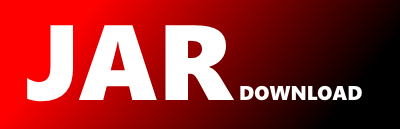
com.google.ortools.constraintsolver.PropagationMonitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-or-tools Show documentation
Show all versions of google-or-tools Show documentation
A project to publish Google OR-Tools as a dependable dependency in Maven.
The newest version!
/* ----------------------------------------------------------------------------
* This file was automatically generated by SWIG (http://www.swig.org).
* Version 3.0.10
*
* Do not make changes to this file unless you know what you are doing--modify
* the SWIG interface file instead.
* ----------------------------------------------------------------------------- */
package com.google.ortools.constraintsolver;
public class PropagationMonitor extends SearchMonitor {
private transient long swigCPtr;
protected PropagationMonitor(long cPtr, boolean cMemoryOwn) {
super(operations_research_constraint_solverJNI.PropagationMonitor_SWIGUpcast(cPtr), cMemoryOwn);
swigCPtr = cPtr;
}
protected static long getCPtr(PropagationMonitor obj) {
return (obj == null) ? 0 : obj.swigCPtr;
}
protected void finalize() {
delete();
}
public synchronized void delete() {
if (swigCPtr != 0) {
if (swigCMemOwn) {
swigCMemOwn = false;
operations_research_constraint_solverJNI.delete_PropagationMonitor(swigCPtr);
}
swigCPtr = 0;
}
super.delete();
}
public void BeginConstraintInitialPropagation(Constraint constraint) {
operations_research_constraint_solverJNI.PropagationMonitor_BeginConstraintInitialPropagation(swigCPtr, this, Constraint.getCPtr(constraint), constraint);
}
public void EndConstraintInitialPropagation(Constraint constraint) {
operations_research_constraint_solverJNI.PropagationMonitor_EndConstraintInitialPropagation(swigCPtr, this, Constraint.getCPtr(constraint), constraint);
}
public void BeginNestedConstraintInitialPropagation(Constraint parent, Constraint nested) {
operations_research_constraint_solverJNI.PropagationMonitor_BeginNestedConstraintInitialPropagation(swigCPtr, this, Constraint.getCPtr(parent), parent, Constraint.getCPtr(nested), nested);
}
public void EndNestedConstraintInitialPropagation(Constraint parent, Constraint nested) {
operations_research_constraint_solverJNI.PropagationMonitor_EndNestedConstraintInitialPropagation(swigCPtr, this, Constraint.getCPtr(parent), parent, Constraint.getCPtr(nested), nested);
}
public void RegisterDemon(Demon demon) {
operations_research_constraint_solverJNI.PropagationMonitor_RegisterDemon(swigCPtr, this, Demon.getCPtr(demon), demon);
}
public void BeginDemonRun(Demon demon) {
operations_research_constraint_solverJNI.PropagationMonitor_BeginDemonRun(swigCPtr, this, Demon.getCPtr(demon), demon);
}
public void EndDemonRun(Demon demon) {
operations_research_constraint_solverJNI.PropagationMonitor_EndDemonRun(swigCPtr, this, Demon.getCPtr(demon), demon);
}
public void StartProcessingIntegerVariable(IntVar var) {
operations_research_constraint_solverJNI.PropagationMonitor_StartProcessingIntegerVariable(swigCPtr, this, IntVar.getCPtr(var), var);
}
public void EndProcessingIntegerVariable(IntVar var) {
operations_research_constraint_solverJNI.PropagationMonitor_EndProcessingIntegerVariable(swigCPtr, this, IntVar.getCPtr(var), var);
}
public void PushContext(String context) {
operations_research_constraint_solverJNI.PropagationMonitor_PushContext(swigCPtr, this, context);
}
public void PopContext() {
operations_research_constraint_solverJNI.PropagationMonitor_PopContext(swigCPtr, this);
}
public void setMin(IntExpr expr, long new_min) {
operations_research_constraint_solverJNI.PropagationMonitor_setMin__SWIG_0(swigCPtr, this, IntExpr.getCPtr(expr), expr, new_min);
}
public void setMax(IntExpr expr, long new_max) {
operations_research_constraint_solverJNI.PropagationMonitor_setMax__SWIG_0(swigCPtr, this, IntExpr.getCPtr(expr), expr, new_max);
}
public void setRange(IntExpr expr, long new_min, long new_max) {
operations_research_constraint_solverJNI.PropagationMonitor_setRange__SWIG_0(swigCPtr, this, IntExpr.getCPtr(expr), expr, new_min, new_max);
}
public void setMin(IntVar var, long new_min) {
operations_research_constraint_solverJNI.PropagationMonitor_setMin__SWIG_1(swigCPtr, this, IntVar.getCPtr(var), var, new_min);
}
public void setMax(IntVar var, long new_max) {
operations_research_constraint_solverJNI.PropagationMonitor_setMax__SWIG_1(swigCPtr, this, IntVar.getCPtr(var), var, new_max);
}
public void setRange(IntVar var, long new_min, long new_max) {
operations_research_constraint_solverJNI.PropagationMonitor_setRange__SWIG_1(swigCPtr, this, IntVar.getCPtr(var), var, new_min, new_max);
}
public void RemoveValue(IntVar var, long value) {
operations_research_constraint_solverJNI.PropagationMonitor_RemoveValue(swigCPtr, this, IntVar.getCPtr(var), var, value);
}
public void setValue(IntVar var, long value) {
operations_research_constraint_solverJNI.PropagationMonitor_setValue__SWIG_0(swigCPtr, this, IntVar.getCPtr(var), var, value);
}
public void RemoveInterval(IntVar var, long imin, long imax) {
operations_research_constraint_solverJNI.PropagationMonitor_RemoveInterval(swigCPtr, this, IntVar.getCPtr(var), var, imin, imax);
}
public void setValue(IntVar var, long[] values) {
operations_research_constraint_solverJNI.PropagationMonitor_setValue__SWIG_1(swigCPtr, this, IntVar.getCPtr(var), var, values);
}
public void RemoveValues(IntVar var, long[] values) {
operations_research_constraint_solverJNI.PropagationMonitor_RemoveValues(swigCPtr, this, IntVar.getCPtr(var), var, values);
}
public void SetStartMin(IntervalVar var, long new_min) {
operations_research_constraint_solverJNI.PropagationMonitor_SetStartMin(swigCPtr, this, IntervalVar.getCPtr(var), var, new_min);
}
public void SetStartMax(IntervalVar var, long new_max) {
operations_research_constraint_solverJNI.PropagationMonitor_SetStartMax(swigCPtr, this, IntervalVar.getCPtr(var), var, new_max);
}
public void SetStartRange(IntervalVar var, long new_min, long new_max) {
operations_research_constraint_solverJNI.PropagationMonitor_SetStartRange(swigCPtr, this, IntervalVar.getCPtr(var), var, new_min, new_max);
}
public void SetEndMin(IntervalVar var, long new_min) {
operations_research_constraint_solverJNI.PropagationMonitor_SetEndMin(swigCPtr, this, IntervalVar.getCPtr(var), var, new_min);
}
public void SetEndMax(IntervalVar var, long new_max) {
operations_research_constraint_solverJNI.PropagationMonitor_SetEndMax(swigCPtr, this, IntervalVar.getCPtr(var), var, new_max);
}
public void SetEndRange(IntervalVar var, long new_min, long new_max) {
operations_research_constraint_solverJNI.PropagationMonitor_SetEndRange(swigCPtr, this, IntervalVar.getCPtr(var), var, new_min, new_max);
}
public void SetDurationMin(IntervalVar var, long new_min) {
operations_research_constraint_solverJNI.PropagationMonitor_SetDurationMin(swigCPtr, this, IntervalVar.getCPtr(var), var, new_min);
}
public void SetDurationMax(IntervalVar var, long new_max) {
operations_research_constraint_solverJNI.PropagationMonitor_SetDurationMax(swigCPtr, this, IntervalVar.getCPtr(var), var, new_max);
}
public void SetDurationRange(IntervalVar var, long new_min, long new_max) {
operations_research_constraint_solverJNI.PropagationMonitor_SetDurationRange(swigCPtr, this, IntervalVar.getCPtr(var), var, new_min, new_max);
}
public void SetPerformed(IntervalVar var, boolean value) {
operations_research_constraint_solverJNI.PropagationMonitor_SetPerformed(swigCPtr, this, IntervalVar.getCPtr(var), var, value);
}
public void RankFirst(SequenceVar var, int index) {
operations_research_constraint_solverJNI.PropagationMonitor_RankFirst(swigCPtr, this, SequenceVar.getCPtr(var), var, index);
}
public void RankNotFirst(SequenceVar var, int index) {
operations_research_constraint_solverJNI.PropagationMonitor_RankNotFirst(swigCPtr, this, SequenceVar.getCPtr(var), var, index);
}
public void RankLast(SequenceVar var, int index) {
operations_research_constraint_solverJNI.PropagationMonitor_RankLast(swigCPtr, this, SequenceVar.getCPtr(var), var, index);
}
public void RankNotLast(SequenceVar var, int index) {
operations_research_constraint_solverJNI.PropagationMonitor_RankNotLast(swigCPtr, this, SequenceVar.getCPtr(var), var, index);
}
public void RankSequence(SequenceVar var, int[] rank_first, int[] rank_last, int[] unperformed) {
operations_research_constraint_solverJNI.PropagationMonitor_RankSequence(swigCPtr, this, SequenceVar.getCPtr(var), var, rank_first, rank_last, unperformed);
}
public void Install() {
operations_research_constraint_solverJNI.PropagationMonitor_Install(swigCPtr, this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy