTraceSearch());
result = result && (getPrintModel()
== other.getPrintModel());
result = result && (getPrintModelStats()
== other.getPrintModelStats());
result = result && (getPrintAddedConstraints()
== other.getPrintAddedConstraints());
result = result && getExportFile()
.equals(other.getExportFile());
result = result && (getDisableSolve()
== other.getDisableSolve());
result = result && (getUseCompactTable()
== other.getUseCompactTable());
result = result && (getUseSmallTable()
== other.getUseSmallTable());
result = result && (getUseSatTable()
== other.getUseSatTable());
result = result && (getAc4RTableThreshold()
== other.getAc4RTableThreshold());
result = result && (getUseMddTable()
== other.getUseMddTable());
result = result && (getUseCumulativeEdgeFinder()
== other.getUseCumulativeEdgeFinder());
result = result && (getUseCumulativeTimeTable()
== other.getUseCumulativeTimeTable());
result = result && (getUseCumulativeTimeTableSync()
== other.getUseCumulativeTimeTableSync());
result = result && (getUseSequenceHighDemandTasks()
== other.getUseSequenceHighDemandTasks());
result = result && (getUseAllPossibleDisjunctions()
== other.getUseAllPossibleDisjunctions());
result = result && (getMaxEdgeFinderSize()
== other.getMaxEdgeFinderSize());
result = result && (getDiffnUseCumulative()
== other.getDiffnUseCumulative());
result = result && (getUseElementRmq()
== other.getUseElementRmq());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COMPRESS_TRAIL_FIELD_NUMBER;
hash = (53 * hash) + compressTrail_;
hash = (37 * hash) + TRAIL_BLOCK_SIZE_FIELD_NUMBER;
hash = (53 * hash) + getTrailBlockSize();
hash = (37 * hash) + ARRAY_SPLIT_SIZE_FIELD_NUMBER;
hash = (53 * hash) + getArraySplitSize();
hash = (37 * hash) + STORE_NAMES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getStoreNames());
hash = (37 * hash) + NAME_CAST_VARIABLES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getNameCastVariables());
hash = (37 * hash) + NAME_ALL_VARIABLES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getNameAllVariables());
hash = (37 * hash) + PROFILE_PROPAGATION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getProfilePropagation());
hash = (37 * hash) + PROFILE_FILE_FIELD_NUMBER;
hash = (53 * hash) + getProfileFile().hashCode();
hash = (37 * hash) + PROFILE_LOCAL_SEARCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getProfileLocalSearch());
hash = (37 * hash) + PRINT_LOCAL_SEARCH_PROFILE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getPrintLocalSearchProfile());
hash = (37 * hash) + TRACE_PROPAGATION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTracePropagation());
hash = (37 * hash) + TRACE_SEARCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTraceSearch());
hash = (37 * hash) + PRINT_MODEL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getPrintModel());
hash = (37 * hash) + PRINT_MODEL_STATS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getPrintModelStats());
hash = (37 * hash) + PRINT_ADDED_CONSTRAINTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getPrintAddedConstraints());
hash = (37 * hash) + EXPORT_FILE_FIELD_NUMBER;
hash = (53 * hash) + getExportFile().hashCode();
hash = (37 * hash) + DISABLE_SOLVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDisableSolve());
hash = (37 * hash) + USE_COMPACT_TABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseCompactTable());
hash = (37 * hash) + USE_SMALL_TABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseSmallTable());
hash = (37 * hash) + USE_SAT_TABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseSatTable());
hash = (37 * hash) + AC4R_TABLE_THRESHOLD_FIELD_NUMBER;
hash = (53 * hash) + getAc4RTableThreshold();
hash = (37 * hash) + USE_MDD_TABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseMddTable());
hash = (37 * hash) + USE_CUMULATIVE_EDGE_FINDER_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseCumulativeEdgeFinder());
hash = (37 * hash) + USE_CUMULATIVE_TIME_TABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseCumulativeTimeTable());
hash = (37 * hash) + USE_CUMULATIVE_TIME_TABLE_SYNC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseCumulativeTimeTableSync());
hash = (37 * hash) + USE_SEQUENCE_HIGH_DEMAND_TASKS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseSequenceHighDemandTasks());
hash = (37 * hash) + USE_ALL_POSSIBLE_DISJUNCTIONS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseAllPossibleDisjunctions());
hash = (37 * hash) + MAX_EDGE_FINDER_SIZE_FIELD_NUMBER;
hash = (53 * hash) + getMaxEdgeFinderSize();
hash = (37 * hash) + DIFFN_USE_CUMULATIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDiffnUseCumulative());
hash = (37 * hash) + USE_ELEMENT_RMQ_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseElementRmq());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ortools.constraintsolver.ConstraintSolverParameters prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Solver parameters.
*
*
* Protobuf type {@code operations_research.ConstraintSolverParameters}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:operations_research.ConstraintSolverParameters)
com.google.ortools.constraintsolver.ConstraintSolverParametersOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.constraintsolver.SolverParameters.internal_static_operations_research_ConstraintSolverParameters_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.constraintsolver.SolverParameters.internal_static_operations_research_ConstraintSolverParameters_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.constraintsolver.ConstraintSolverParameters.class, com.google.ortools.constraintsolver.ConstraintSolverParameters.Builder.class);
}
// Construct using com.google.ortools.constraintsolver.ConstraintSolverParameters.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
compressTrail_ = 0;
trailBlockSize_ = 0;
arraySplitSize_ = 0;
storeNames_ = false;
nameCastVariables_ = false;
nameAllVariables_ = false;
profilePropagation_ = false;
profileFile_ = "";
profileLocalSearch_ = false;
printLocalSearchProfile_ = false;
tracePropagation_ = false;
traceSearch_ = false;
printModel_ = false;
printModelStats_ = false;
printAddedConstraints_ = false;
exportFile_ = "";
disableSolve_ = false;
useCompactTable_ = false;
useSmallTable_ = false;
useSatTable_ = false;
ac4RTableThreshold_ = 0;
useMddTable_ = false;
useCumulativeEdgeFinder_ = false;
useCumulativeTimeTable_ = false;
useCumulativeTimeTableSync_ = false;
useSequenceHighDemandTasks_ = false;
useAllPossibleDisjunctions_ = false;
maxEdgeFinderSize_ = 0;
diffnUseCumulative_ = false;
useElementRmq_ = false;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ortools.constraintsolver.SolverParameters.internal_static_operations_research_ConstraintSolverParameters_descriptor;
}
public com.google.ortools.constraintsolver.ConstraintSolverParameters getDefaultInstanceForType() {
return com.google.ortools.constraintsolver.ConstraintSolverParameters.getDefaultInstance();
}
public com.google.ortools.constraintsolver.ConstraintSolverParameters build() {
com.google.ortools.constraintsolver.ConstraintSolverParameters result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.ortools.constraintsolver.ConstraintSolverParameters buildPartial() {
com.google.ortools.constraintsolver.ConstraintSolverParameters result = new com.google.ortools.constraintsolver.ConstraintSolverParameters(this);
result.compressTrail_ = compressTrail_;
result.trailBlockSize_ = trailBlockSize_;
result.arraySplitSize_ = arraySplitSize_;
result.storeNames_ = storeNames_;
result.nameCastVariables_ = nameCastVariables_;
result.nameAllVariables_ = nameAllVariables_;
result.profilePropagation_ = profilePropagation_;
result.profileFile_ = profileFile_;
result.profileLocalSearch_ = profileLocalSearch_;
result.printLocalSearchProfile_ = printLocalSearchProfile_;
result.tracePropagation_ = tracePropagation_;
result.traceSearch_ = traceSearch_;
result.printModel_ = printModel_;
result.printModelStats_ = printModelStats_;
result.printAddedConstraints_ = printAddedConstraints_;
result.exportFile_ = exportFile_;
result.disableSolve_ = disableSolve_;
result.useCompactTable_ = useCompactTable_;
result.useSmallTable_ = useSmallTable_;
result.useSatTable_ = useSatTable_;
result.ac4RTableThreshold_ = ac4RTableThreshold_;
result.useMddTable_ = useMddTable_;
result.useCumulativeEdgeFinder_ = useCumulativeEdgeFinder_;
result.useCumulativeTimeTable_ = useCumulativeTimeTable_;
result.useCumulativeTimeTableSync_ = useCumulativeTimeTableSync_;
result.useSequenceHighDemandTasks_ = useSequenceHighDemandTasks_;
result.useAllPossibleDisjunctions_ = useAllPossibleDisjunctions_;
result.maxEdgeFinderSize_ = maxEdgeFinderSize_;
result.diffnUseCumulative_ = diffnUseCumulative_;
result.useElementRmq_ = useElementRmq_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ortools.constraintsolver.ConstraintSolverParameters) {
return mergeFrom((com.google.ortools.constraintsolver.ConstraintSolverParameters)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ortools.constraintsolver.ConstraintSolverParameters other) {
if (other == com.google.ortools.constraintsolver.ConstraintSolverParameters.getDefaultInstance()) return this;
if (other.compressTrail_ != 0) {
setCompressTrailValue(other.getCompressTrailValue());
}
if (other.getTrailBlockSize() != 0) {
setTrailBlockSize(other.getTrailBlockSize());
}
if (other.getArraySplitSize() != 0) {
setArraySplitSize(other.getArraySplitSize());
}
if (other.getStoreNames() != false) {
setStoreNames(other.getStoreNames());
}
if (other.getNameCastVariables() != false) {
setNameCastVariables(other.getNameCastVariables());
}
if (other.getNameAllVariables() != false) {
setNameAllVariables(other.getNameAllVariables());
}
if (other.getProfilePropagation() != false) {
setProfilePropagation(other.getProfilePropagation());
}
if (!other.getProfileFile().isEmpty()) {
profileFile_ = other.profileFile_;
onChanged();
}
if (other.getProfileLocalSearch() != false) {
setProfileLocalSearch(other.getProfileLocalSearch());
}
if (other.getPrintLocalSearchProfile() != false) {
setPrintLocalSearchProfile(other.getPrintLocalSearchProfile());
}
if (other.getTracePropagation() != false) {
setTracePropagation(other.getTracePropagation());
}
if (other.getTraceSearch() != false) {
setTraceSearch(other.getTraceSearch());
}
if (other.getPrintModel() != false) {
setPrintModel(other.getPrintModel());
}
if (other.getPrintModelStats() != false) {
setPrintModelStats(other.getPrintModelStats());
}
if (other.getPrintAddedConstraints() != false) {
setPrintAddedConstraints(other.getPrintAddedConstraints());
}
if (!other.getExportFile().isEmpty()) {
exportFile_ = other.exportFile_;
onChanged();
}
if (other.getDisableSolve() != false) {
setDisableSolve(other.getDisableSolve());
}
if (other.getUseCompactTable() != false) {
setUseCompactTable(other.getUseCompactTable());
}
if (other.getUseSmallTable() != false) {
setUseSmallTable(other.getUseSmallTable());
}
if (other.getUseSatTable() != false) {
setUseSatTable(other.getUseSatTable());
}
if (other.getAc4RTableThreshold() != 0) {
setAc4RTableThreshold(other.getAc4RTableThreshold());
}
if (other.getUseMddTable() != false) {
setUseMddTable(other.getUseMddTable());
}
if (other.getUseCumulativeEdgeFinder() != false) {
setUseCumulativeEdgeFinder(other.getUseCumulativeEdgeFinder());
}
if (other.getUseCumulativeTimeTable() != false) {
setUseCumulativeTimeTable(other.getUseCumulativeTimeTable());
}
if (other.getUseCumulativeTimeTableSync() != false) {
setUseCumulativeTimeTableSync(other.getUseCumulativeTimeTableSync());
}
if (other.getUseSequenceHighDemandTasks() != false) {
setUseSequenceHighDemandTasks(other.getUseSequenceHighDemandTasks());
}
if (other.getUseAllPossibleDisjunctions() != false) {
setUseAllPossibleDisjunctions(other.getUseAllPossibleDisjunctions());
}
if (other.getMaxEdgeFinderSize() != 0) {
setMaxEdgeFinderSize(other.getMaxEdgeFinderSize());
}
if (other.getDiffnUseCumulative() != false) {
setDiffnUseCumulative(other.getDiffnUseCumulative());
}
if (other.getUseElementRmq() != false) {
setUseElementRmq(other.getUseElementRmq());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.ortools.constraintsolver.ConstraintSolverParameters parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.ortools.constraintsolver.ConstraintSolverParameters) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int compressTrail_ = 0;
/**
*
* This parameter indicates if the solver should compress the trail
* during the search. No compression means that the solver will be faster,
* but will use more memory.
*
*
* .operations_research.ConstraintSolverParameters.TrailCompression compress_trail = 1;
*/
public int getCompressTrailValue() {
return compressTrail_;
}
/**
*
* This parameter indicates if the solver should compress the trail
* during the search. No compression means that the solver will be faster,
* but will use more memory.
*
*
* .operations_research.ConstraintSolverParameters.TrailCompression compress_trail = 1;
*/
public Builder setCompressTrailValue(int value) {
compressTrail_ = value;
onChanged();
return this;
}
/**
*
* This parameter indicates if the solver should compress the trail
* during the search. No compression means that the solver will be faster,
* but will use more memory.
*
*
* .operations_research.ConstraintSolverParameters.TrailCompression compress_trail = 1;
*/
public com.google.ortools.constraintsolver.ConstraintSolverParameters.TrailCompression getCompressTrail() {
com.google.ortools.constraintsolver.ConstraintSolverParameters.TrailCompression result = com.google.ortools.constraintsolver.ConstraintSolverParameters.TrailCompression.valueOf(compressTrail_);
return result == null ? com.google.ortools.constraintsolver.ConstraintSolverParameters.TrailCompression.UNRECOGNIZED : result;
}
/**
*
* This parameter indicates if the solver should compress the trail
* during the search. No compression means that the solver will be faster,
* but will use more memory.
*
*
* .operations_research.ConstraintSolverParameters.TrailCompression compress_trail = 1;
*/
public Builder setCompressTrail(com.google.ortools.constraintsolver.ConstraintSolverParameters.TrailCompression value) {
if (value == null) {
throw new NullPointerException();
}
compressTrail_ = value.getNumber();
onChanged();
return this;
}
/**
*
* This parameter indicates if the solver should compress the trail
* during the search. No compression means that the solver will be faster,
* but will use more memory.
*
*
* .operations_research.ConstraintSolverParameters.TrailCompression compress_trail = 1;
*/
public Builder clearCompressTrail() {
compressTrail_ = 0;
onChanged();
return this;
}
private int trailBlockSize_ ;
/**
*
* This parameter indicates the default size of a block of the trail.
* Compression applies at the block level.
*
*
* int32 trail_block_size = 2;
*/
public int getTrailBlockSize() {
return trailBlockSize_;
}
/**
*
* This parameter indicates the default size of a block of the trail.
* Compression applies at the block level.
*
*
* int32 trail_block_size = 2;
*/
public Builder setTrailBlockSize(int value) {
trailBlockSize_ = value;
onChanged();
return this;
}
/**
*
* This parameter indicates the default size of a block of the trail.
* Compression applies at the block level.
*
*
* int32 trail_block_size = 2;
*/
public Builder clearTrailBlockSize() {
trailBlockSize_ = 0;
onChanged();
return this;
}
private int arraySplitSize_ ;
/**
*
* When a sum/min/max operation is applied on a large array, this
* array is recursively split into blocks of size 'array_split_size'.
*
*
* int32 array_split_size = 3;
*/
public int getArraySplitSize() {
return arraySplitSize_;
}
/**
*
* When a sum/min/max operation is applied on a large array, this
* array is recursively split into blocks of size 'array_split_size'.
*
*
* int32 array_split_size = 3;
*/
public Builder setArraySplitSize(int value) {
arraySplitSize_ = value;
onChanged();
return this;
}
/**
*
* When a sum/min/max operation is applied on a large array, this
* array is recursively split into blocks of size 'array_split_size'.
*
*
* int32 array_split_size = 3;
*/
public Builder clearArraySplitSize() {
arraySplitSize_ = 0;
onChanged();
return this;
}
private boolean storeNames_ ;
/**
*
* This parameters indicates if the solver should store the names of
* the objets it manages.
*
*
* bool store_names = 4;
*/
public boolean getStoreNames() {
return storeNames_;
}
/**
*
* This parameters indicates if the solver should store the names of
* the objets it manages.
*
*
* bool store_names = 4;
*/
public Builder setStoreNames(boolean value) {
storeNames_ = value;
onChanged();
return this;
}
/**
*
* This parameters indicates if the solver should store the names of
* the objets it manages.
*
*
* bool store_names = 4;
*/
public Builder clearStoreNames() {
storeNames_ = false;
onChanged();
return this;
}
private boolean nameCastVariables_ ;
/**
*
* Create names for cast variables.
*
*
* bool name_cast_variables = 5;
*/
public boolean getNameCastVariables() {
return nameCastVariables_;
}
/**
*
* Create names for cast variables.
*
*
* bool name_cast_variables = 5;
*/
public Builder setNameCastVariables(boolean value) {
nameCastVariables_ = value;
onChanged();
return this;
}
/**
*
* Create names for cast variables.
*
*
* bool name_cast_variables = 5;
*/
public Builder clearNameCastVariables() {
nameCastVariables_ = false;
onChanged();
return this;
}
private boolean nameAllVariables_ ;
/**
*
* Should anonymous variables be given a name.
*
*
* bool name_all_variables = 6;
*/
public boolean getNameAllVariables() {
return nameAllVariables_;
}
/**
*
* Should anonymous variables be given a name.
*
*
* bool name_all_variables = 6;
*/
public Builder setNameAllVariables(boolean value) {
nameAllVariables_ = value;
onChanged();
return this;
}
/**
*
* Should anonymous variables be given a name.
*
*
* bool name_all_variables = 6;
*/
public Builder clearNameAllVariables() {
nameAllVariables_ = false;
onChanged();
return this;
}
private boolean profilePropagation_ ;
/**
*
* Activate propagation profiling.
*
*
* bool profile_propagation = 7;
*/
public boolean getProfilePropagation() {
return profilePropagation_;
}
/**
*
* Activate propagation profiling.
*
*
* bool profile_propagation = 7;
*/
public Builder setProfilePropagation(boolean value) {
profilePropagation_ = value;
onChanged();
return this;
}
/**
*
* Activate propagation profiling.
*
*
* bool profile_propagation = 7;
*/
public Builder clearProfilePropagation() {
profilePropagation_ = false;
onChanged();
return this;
}
private java.lang.Object profileFile_ = "";
/**
*
* Export propagation profiling data to file.
*
*
* string profile_file = 8;
*/
public java.lang.String getProfileFile() {
java.lang.Object ref = profileFile_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
profileFile_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Export propagation profiling data to file.
*
*
* string profile_file = 8;
*/
public com.google.protobuf.ByteString
getProfileFileBytes() {
java.lang.Object ref = profileFile_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
profileFile_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Export propagation profiling data to file.
*
*
* string profile_file = 8;
*/
public Builder setProfileFile(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
profileFile_ = value;
onChanged();
return this;
}
/**
*
* Export propagation profiling data to file.
*
*
* string profile_file = 8;
*/
public Builder clearProfileFile() {
profileFile_ = getDefaultInstance().getProfileFile();
onChanged();
return this;
}
/**
*
* Export propagation profiling data to file.
*
*
* string profile_file = 8;
*/
public Builder setProfileFileBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
profileFile_ = value;
onChanged();
return this;
}
private boolean profileLocalSearch_ ;
/**
*
* Activate local search profiling.
*
*
* bool profile_local_search = 16;
*/
public boolean getProfileLocalSearch() {
return profileLocalSearch_;
}
/**
*
* Activate local search profiling.
*
*
* bool profile_local_search = 16;
*/
public Builder setProfileLocalSearch(boolean value) {
profileLocalSearch_ = value;
onChanged();
return this;
}
/**
*
* Activate local search profiling.
*
*
* bool profile_local_search = 16;
*/
public Builder clearProfileLocalSearch() {
profileLocalSearch_ = false;
onChanged();
return this;
}
private boolean printLocalSearchProfile_ ;
/**
*
* Print local search profiling data after solving.
*
*
* bool print_local_search_profile = 17;
*/
public boolean getPrintLocalSearchProfile() {
return printLocalSearchProfile_;
}
/**
*
* Print local search profiling data after solving.
*
*
* bool print_local_search_profile = 17;
*/
public Builder setPrintLocalSearchProfile(boolean value) {
printLocalSearchProfile_ = value;
onChanged();
return this;
}
/**
*
* Print local search profiling data after solving.
*
*
* bool print_local_search_profile = 17;
*/
public Builder clearPrintLocalSearchProfile() {
printLocalSearchProfile_ = false;
onChanged();
return this;
}
private boolean tracePropagation_ ;
/**
*
* Activate propagate tracing.
*
*
* bool trace_propagation = 9;
*/
public boolean getTracePropagation() {
return tracePropagation_;
}
/**
*
* Activate propagate tracing.
*
*
* bool trace_propagation = 9;
*/
public Builder setTracePropagation(boolean value) {
tracePropagation_ = value;
onChanged();
return this;
}
/**
*
* Activate propagate tracing.
*
*
* bool trace_propagation = 9;
*/
public Builder clearTracePropagation() {
tracePropagation_ = false;
onChanged();
return this;
}
private boolean traceSearch_ ;
/**
*
* Trace search.
*
*
* bool trace_search = 10;
*/
public boolean getTraceSearch() {
return traceSearch_;
}
/**
*
* Trace search.
*
*
* bool trace_search = 10;
*/
public Builder setTraceSearch(boolean value) {
traceSearch_ = value;
onChanged();
return this;
}
/**
*
* Trace search.
*
*
* bool trace_search = 10;
*/
public Builder clearTraceSearch() {
traceSearch_ = false;
onChanged();
return this;
}
private boolean printModel_ ;
/**
*
* Print the model before solving.
*
*
* bool print_model = 11;
*/
public boolean getPrintModel() {
return printModel_;
}
/**
*
* Print the model before solving.
*
*
* bool print_model = 11;
*/
public Builder setPrintModel(boolean value) {
printModel_ = value;
onChanged();
return this;
}
/**
*
* Print the model before solving.
*
*
* bool print_model = 11;
*/
public Builder clearPrintModel() {
printModel_ = false;
onChanged();
return this;
}
private boolean printModelStats_ ;
/**
*
* Print model statistics before solving.
*
*
* bool print_model_stats = 12;
*/
public boolean getPrintModelStats() {
return printModelStats_;
}
/**
*
* Print model statistics before solving.
*
*
* bool print_model_stats = 12;
*/
public Builder setPrintModelStats(boolean value) {
printModelStats_ = value;
onChanged();
return this;
}
/**
*
* Print model statistics before solving.
*
*
* bool print_model_stats = 12;
*/
public Builder clearPrintModelStats() {
printModelStats_ = false;
onChanged();
return this;
}
private boolean printAddedConstraints_ ;
/**
*
* Print added constraints.
*
*
* bool print_added_constraints = 13;
*/
public boolean getPrintAddedConstraints() {
return printAddedConstraints_;
}
/**
*
* Print added constraints.
*
*
* bool print_added_constraints = 13;
*/
public Builder setPrintAddedConstraints(boolean value) {
printAddedConstraints_ = value;
onChanged();
return this;
}
/**
*
* Print added constraints.
*
*
* bool print_added_constraints = 13;
*/
public Builder clearPrintAddedConstraints() {
printAddedConstraints_ = false;
onChanged();
return this;
}
private java.lang.Object exportFile_ = "";
/**
*
* Export model to file.
*
*
* string export_file = 14;
*/
public java.lang.String getExportFile() {
java.lang.Object ref = exportFile_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
exportFile_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Export model to file.
*
*
* string export_file = 14;
*/
public com.google.protobuf.ByteString
getExportFileBytes() {
java.lang.Object ref = exportFile_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
exportFile_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Export model to file.
*
*
* string export_file = 14;
*/
public Builder setExportFile(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
exportFile_ = value;
onChanged();
return this;
}
/**
*
* Export model to file.
*
*
* string export_file = 14;
*/
public Builder clearExportFile() {
exportFile_ = getDefaultInstance().getExportFile();
onChanged();
return this;
}
/**
*
* Export model to file.
*
*
* string export_file = 14;
*/
public Builder setExportFileBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
exportFile_ = value;
onChanged();
return this;
}
private boolean disableSolve_ ;
/**
* bool disable_solve = 15;
*/
public boolean getDisableSolve() {
return disableSolve_;
}
/**
* bool disable_solve = 15;
*/
public Builder setDisableSolve(boolean value) {
disableSolve_ = value;
onChanged();
return this;
}
/**
* bool disable_solve = 15;
*/
public Builder clearDisableSolve() {
disableSolve_ = false;
onChanged();
return this;
}
private boolean useCompactTable_ ;
/**
*
* Control the implementation of the table constraint.
*
*
* bool use_compact_table = 100;
*/
public boolean getUseCompactTable() {
return useCompactTable_;
}
/**
*
* Control the implementation of the table constraint.
*
*
* bool use_compact_table = 100;
*/
public Builder setUseCompactTable(boolean value) {
useCompactTable_ = value;
onChanged();
return this;
}
/**
*
* Control the implementation of the table constraint.
*
*
* bool use_compact_table = 100;
*/
public Builder clearUseCompactTable() {
useCompactTable_ = false;
onChanged();
return this;
}
private boolean useSmallTable_ ;
/**
* bool use_small_table = 101;
*/
public boolean getUseSmallTable() {
return useSmallTable_;
}
/**
* bool use_small_table = 101;
*/
public Builder setUseSmallTable(boolean value) {
useSmallTable_ = value;
onChanged();
return this;
}
/**
* bool use_small_table = 101;
*/
public Builder clearUseSmallTable() {
useSmallTable_ = false;
onChanged();
return this;
}
private boolean useSatTable_ ;
/**
* bool use_sat_table = 102;
*/
public boolean getUseSatTable() {
return useSatTable_;
}
/**
* bool use_sat_table = 102;
*/
public Builder setUseSatTable(boolean value) {
useSatTable_ = value;
onChanged();
return this;
}
/**
* bool use_sat_table = 102;
*/
public Builder clearUseSatTable() {
useSatTable_ = false;
onChanged();
return this;
}
private int ac4RTableThreshold_ ;
/**
* int32 ac4r_table_threshold = 103;
*/
public int getAc4RTableThreshold() {
return ac4RTableThreshold_;
}
/**
* int32 ac4r_table_threshold = 103;
*/
public Builder setAc4RTableThreshold(int value) {
ac4RTableThreshold_ = value;
onChanged();
return this;
}
/**
* int32 ac4r_table_threshold = 103;
*/
public Builder clearAc4RTableThreshold() {
ac4RTableThreshold_ = 0;
onChanged();
return this;
}
private boolean useMddTable_ ;
/**
* bool use_mdd_table = 104;
*/
public boolean getUseMddTable() {
return useMddTable_;
}
/**
* bool use_mdd_table = 104;
*/
public Builder setUseMddTable(boolean value) {
useMddTable_ = value;
onChanged();
return this;
}
/**
* bool use_mdd_table = 104;
*/
public Builder clearUseMddTable() {
useMddTable_ = false;
onChanged();
return this;
}
private boolean useCumulativeEdgeFinder_ ;
/**
*
* Control the propagation of the cumulative constraint.
*
*
* bool use_cumulative_edge_finder = 105;
*/
public boolean getUseCumulativeEdgeFinder() {
return useCumulativeEdgeFinder_;
}
/**
*
* Control the propagation of the cumulative constraint.
*
*
* bool use_cumulative_edge_finder = 105;
*/
public Builder setUseCumulativeEdgeFinder(boolean value) {
useCumulativeEdgeFinder_ = value;
onChanged();
return this;
}
/**
*
* Control the propagation of the cumulative constraint.
*
*
* bool use_cumulative_edge_finder = 105;
*/
public Builder clearUseCumulativeEdgeFinder() {
useCumulativeEdgeFinder_ = false;
onChanged();
return this;
}
private boolean useCumulativeTimeTable_ ;
/**
* bool use_cumulative_time_table = 106;
*/
public boolean getUseCumulativeTimeTable() {
return useCumulativeTimeTable_;
}
/**
* bool use_cumulative_time_table = 106;
*/
public Builder setUseCumulativeTimeTable(boolean value) {
useCumulativeTimeTable_ = value;
onChanged();
return this;
}
/**
* bool use_cumulative_time_table = 106;
*/
public Builder clearUseCumulativeTimeTable() {
useCumulativeTimeTable_ = false;
onChanged();
return this;
}
private boolean useCumulativeTimeTableSync_ ;
/**
* bool use_cumulative_time_table_sync = 112;
*/
public boolean getUseCumulativeTimeTableSync() {
return useCumulativeTimeTableSync_;
}
/**
* bool use_cumulative_time_table_sync = 112;
*/
public Builder setUseCumulativeTimeTableSync(boolean value) {
useCumulativeTimeTableSync_ = value;
onChanged();
return this;
}
/**
* bool use_cumulative_time_table_sync = 112;
*/
public Builder clearUseCumulativeTimeTableSync() {
useCumulativeTimeTableSync_ = false;
onChanged();
return this;
}
private boolean useSequenceHighDemandTasks_ ;
/**
* bool use_sequence_high_demand_tasks = 107;
*/
public boolean getUseSequenceHighDemandTasks() {
return useSequenceHighDemandTasks_;
}
/**
* bool use_sequence_high_demand_tasks = 107;
*/
public Builder setUseSequenceHighDemandTasks(boolean value) {
useSequenceHighDemandTasks_ = value;
onChanged();
return this;
}
/**
* bool use_sequence_high_demand_tasks = 107;
*/
public Builder clearUseSequenceHighDemandTasks() {
useSequenceHighDemandTasks_ = false;
onChanged();
return this;
}
private boolean useAllPossibleDisjunctions_ ;
/**
* bool use_all_possible_disjunctions = 108;
*/
public boolean getUseAllPossibleDisjunctions() {
return useAllPossibleDisjunctions_;
}
/**
* bool use_all_possible_disjunctions = 108;
*/
public Builder setUseAllPossibleDisjunctions(boolean value) {
useAllPossibleDisjunctions_ = value;
onChanged();
return this;
}
/**
* bool use_all_possible_disjunctions = 108;
*/
public Builder clearUseAllPossibleDisjunctions() {
useAllPossibleDisjunctions_ = false;
onChanged();
return this;
}
private int maxEdgeFinderSize_ ;
/**
* int32 max_edge_finder_size = 109;
*/
public int getMaxEdgeFinderSize() {
return maxEdgeFinderSize_;
}
/**
* int32 max_edge_finder_size = 109;
*/
public Builder setMaxEdgeFinderSize(int value) {
maxEdgeFinderSize_ = value;
onChanged();
return this;
}
/**
* int32 max_edge_finder_size = 109;
*/
public Builder clearMaxEdgeFinderSize() {
maxEdgeFinderSize_ = 0;
onChanged();
return this;
}
private boolean diffnUseCumulative_ ;
/**
*
* Control the propagation of the diffn constraint.
*
*
* bool diffn_use_cumulative = 110;
*/
public boolean getDiffnUseCumulative() {
return diffnUseCumulative_;
}
/**
*
* Control the propagation of the diffn constraint.
*
*
* bool diffn_use_cumulative = 110;
*/
public Builder setDiffnUseCumulative(boolean value) {
diffnUseCumulative_ = value;
onChanged();
return this;
}
/**
*
* Control the propagation of the diffn constraint.
*
*
* bool diffn_use_cumulative = 110;
*/
public Builder clearDiffnUseCumulative() {
diffnUseCumulative_ = false;
onChanged();
return this;
}
private boolean useElementRmq_ ;
/**
*
* Control the implementation of the element constraint.
*
*
* bool use_element_rmq = 111;
*/
public boolean getUseElementRmq() {
return useElementRmq_;
}
/**
*
* Control the implementation of the element constraint.
*
*
* bool use_element_rmq = 111;
*/
public Builder setUseElementRmq(boolean value) {
useElementRmq_ = value;
onChanged();
return this;
}
/**
*
* Control the implementation of the element constraint.
*
*
* bool use_element_rmq = 111;
*/
public Builder clearUseElementRmq() {
useElementRmq_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:operations_research.ConstraintSolverParameters)
}
// @@protoc_insertion_point(class_scope:operations_research.ConstraintSolverParameters)
private static final com.google.ortools.constraintsolver.ConstraintSolverParameters DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ortools.constraintsolver.ConstraintSolverParameters();
}
public static com.google.ortools.constraintsolver.ConstraintSolverParameters getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ConstraintSolverParameters parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConstraintSolverParameters(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.google.ortools.constraintsolver.ConstraintSolverParameters getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy